spirmmvc框架整合手抄版示例,供基础搭建代码对照
注明所有文档和图片完整对照,辟免笔记出错,不能复习

package com.ithm.config; import com.alibaba.druid.pool.DruidDataSource; import org.springframework.beans.factory.annotation.Value; import org.springframework.context.annotation.Bean; import javax.sql.DataSource; public class jdbcConfig { @Value("${jdbc.driver}") private String driver; @Value("${jdbc.url}") private String url; @Value("${jdbc.username}") private String username; @Value("${jdbc.password}") private String password; @Bean public DataSource dataSource(){ DruidDataSource dataSource = new DruidDataSource(); dataSource.setDriverClassName("com.mysql.jdbc.Driver"); dataSource.setUrl("jdbc:mysql://localhost:3306/xsytest"); dataSource.setUsername(username); dataSource.setPassword(password); return dataSource; } }
第一步配置数据库
第二步 这步配置mybates数据要扫的包和数据类型印射,详细代码可复制于代码

第三步 servlet配置请求解析器

package com.ithm.config; import org.springframework.web.servlet.support.AbstractAnnotationConfigDispatcherServletInitializer; public class ServletConfig extends AbstractAnnotationConfigDispatcherServletInitializer { @Override protected Class<?>[] getRootConfigClasses() { return new Class[]{SpingConfig.class}; } @Override protected Class<?>[] getServletConfigClasses() { return new Class[]{SpringmvcConfig.class}; } @Override protected String[] getServletMappings() { return new String[]{"/"}; } }

package com.ithm.config; import org.springframework.context.annotation.ComponentScan; import org.springframework.context.annotation.Configuration; import org.springframework.context.annotation.Import; import org.springframework.context.annotation.PropertySource; @Configuration @ComponentScan("com.ithm.service") @PropertySource("classpath:Jdbc.properties") @Import({jdbcConfig.class,MybatiesConfig.class}) public class SpingConfig { }

package com.ithm.config; import org.springframework.context.annotation.ComponentScan; import org.springframework.context.annotation.Configuration; import org.springframework.web.servlet.config.annotation.EnableWebMvc; @Configuration @ComponentScan("com.ithm.controller") @EnableWebMvc public class SpringmvcConfig { }
最后差一人propetie配置文件

jdbc.driver = com.mysql.jdbc.Driver jdbc.url = jdbc:mysql://localhost:3306/xsytest jdbc.username = root jdbc.password = 142857
++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++配置结束,以下是工程文件+++
+++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++

import java.util.List; @RestController @RequestMapping("/book") public class BookController { @Autowired private BookService bookService; @PostMapping public boolean save(@RequestBody Book book) { return bookService.save(book); } @PutMapping public boolean update(@RequestBody Book book) { return bookService.update(book); } @DeleteMapping("/{id}") public boolean delete(@PathVariable Integer id) { return bookService.delete(id); } @GetMapping("/{id}") public Book getById(@PathVariable Integer id) { return bookService.getByid(id); } @GetMapping public List<Book> getAll() { return bookService.getAll(); } }

import org.apache.ibatis.annotations.Delete; import org.apache.ibatis.annotations.Insert; import org.apache.ibatis.annotations.Select; import org.apache.ibatis.annotations.Update; import org.springframework.stereotype.Service; import java.util.List; public interface BookDao { //@Insert("insert into tbl_book values(null,#{type},#{name})") @Insert("insert into tbl_book (type, name) values(null,#{type},#{name})") public void save(Book book); @Update("update tbl_book set type=#{type},name=#{name} where id = #{id}") public void update(Book book); @Delete("delete from tbl_book where id = #{id}") public void delete(Integer id); @Select("select * from tbl_book where id=#{id}") public Book getByid(Integer id); @Select("select * from tbl_book") public List<Book> getAll(); }

package com.ithm.domain; public class Book { private Integer id; private String type; private String name; public Integer getId() { return id; } public void setId(Integer id) { this.id = id; } public String getType() { return type; } public void setType(String type) { this.type = type; } public String getName() { return name; } public void setName(String name) { this.name = name; } @Override public String toString() { return "Book{" + "id=" + id + ", type='" + type + '\'' + ", name='" + name + '\'' + '}'; } }

