Prime Ring Problem
Problem Description
Note: the number of first circle should always be 1.
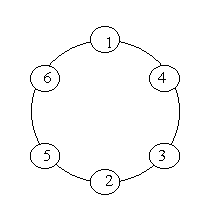
Input
Output
You are to write a program that completes above process.
Print a blank line after each case.
Sample Input
6 8
Sample Output
Case 1: 1 4 3 2 5 6 1 6 5 2 3 4 Case 2: 1 2 3 8 5 6 7 4 1 2 5 8 3 4 7 6 1 4 7 6 5 8 3 2 1 6 7 4 3 8 5 2
题意:给定一个数n求满足相邻元素的和值为素数的所有序列
题解:dfs
#include<stdio.h>
#include<math.h>
#include<string.h>
int n;
int arr[20];
int visit[20];
bool is(int n)
{
int i;
for(i=2;i<=(int)sqrt((double)(n));i++)
{
if(n%i==0) return false;
}
return true;
}
void dfs(int k)
{
int i;
if(k==n&&is(arr[1]+arr[n]))
{
for(i=1;i<n;i++)
{
printf("%d ",arr[i]);
}
printf("%d\n",arr[n]);
return;
}
for(i=2;i<=n;i++)
{
if(visit[i]==0&&is(arr[k]+i))
{
visit[i]=1;
arr[k+1]=i;
dfs(k+1);
visit[i]=0;
}
}
}
int main()
{
int i,ca=0;
while(scanf("%d",&n)!=EOF)
{
ca++;
printf("Case %d:\n",ca);
arr[1]=1;
memset(visit,0,sizeof(visit));
visit[1]=1;
dfs(1);
puts("");
}
return 0;
}