1.排序
1.1元素正排序
list = list.stream()
.sorted(Comparator.comparing(Person::getAge))
.collect(Collectors.toList());
1.2元素逆排序(reversed())
list = list.stream()
.sorted(Comparator.comparing(Person::getAge).reversed())
.collect(Collectors.toList());
2.List排名并获取名次示例
import lombok.Data;
import java.util.*;
import java.util.stream.Collectors;
public class RankTest {
public static void main(String[] args) {
List<Person> list = new ArrayList<Person>() {{
add(new Person(10, "北京"));
add(new Person(10, "西安"));
add(new Person(20, "呼市"));
add(new Person(20, "上海"));
add(new Person(40, "南京"));
add(new Person(60, "青海"));
}};
//从小到大正序
list = list.stream()
.sorted(Comparator.comparing(Person::getAge))
.collect(Collectors.toList());
//从大到小逆序
// list = list.stream()
// .sorted(Comparator.comparing(Person::getAge).reversed())
// .collect(Collectors.toList());
//从大到小
// list.sort((s1, s2) -> -Double.compare(s1.getAge(), s2.getAge()));
int index = 0;
double lastAge = -1;
/**
* 方法1: 出现并列排名后名次不间断
*/
for (Person bean : list) {
if (Double.compare(lastAge, bean.getAge()) != 0) {
lastAge = bean.getAge();
index++;
}
bean.setRank(index);
}
/**
* 方法2.1: 出现并列排名后名次间断,后续名次按实际数据位置累计
*/
// for (int i = 0; i < list.size(); i++) {
// if (list.get(i).getAge() == lastAge ){
// list.get(i).setRank(index);
// if (i + 1 < list.size() && list.get(i + 1).getAge() != lastAge){
// index = i + 1 ;
// }
// }else{
// list.get(i).setRank(++index);
// }
// lastAge = list.get(i).getAge();
// }
/**
* 方法2.2: 出现并列排名后名次间断,后续名次按实际数据位置累计(业务在name唯一的前提下使用)
*/
// Map<String, Integer> rankMap = new HashMap<>();
// for (int i = 1; i <= list.size(); i++) {
// if (i != 1) {
// if (list.get(i - 1).getAge().equals(list.get(i - 2).getAge())) {
// rankMap.put(list.get(i - 1).getName(), rankMap.get(list.get(i - 2).getName()));
// } else {
// rankMap.put(list.get(i - 1).getName(), i);
// }
// } else {
// rankMap.put(list.get(0).getName(), i);
// }
// }
// for (Person person : list) {
// person.setRank(rankMap.get(person.getName()));
// }
//打印结果
list.forEach(p -> {
System.out.println(p);
});
}
}
@Data
class Person {
private Integer age;
private Integer rank;
private String name;
public Person(Integer age, String name) {
this.age = age;
this.name = name;
}
}
2.方法1结果示例
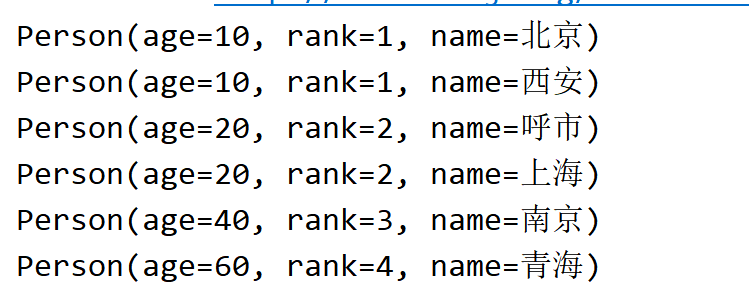
2.方法2.1结果示例
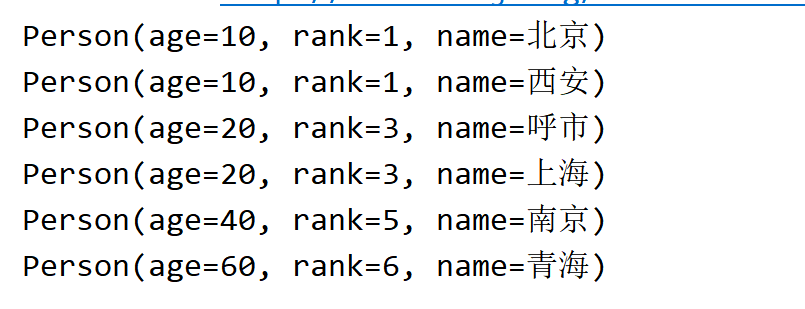
2.方法2.2结果示例
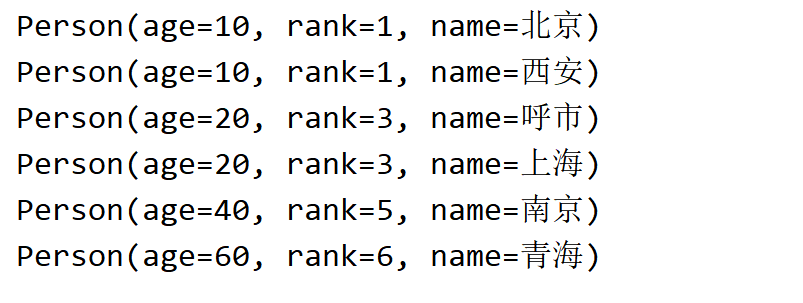