jQuery AJAX and JSON Example in ASP.Net Core MVC
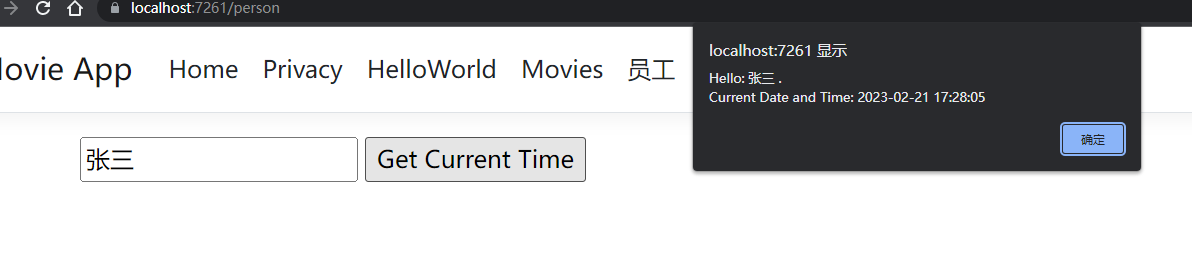
public class PersonModel { public string Name { get; set; } public string DateTime { get; set; } }
public class PersonController : Controller { public IActionResult Index() { return View(); } [HttpPost] public JsonResult AjaxMethod(string name) { PersonModel model = new PersonModel { Name = name, DateTime = DateTime.Now.ToString(), }; return Json(false); return Json(model); } }
<input type="text" id="txtName" /> <input type="button" id="btnGet" value="Get Current Time" />
@section Scripts { <script> $(function () { $("#btnGet").click(function () { $.ajax({ type: "POST", url: "/Person/AjaxMethod", data: { "name": $("#txtName").val() }, success: function (response) { alert("Hello: " + response.name + " .\nCurrent Date and Time: " + response.dateTime); }, failure: function (response) { alert(response.responseText); }, error: function (response) { alert(response.responseText); } }); }); }); </script> }