Java学习-018-EXCEL 文件写入实例源代码
众所周知,EXCEL 也是软件测试开发过程中,常用的数据文件导入导出时的类型文件之一,此文主要讲述如何通过 EXCEL 文件中 Sheet 的索引(index)或者 Sheet 名称获取文件中对应 Sheet 页中的数据。敬请各位小主参阅,若有不足之处,敬请大神指正,不胜感激!
不多言,小二上码咯。。。
通过 sheet_name 写入 EXCEL 数据源代码如下所示,敬请参阅!

1 /** 2 * @function 文件写入: EXCEL文件 3 * 4 * @author Aaron.ffp 5 * @version V1.0.0: autoUISelenium main.java.aaron.java.tools FileUtils.java excelWrite, 2014-11-25 16:03:35 Exp $ 6 * 7 * @param filename : EXCEL文件名称 8 * @param sheetname : EXCEL文件sheet名字 9 * @param excelData : EXCEL文件内容, 二维数组 10 * 11 * @return boolean 12 */ 13 public boolean excelWrite(String filename, String sheetname, ArrayList<String[]> excelData){ 14 FileOutputStream fos; // 文件输出流 15 WritableWorkbook excel; // Excel 工作薄 16 WritableSheet sheet; // Excel 工作表 17 Label label; // Excel 工作表单元格 Lable(col, row, content) 18 String[] colname; // Excel 工作表列头 19 String[] rowdata; // Excel 工作表行数据 20 // long start; 21 // long mid; 22 // long end; 23 boolean success = false; 24 25 // start = System.currentTimeMillis(); 26 27 /* 参数校验: 为null或空字符串时, 抛出参数非法异常 */ 28 if (filename == null || "".equals(filename) || !assertFileType(filename, "EXCEL")) { 29 throw new IllegalArgumentException(); 30 } 31 32 /* 若工作表名称为null或空, 则默认工作表名与工作薄名相同 */ 33 if (sheetname == null || "".equals(sheetname)) { 34 sheetname = filename; 35 } 36 37 /* Excel 最大行列校验: xls 最大行列:65536, 256; XLSX 最大行列:1048576, 16384 */ 38 if (filename.endsWith(".xls") && ((excelData.size() > 65536) || (excelData.get(0).length > 256))) { 39 this.message = "数据超过 " + filename + " 文件的最大行列【65536, 256】, 数据行列为【" + excelData.size() + ", " + excelData.get(0).length + "】"; 40 this.logger.warn(this.message); 41 42 return success; 43 } else if (filename.endsWith(".xlsx") && ((excelData.size() > 1048576) || (excelData.get(0).length > 16384))) { 44 this.message = "数据超过 " + filename + " 文件的最大行列【1048576, 16384】, 数据行列为【" + excelData.size() + ", " + excelData.get(0).length + "】"; 45 this.logger.warn(this.message); 46 47 return success; 48 } 49 50 try { 51 fos = new FileOutputStream(filename); // 创建文件输出流 52 excel = Workbook.createWorkbook(fos); // 创建 Excel 工作薄 53 sheet = excel.createSheet(sheetname, 0); // 创建 Excel 工作表 54 55 /* 若数据为空, 则仅创建 Excel 文件 */ 56 if (excelData.isEmpty() || excelData.size() == 0) { 57 excel.write(); 58 excel.close(); 59 fos.close(); 60 61 success = true; 62 return success; 63 } 64 65 /* 数据不为空, 则将数据第一行默认视作列头 */ 66 colname = excelData.get(0); 67 68 /* 写入工作表列头 */ 69 for (int i = 0; i < colname.length; i++) { 70 label = new Label(i, 0, colname[i], this.excelSetCellCssForColname()); 71 sheet.addCell(label); 72 } 73 74 /* 遍历数组, 写入数据 */ 75 for (int i = 1; i < excelData.size(); i++) { // 行处理 76 rowdata = excelData.get(i); 77 78 for (int j = 0; j < colname.length; j++) {// 列处理 79 label = new Label(j, i, rowdata[j], this.excelSetCellCss()); 80 sheet.addCell(label); 81 } 82 } 83 84 // mid = System.currentTimeMillis(); 85 86 excel.write(); 87 excel.close(); 88 fos.close(); 89 90 // end = System.currentTimeMillis(); 91 92 // System.out.println("Excel {" + filename + "} 数据解析共耗时 " + (mid - start) + " s"); 93 // System.out.println("Excel {" + filename + "} 数据写入共耗时 " + (end - mid) + " s"); 94 // System.out.println("Excel {" + filename + "} 数据写入共耗时 " + (end - start) + " s"); 95 96 success = true; 97 } catch (FileNotFoundException fnfe) { 98 this.message = "文件 {" + filename + "} 不存在!"; 99 this.logger.error(this.message, fnfe); 100 } catch (RowsExceededException ree) { 101 this.message = "文件 {" + filename + "} 写入失败!"; 102 this.logger.error(this.message, ree); 103 } catch (WriteException we) { 104 this.message = "文件 {" + filename + "} 写入失败!"; 105 this.logger.error(this.message, we); 106 } catch (IOException ioe) { 107 this.message = "文件 {" + filename + "} 写入失败!"; 108 this.logger.error(this.message, ioe); 109 } 110 111 return success; 112 }
输出的 excel 文件如下所示:
上述代码中调用的设置 EXCEL 文件列头样式的源码如下所示,敬请参阅!

