Selenium2学习-009-WebUI自动化实战实例-007-Selenium 8种元素定位实战实例源代码(百度首页搜索录入框及登录链接)
此 文主要讲述用 Java 编写 Selenium 自动化测试脚本编写过程中,通过 ID、name、xpath、cssSelector、linkText、className、partialLinkText、tagName 的实战实例源代码演示 Selenium 元素定位的 8 种方法,源代码测试通过日期为:2015-01-26 00:18,请知悉。注意:其中通过 tagName 定位元素时,返回的是一个 WebElement 的数组,需要根据实际的测试需求对其进行进一步的处理。
希望能对初学 Selenium2 WebUI 自动化测试编程的亲们有所帮助。若有不足之处,敬请大神指正,不胜感激!
以下为 Selenium 的 8 种元素定位的 Java 自动化测试脚本实例源代码,敬请参阅!

1 /** 2 * Aaron.ffp Inc. 3 * Copyright (c) 2004-2015 All Rights Reserved. 4 */ 5 package main.java.aaron.sele.demo; 6 7 import java.util.Arrays; 8 import java.util.List; 9 import java.util.concurrent.TimeUnit; 10 11 import org.openqa.selenium.By; 12 import org.openqa.selenium.InvalidElementStateException; 13 import org.openqa.selenium.NoSuchElementException; 14 import org.openqa.selenium.WebDriver; 15 import org.openqa.selenium.WebElement; 16 import org.openqa.selenium.chrome.ChromeDriver; 17 import org.openqa.selenium.chrome.ChromeOptions; 18 import org.openqa.selenium.remote.DesiredCapabilities; 19 20 /** 21 * UI自动化功能测试脚本:元素定位示例-通过百度首页搜索框及登录链接实例演示 22 * 1.通过 ID 查找元素 23 * 2.通过 name 查找元素 24 * 3.通过 xpath 查找元素 25 * 4.通过 cssSelector 查找元素 26 * 5.通过 linkText 查找元素 27 * 6.通过 className 查找元素 28 * 7.通过 partialLinkText 查找元素 29 * 8.通过 tagName 查找元素(查找的结果为元素数组) 30 * 31 * @author Aaron.ffp 32 * @version V1.0.0: autoUISelenium main.java.aaron.sele.demo Selenium_LocationDemo.java, 2015-1-25 22:59:55 Exp $ 33 */ 34 public class Selenium_LocationDemo { 35 36 private static WebDriver webdriver; 37 private static String baseUrl; // 百度首页网址 38 private static WebElement search; // 百度搜索框-页面元素 39 private static String element_id; // 百度搜索框-ID 40 private static String element_name; // 百度搜索框-name 41 private static String element_xpath; // 百度搜索框-xpath 42 private static String element_cssSelector; // 百度搜索框-cssSelector 43 private static String element_linkText; // 登录链接-linkText 44 private static String element_className; // 百度搜索框-className 45 private static String element_partialLinkText; // 登录链接-partialLinkText 46 private static String element_tagName; // 百度搜索框-tagName 47 48 /** 49 * Chrome WebDriver 设置, 网址及搜索内容初始化, 打开 Chrome 浏览器 50 * 51 * @author Aaron.ffp 52 * @version V1.0.0: autoUISelenium main.java.aaron.sele.demo Selenium_LocationDemo.java chromeStart, 2015-1-25 23:03:38 Exp $ 53 * 54 */ 55 public static void chromeStart(){ 56 /* 设定 chrome 启动文件的位置, 若未设定则取默认安装目录的 chrome */ 57 System.setProperty("webdriver.chrome.bin", "C:/Program Files (x86)/Google/Chrome/Application/chrome.exe"); 58 /* 设定 chrome webdirver 的位置 */ 59 System.setProperty("webdriver.chrome.driver", "C:/Windows/System32/chromedriver.exe"); 60 /* 百度首页网址赋值 */ 61 baseUrl = "http://www.baidu.com/"; 62 63 element_id = "kw"; // 百度搜索框-ID 64 element_name = "wd"; // 百度搜索框-name 65 element_xpath = "//input[@id='kw']"; // 百度搜索框-xpath 66 element_cssSelector = ".s_ipt"; // 百度搜索框-cssSelector 67 element_linkText = "登录"; // 登录链接-linkText 68 element_className = "s_ipt"; // 百度搜索框-className 69 element_partialLinkText = "录"; // 登录链接-partialLinkText 70 element_tagName = "input"; // 百度搜索框-tagName 71 72 /* 启动 chrome 浏览器 */ 73 webdriver = new ChromeDriver(chromeOptions()); 74 } 75 76 /** 77 * 主方法入口 78 * @author Aaron.ffp 79 * @version V1.0.0: autoUISelenium main.java.aaron.sele.demo Selenium_LocationDemo.java main, 2015-1-25 22:59:55 Exp $ 80 * 81 * @param args 82 * @throws InterruptedException 83 */ 84 public static void main(String[] args) throws InterruptedException { 85 /* 启动 chrome */ 86 chromeStart(); 87 /* 打开百度 */ 88 webdriver.get(baseUrl); 89 /* 等待加载 */ 90 webdriver.manage().timeouts().implicitlyWait(5, TimeUnit.SECONDS); 91 92 /* 通过 ID 查找元素 */ 93 try { 94 search = webdriver.findElement(By.id(element_id)); 95 search.clear(); 96 search.sendKeys("通过 ID 查找元素"); 97 } catch (NoSuchElementException nsee) { 98 nsee.printStackTrace(); 99 } 100 101 TimeUnit.SECONDS.sleep(5); 102 103 /* 通过 name 查找元素 */ 104 try { 105 search = webdriver.findElement(By.name(element_name)); 106 search.clear(); 107 search.sendKeys("通过 name 查找元素"); 108 } catch (NoSuchElementException nsee) { 109 nsee.printStackTrace(); 110 } 111 112 TimeUnit.SECONDS.sleep(5); 113 114 /* 通过 xpath 查找元素 */ 115 try { 116 search = webdriver.findElement(By.xpath(element_xpath)); 117 search.clear(); 118 search.sendKeys("通过 xpath 查找元素"); 119 } catch (NoSuchElementException nsee) { 120 nsee.printStackTrace(); 121 } 122 123 TimeUnit.SECONDS.sleep(5); 124 125 /* 通过 cssSelector 查找元素 */ 126 try { 127 search = webdriver.findElement(By.cssSelector(element_cssSelector)); 128 search.clear(); 129 search.sendKeys("通过 cssSelector 查找元素"); 130 } catch (NoSuchElementException nsee) { 131 nsee.printStackTrace(); 132 } catch (InvalidElementStateException iese){ 133 iese.printStackTrace(); 134 } 135 136 TimeUnit.SECONDS.sleep(5); 137 138 /* 通过 linkText 查找元素 */ 139 try { 140 search = webdriver.findElement(By.linkText(element_linkText)); 141 System.out.println(search.getText());; 142 } catch (NoSuchElementException nsee) { 143 nsee.printStackTrace(); 144 } 145 146 TimeUnit.SECONDS.sleep(5); 147 148 /* 通过 className 查找元素 */ 149 try { 150 