package com.ithm.service.impl; import com.ithm.dao.BookDao; import com.ithm.domain.Book; import com.ithm.service.BookService; import org.springframework.beans.factory.annotation.Autowired; import org.springframework.stereotype.Service; import java.util.List; @Service public class BookServiceimpl implements BookService { @Autowired private BookDao bookDao; @Override public boolean save(Book book) { bookDao.save(book); return true; } @Override public boolean update(Book book) { bookDao.update(book); return true; } @Override public boolean delete(Integer id) { bookDao.delete(id); return true; } @Override public Book getByid(Integer id) { return bookDao.getByid(id); } @Override public List<Book> getAll() { return bookDao.getAll(); } }

package com.ithm.service; import com.ithm.domain.Book; import java.util.List; public interface BookService { public boolean save(Book book); public boolean update(Book book); public boolean delete(Integer id); public Book getByid(Integer id); public List<Book> getAll(); }
++++++++++++++++++++++++++++++++++++++++++++++++++++++最后编写测试类和postman工具测试+++++++++++++++++++++++++++

package com.ithm.text; import com.ithm.config.SpingConfig; import com.ithm.domain.Book; import com.ithm.service.BookService; import org.junit.Test; import org.junit.runner.RunWith; import org.springframework.beans.factory.annotation.Autowired; import org.springframework.test.context.ContextConfiguration; import org.springframework.test.context.junit4.SpringJUnit4ClassRunner; @RunWith(SpringJUnit4ClassRunner.class) @ContextConfiguration(classes = SpingConfig.class) public class servie { @Autowired private BookService bookService; @Test public void testgetbyid(){ Book book = bookService.getByid(1); System.out.println("ok"); System.out.println(book); } }
+++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++以上是junit测试++++++++++++++++++++++++++
如果是postMan接口测试需要:
如果是sava接口需要{“a”:"b","ee":"bb"} pust传这样格式数据上去 选择jsons格式
++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++=
开启事务:
需要在springConfig
1 新增注解 @EnableTransactionManagement
2 在jdbcConfig 中新增代码,事务管理器
@Bean
public PlatformTransactionManager platformTransactionManager(DataSource dataSource){
DataSourceTransactionManager ds = new DataSourceTransactionManager();
ds.setDataSource(dataSource);
return ds;
}
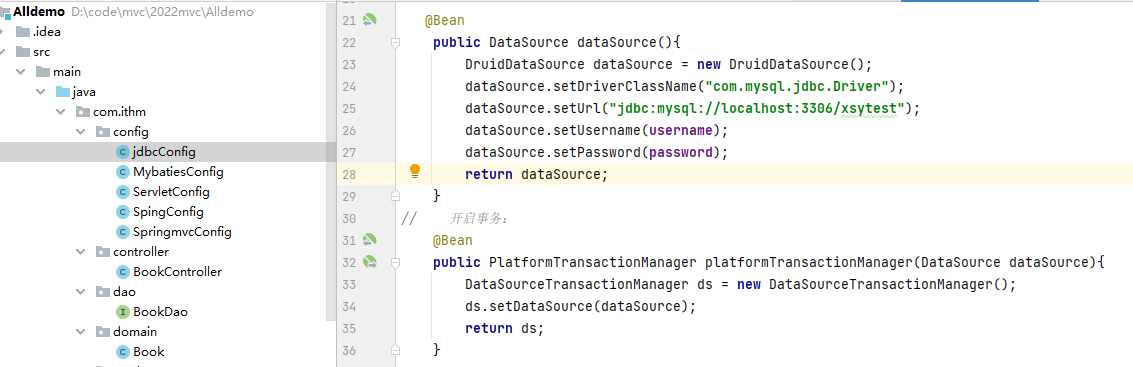
3,最后在servlet接口层上加事务 @Transactional
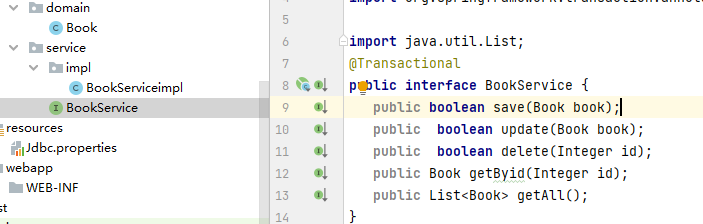