leetcode3
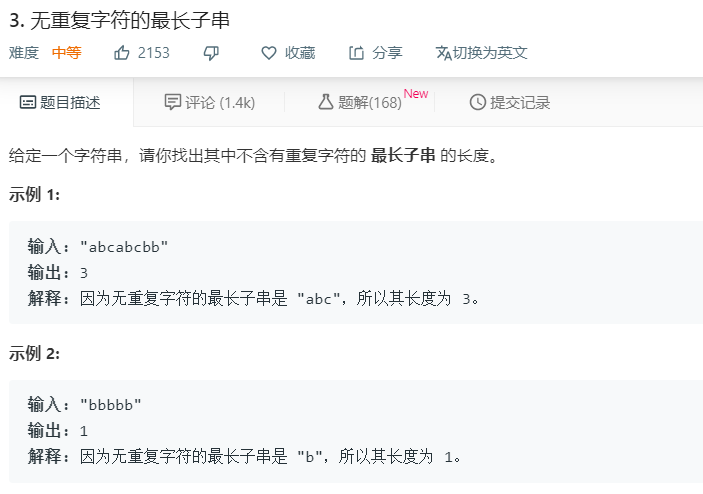
class Solution():
def lengthOfLongestSubstring(self, s):
l = [] # 记录子串的容器
res = [] # 记录字串的长度
for x in range(s): # 遍历字符串
if x not in l: # 一直追加到不重复的子串
l.append(x)
else: # 重复字符
res.append(len(l)) # 记录子串的长度
i = l.index(l) # 记录子串中在添加元素重复的字符位置
l = l[i+1:] # 更新子串
l.append(x) #加上字符
res.append(len(l)) # 记录最后一个不重复子串长度
return max(res) if res else 0
leetcode 11
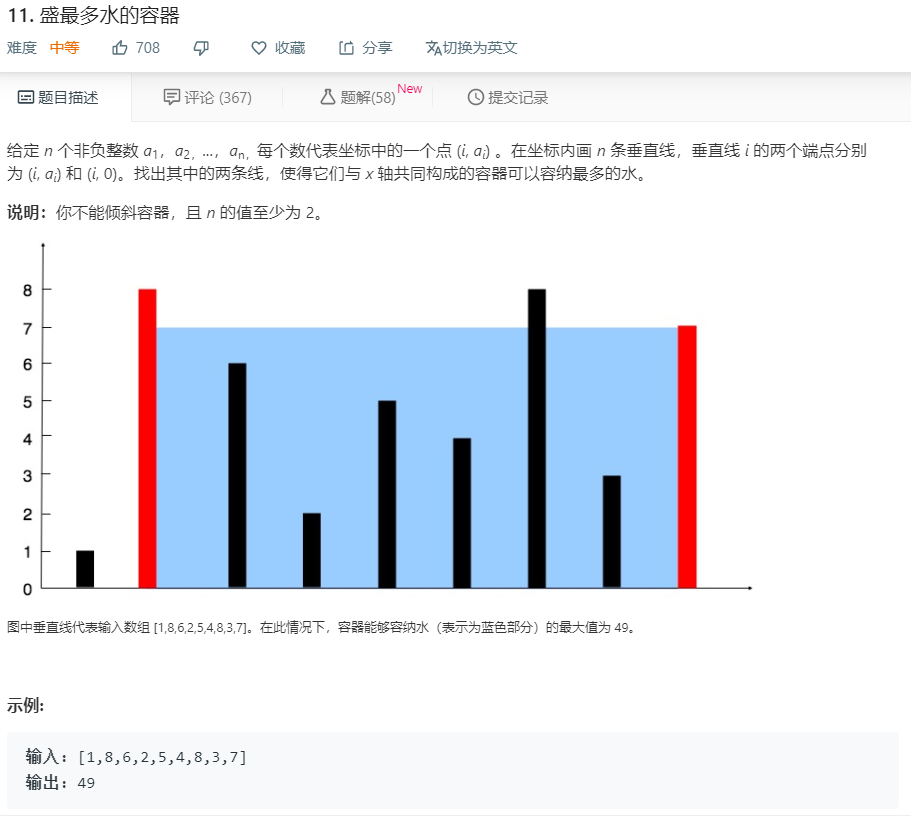
class Solution(object):
def maxArea(self, height):
"""
:type height: List[int]
:rtype: int
"""
left = 0 # 双指针,左和右
right = len(height) - 1
maxArea = 0 # 初始面积为0
while left < right: # 左边 不能超过到右边
b = right - left # 水池的宽
if height[left] < height[right]: # 短板为高
h = height[left]
left += 1 # 移动短板边
else:
h = height[right]
right -= 1
area = b*h # 求面积
if maxArea < area: # 面积变大即更新
maxArea = area
return maxArea