search = webdriver.findElement(By.className(element_className)); 151 search.clear(); 152 search.sendKeys("通过 className 查找元素"); 153 } catch (NoSuchElementException nsee) { 154 nsee.printStackTrace(); 155 } 156 157 TimeUnit.SECONDS.sleep(5); 158 159 /* 通过 partialLinkText 查找元素 */ 160 try { 161 search = webdriver.findElement(By.partialLinkText(element_partialLinkText)); 162 System.out.println(search.getText()); 163 } catch (NoSuchElementException nsee) { 164 nsee.printStackTrace(); 165 } 166 167 TimeUnit.SECONDS.sleep(5); 168 169 /* 通过 tagName 查找元素 170 * 此种方法返回的为一个 WebElement 数组,需要根据测试需求进行相应的处理 171 */ 172 try { 173 webdriver.get(baseUrl); 174 175 List<WebElement> tagName = webdriver.findElements(By.tagName(element_tagName)); 176 177 for (WebElement webElement : tagName) { 178 if ("wd".equals(webElement.getAttribute("name"))) { 179 webElement.sendKeys("通过 tagName 查找元素"); 180 } 181 } 182 } catch (NoSuchElementException nsee) { 183 nsee.printStackTrace(); 184 } catch (Exception e){ 185 e.printStackTrace(); 186 } 187 188 /* 关闭 chrome */ 189 // chromeQuit(); 190 } 191 192 /** 193 * 设置 Chrome 浏览器的启动参数, 设置启动后浏览器窗口最大化, 忽略认证错误警示 194 * 195 * @author Aaron.ffp 196 * @version V1.0.0: autoUISelenium main.java.aaron.sele.demo Selenium_LocationDemo.java chromeOptions, 2015-1-25 23:03:54 Exp $ 197 * 198 * @search = ChromeOptions Chrome 参数设置 199 */ 200 public static ChromeOptions chromeOptions(){ 201 ChromeOptions options = new ChromeOptions(); 202 DesiredCapabilities capabilities = DesiredCapabilities.chrome(); 203 capabilities.setCapability("chrome.switches", Arrays.asList("--start-maximized")); 204 /* 浏览器最大化 */ 205 options.addArguments("--test-type", "--start-maximized"); 206 /* 忽略 Chrome 浏览器的认证错误 */ 207 options.addArguments("--test-type", "--ignore-certificate-errors"); 208 209 return options; 210 } 211 212 /** 213 * 关闭并退出 Chrome 214 * 215 * @author Aaron.ffp 216 * @version V1.0.0: autoUISelenium main.java.aaron.sele.demo Selenium_LocationDemo.java chromeQuit, 2015-1-25 23:04:19 Exp $ 217 */ 218 public static void chromeQuit(){ 219 /* 关闭 chrome */ 220 webdriver.close(); 221 /* 退出 chrome */ 222 webdriver.quit(); 223 } 224 }
至此,WebUI 自动化功能测试脚本第 007 篇-Selenium 8种元素定位实战实例源代码(百度首页搜索录入框及登录链接) 顺利完结,希望此文能够给初学 Selenium 的您一份参考。
最后,非常感谢亲的驻足,希望此文能对亲有所帮助。热烈欢迎亲一起探讨,共同进步。非常感谢! ^_^
欢迎 【 留言 || 关注 || 打赏 】 。您的每一份心意都是对我的鼓励和支持!非常感谢!欢迎互加,相互交流学习!
作者:范丰平,本文链接:https://www.cnblogs.com/fengpingfan/p/4249251.html
Copyright @范丰平 版权所有,如需转载请标明本文原始链接出处,严禁商业用途! 我的个人博客链接地址:http://www.cnblogs.com/fengpingfan
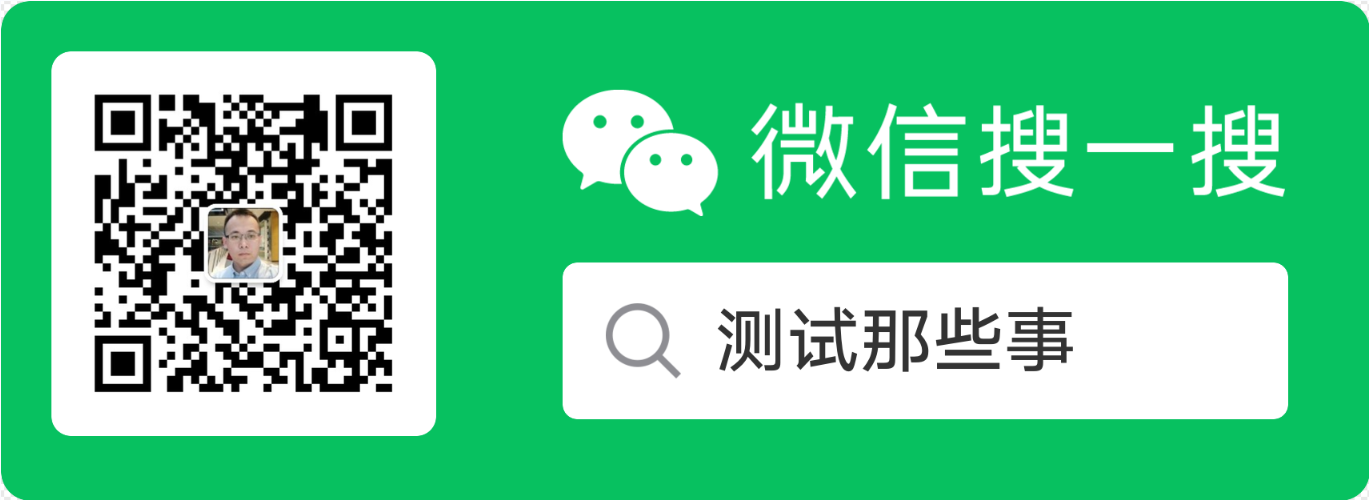