vite+vue3+ts+elementPlus前端框架搭建 [二] pinia状态管理
前面已经完成了基本框架搭建,下一步针对各个模块的封装以及实验
本章主要是针对pinia的状态模块实现
1. 创建Store
在src文件夹下创建一个store的文件夹,并在该文件夹下创建index.ts文件,内容如下:import type { App } from 'vue';
import { createPinia } from 'pinia';
const store = createPinia();
// 全局注册 store
export function setupStore(app: App) {
app.use(store);
}
export { store };
有两种写法,第一种写法如下:
在store的文件夹下创建一个新的文件夹:modules,并在该文件夹下创建文件app.ts,内容如下:
import { type Ref, ref } from "vue"
import { defineStore } from "pinia"
export const useAppStore = defineStore('app', {
/**
* 存储全局状态
* 1.必须是箭头函数: 为了在服务器端渲染的时候避免交叉请求导致数据状态污染
* 和 TS 类型推导
*/
state: () => {
return {
count: 0,
list: [1, 2, 3, 4]
}
},
/**
* 用来封装计算属性 有缓存功能 类似于computed
*/
getters: {
getNum(state) {
return state.count + 1
},
getListCount:(state) => state.list.length,
},
/**
* 编辑业务逻辑 类似于methods
*/
actions: {
}
})
第二种写法如下:
modules,并在该文件夹下创建文件setting.ts,内容如下
import { defineStore } from "pinia"
import { useStorage } from "@vueuse/core";
export const useSettingsStore = defineStore("setting", () => {
const device = useStorage("device", "desktop");
const resetDevice = ()=>{
device.value="desktop"
}
return {
device,
resetDevice
}
})
2. 使用useAppStore
在src目录下的index.vue中使用并测试useAppStore、useSettingsStorejs部分
import { ref } from "vue";
import { useRouter } from "vue-router";
import { useAppStore } from "../store/modules/app";
import { useSettingsStore } from "../store/modules/setting";
const router = useRouter();
const appStore = useAppStore();
const settingStore = useSettingsStore();
interface State {
msg: string;
}
const addCount = () => {
appStore.$patch((state) => {
state.count = 10
});
};
const changeDevice = () => {
settingStore.$patch((state) => {
state.device = "desktop-010101"
});
};
const resetDevice = () => {
settingStore.resetDevice()
};
const msg = ref("我是Home.vue");
html部分
<div>
<h1>{{ appStore.count }}</h1>
<h1>{{ appStore.getNum }}</h1>
<h1>{{ appStore.getListCount }}</h1>
</div>
<hr>
<div>
<h1>{{settingStore.device}}</h1>
</div>
<div>
<div><el-button type="primary" @click="addCount">增加Count</el-button></div>
<div><el-button type="primary" @click="changeDevice">改变Device值</el-button></div>
<div><el-button type="primary" @click="resetDevice">重置Device值</el-button></div>
</div>
3. 效果如下:
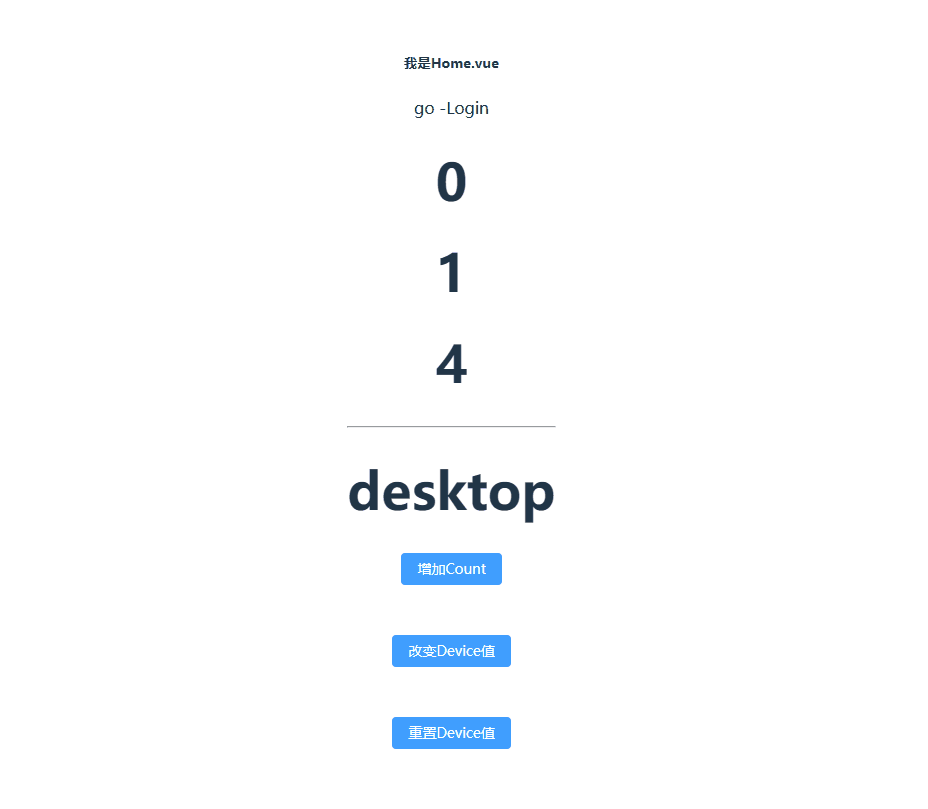