1 /** 2 * @function 设置EXCEL列头样式 3 * 4 * @author Aaron.ffp 5 * @version V1.0.0: autoUISelenium main.java.aaron.java.tools FileUtils.java excelSetCellCssForColname, 2014-11-25 5:52:42 Exp $ 6 * 7 * @return WritableCellFormat 8 */ 9 public WritableCellFormat excelSetCellCssForColname(){ 10 WritableCellFormat cssFormat; 11 WritableFont font; 12 13 /* 设置字体, 大小, 字形 */ 14 font = new WritableFont(WritableFont.createFont("Courier New"), 20, WritableFont.BOLD); 15 16 try { 17 font.setColour(Colour.BLUE); // 设置字体颜色 18 } catch (WriteException we) { 19 we.printStackTrace(); 20 } 21 22 /* 设置单元格格式布局 */ 23 cssFormat = new WritableCellFormat(font); 24 25 try { 26 cssFormat.setAlignment(jxl.format.Alignment.CENTRE); // 水平居中 27 cssFormat.setVerticalAlignment(jxl.format.VerticalAlignment.CENTRE); // 垂直居中 28 cssFormat.setBorder(Border.ALL, BorderLineStyle.THIN, Colour.BLACK); // 黑色边框 29 cssFormat.setBackground(Colour.YELLOW); // 黄色背景 30 31 return cssFormat; 32 } catch (WriteException we) { 33 this.message = "设置 excel 列头样式失败!"; 34 this.logger.error(this.message, we); 35 } 36 37 return cssFormat; 38 }
调用设置 EXCEL 文件单元格样式的源码如下所示,敬请参阅!

1 /** 2 * @function 设置EXCEL单元格样式 3 * 4 * @author Aaron.ffp 5 * @version V1.0.0: autoUISelenium main.java.aaron.java.tools FileUtils.java excelSetCellCss, 2014-11-25 17:53:35 Exp $ 6 * 7 * @return WritableCellFormat 8 */ 9 public WritableCellFormat excelSetCellCss(){ 10 WritableCellFormat cssFormat; 11 WritableFont font; 12 13 /* 设置字体, 大小, 字形 */ 14 font = new WritableFont(WritableFont.createFont("Courier New"), 16, WritableFont.NO_BOLD); 15 16 try { 17 font.setColour(Colour.BLUE); // 设置字体颜色 18 } catch (WriteException we) { 19 we.printStackTrace(); 20 } 21 22 /* 设置单元格格式布局 */ 23 cssFormat = new WritableCellFormat(font); 24 25 try { 26 cssFormat.setAlignment(jxl.format.Alignment.LEFT); // 水平靠左 27 cssFormat.setVerticalAlignment(jxl.format.VerticalAlignment.CENTRE); // 垂直居中 28 cssFormat.setBorder(Border.ALL, BorderLineStyle.THIN, Colour.BLACK); // 黑色边框 29 30 return cssFormat; 31 } catch (WriteException we) { 32 this.message = "设置 excel 单元格格式失败!"; 33 this.logger.error(this.message, we); 34 } 35 36 return cssFormat; 37 }
Excel 文件写入测试脚本如下所示,敬请参阅!

