力扣 669. 修剪二叉搜索树
给你二叉搜索树的根节点 root
,同时给定最小边界low
和最大边界 high
。通过修剪二叉搜索树,使得所有节点的值在[low, high]
中。修剪树 不应该 改变保留在树中的元素的相对结构 (即,如果没有被移除,原有的父代子代关系都应当保留)。 可以证明,存在 唯一的答案 。
所以结果应当返回修剪好的二叉搜索树的新的根节点。注意,根节点可能会根据给定的边界发生改变。
示例 1:
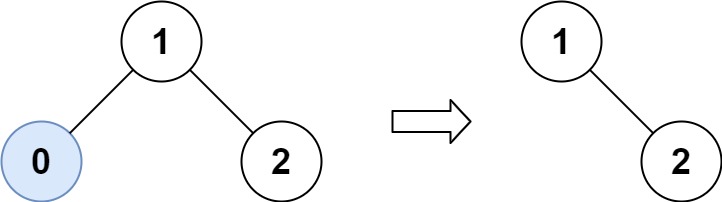
输入:root = [1,0,2], low = 1, high = 2
输出:[1,null,2]
示例 2:
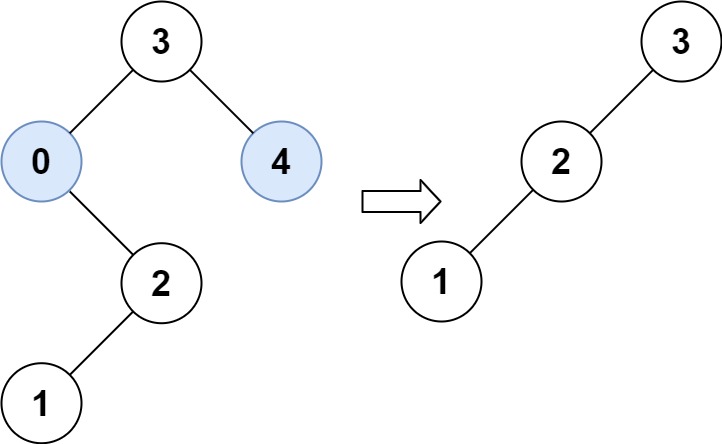
输入:root = [3,0,4,null,2,null,null,1], low = 1, high = 3
输出:[3,2,null,1]
提示:
- 树中节点数在范围
[1, 104]
内 0 <= Node.val <= 104
- 树中每个节点的值都是 唯一 的
- 题目数据保证输入是一棵有效的二叉搜索树
0 <= low <= high <= 104
题解
可以使用递归依次处理每个节点,然后再返回,不过为了节约空间复杂度,选择非递归的方法。
查看代码
/**
* Definition for a binary tree node.
* struct TreeNode {
* int val;
* TreeNode *left;
* TreeNode *right;
* TreeNode() : val(0), left(nullptr), right(nullptr) {}
* TreeNode(int x) : val(x), left(nullptr), right(nullptr) {}
* TreeNode(int x, TreeNode *left, TreeNode *right) : val(x), left(left), right(right) {}
* };
*/
class Solution {
public:
TreeNode* trimBST(TreeNode* root, int low, int high) {
if(root==nullptr)//传入Null
return nullptr;
if(root->val>high&&root->left==nullptr)//没有左子树,val为最小,最小都>high
return nullptr;
if(root->val<low&&root->right==nullptr)//没有右子树,val为最大,最大都<low
return nullptr;
//寻找第一个满足的节点,最后返回这个节点
while(root->val>high||root->val<low){
if(low>root->val)//当前值比较小,往右走
root=root->right;
else
root=root->left;
}
TreeNode* cur=root;//使用cur递归处理子树中不符合条件的节点
while(cur!=nullptr){//递归处理左子树
while(cur->left!=nullptr&&cur->left->val<low)//左节点<low,则删除左子树,用左子树的右节点替代
cur->left=cur->left->right;
cur=cur->left;//递归处理
}
cur=root;//使用cur递归处理子树中不符合条件的节点
while(cur!=nullptr){//递归处理左子树
while(cur->right!=nullptr&&cur->right->val>high)//右节点>high,则删除右子树,用右子树的左节点替代
cur->right=cur->right->left;
cur=cur->right;//递归处理
}
return root;
}
};