模拟鼠标自动点击
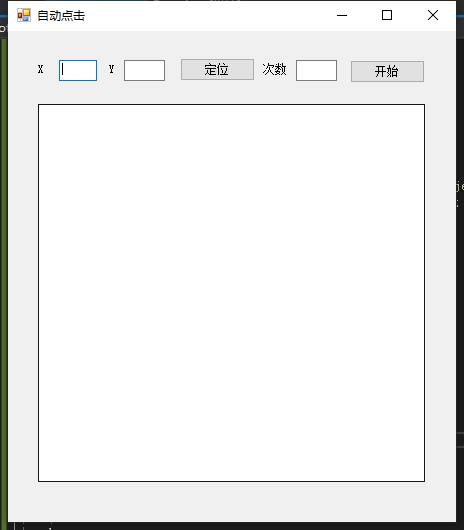
using Newtonsoft.Json; using System; using System.Collections.Generic; using System.Configuration; using System.Threading; using System.Windows.Forms; namespace MouseAoto { public partial class Form1 : Form { #region 鼠标控制 /// <summary> /// 制库 /// </summary> /// <param name="dwFlags">标志位集,指定点击按钮和鼠标动作的多种情况</param> /// <param name="dx">指定鼠标沿x轴的绝对位置或者从上次鼠标事件产生以来移动的数量</param> /// <param name="dy">指定鼠标沿y轴的绝对位置或者从上次鼠标事件产生以来移动的数量</param> /// <param name="dwData">鼠标滚轮移动的数量</param> /// <param name="dwExtraInfo">指定与鼠标事件相关的附加32位值</param> /// <returns></returns> [System.Runtime.InteropServices.DllImport("user32")] private static extern int mouse_event(int dwFlags, int dx, int dy, int dwData, int dwExtraInfo); [System.Runtime.InteropServices.DllImport("user32")] static extern bool SetCursorPos(int X, int Y); //移动鼠标 const int MOUSEEVENTF_MOVE = 0x0001; //模拟鼠标左键按下 const int MOUSEEVENTF_LEFTDOWN = 0x0002; //模拟鼠标左键抬起 const int MOUSEEVENTF_LEFTUP = 0x0004; //模拟鼠标右键按下 const int MOUSEEVENTF_RIGHTDOWN = 0x0008; //模拟鼠标右键抬起 const int MOUSEEVENTF_RIGHTUP = 0x0010; //模拟鼠标中键按下 const int MOUSEEVENTF_MIDDLEDOWN = 0x0020; //模拟鼠标中键抬起 const int MOUSEEVENTF_MIDDLEUP = 0x0040; //标示是否采用绝对坐标 const int MOUSEEVENTF_ABSOLUTE = 0x8000; //模拟鼠标滚轮滚动操作,必须配合dwData参数 const int MOUSEEVENTF_WHEEL = 0x0800; #endregion public Form1() { InitializeComponent(); this.KeyPreview = true; } public void TestMoveMouse(int X, int Y) { SetCursorPos(X, Y); mouse_event(MOUSEEVENTF_LEFTDOWN | MOUSEEVENTF_LEFTUP, 0, 0, 0, 0); } /// <summary> /// 键盘监控 /// </summary> /// <param name="sender"></param> /// <param name="e"></param> private void Form1_PreviewKeyDown(object sender, PreviewKeyDownEventArgs e) { if (e.KeyData == Keys.Escape) { Application.Exit(); } } private void button1_Click(object sender, EventArgs e) { int X = Convert.ToInt32(textBox1.Text); int Y = Convert.ToInt32(textBox2.Text); SetCursorPos(X, Y); } private void button2_Click(object sender, EventArgs e) { List<MouseModel> model = JsonConvert.DeserializeObject<List<MouseModel>>(textBox3.Text); for (int i = 0; i < Convert.ToInt32(textBox4.Text); i++) { foreach (var item in model) { TestMoveMouse(item.X, item.Y); Thread.Sleep(item.date); } } } } public class MouseModel { public int X { get; set; } public int Y { get; set; } public int date { get; set; } } }