1 /** 2 * Test : excel write 3 * 4 * @author Aaron.ffp 5 * @version V1.0.0: autoUISelenium test.java.aaron.java.tools FileUtilsTest.java test_excelWrite, 2014-11-25 16:23:35 Exp $ 6 * 7 */ 8 @Test 9 public void test_excelWrite(){ 10 this.message = "\n\n\nTEST:FileUtils.excelWrite(String filename, String sheetname, ArrayList<String[]> excelData)"; 11 this.logger.debug(this.message); 12 13 this.fu = new FileUtils(); 14 String filename = this.constantslist.PROJECTHOME + this.constantslist.FILESEPARATOR + 15 "testng-temp" + this.constantslist.FILESEPARATOR + "excelWrite.xls"; 16 17 ArrayList<String[]> exceldata = new ArrayList<String[]>(); 18 String[] rows; 19 20 // init excel data 21 for (int i = 0; i < 20; i++) { 22 rows = new String[10]; 23 24 for (int j = 0; j < rows.length; j++) { 25 rows[j] = i + " = " + j; 26 } 27 28 exceldata.add(rows); 29 } 30 31 boolean w = this.fu.excelWrite(filename, "excelWrite", exceldata); 32 boolean r = this.fu.excelReadByName(filename, "excelWrite", 0).get(5)[7].equals("5 = 7"); 33 34 Assert.assertEquals(w, r, "Test case failed."); 35 }
测试源码执行结果如下所示:
至此, Java学习-018-EXCEL 文件写入实例源代码 顺利完结,希望此文能够给初学 Java 的您一份参考。
最后,非常感谢亲的驻足,希望此文能对亲有所帮助。热烈欢迎亲一起探讨,共同进步。非常感谢! ^_^
欢迎 【 留言 || 关注 || 打赏 】 。您的每一份心意都是对我的鼓励和支持!非常感谢!欢迎互加,相互交流学习!
作者:范丰平,本文链接:https://www.cnblogs.com/fengpingfan/p/4602934.html
Copyright @范丰平 版权所有,如需转载请标明本文原始链接出处,严禁商业用途! 我的个人博客链接地址:http://www.cnblogs.com/fengpingfan
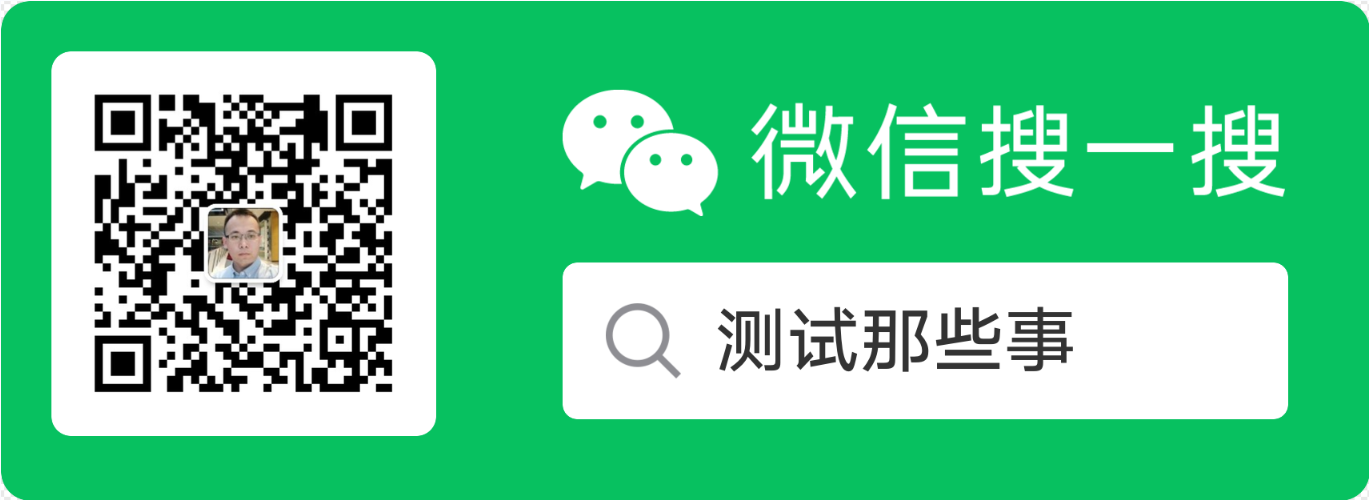