原生技巧篇
name
let msy='a';
msy.name='xxx'
console.log(msy);
//undefined
非严格模式返回 undefined
,严格模式报错
==
10 == [[[10]]]
+[[[10]]]
//不管多少层,都是转成10
解构
const arr = [{age: 12}, {age: 15}]
for (const {age} of arr) {
console.log(age);
}
函数里面这个大括号是局部作用域
function add1() {
let b = 11;
{
const a = 10;
console.log(a, b);
}
{
const a=11;
console.log(a);
}
}
console.log
console.log('x = %d,y = %s', 10,'aaa');
// x = 10,y=aaa
数组
const obj = {
[Symbol.iterator]() {
const it = [1, 2].values();
return {
next() {
return it.next();
}
}
}
}
for (let item of obj) {
console.log(item);
}
// 1,2
//值
const a = [1,2,3].values();
console.log(a.next());
// (value: 1, done: false)
// 索引
const b = [4,5, 6].keys();
console.log(b.next());
// { value: 0, done: false ]
const c = [7,8, 9].entries();
console.log(c.next());
// { value: [ 0,7 ], done: false ]
链表的有趣实践
let obj = {val: 'xxx'};
let obj1 = obj;
obj.next = [val: 123];
obj = obj.next;
obj.next = {val: 456};
obj=obj.next;
console.log(obj);
console.log(obj1);
删除对象属性
//删除单个属性
const deleteProp = (name, {[name]: propValuc, ..obj}) => {
return obj
}
// deleteProp('a',{a: 1, b: 2,c: 4})
//删除多个属性
const removeProp = (obj,...keys) => {
return keys.length ? removeProp(deleteProp(keys.pop(), obj)) : obj
}
//
精度问题
Math.round(num*108)/100
// 1.005 等问题的精度问题
Math.round((num+Number.EPSILON)*100)/100
//尾数
const randTo = (x, num) => +(Math.round(x + `e+${num}`) + `e-${num}`);
// e+ 可以简写成 e
console.log(randTo(1.125,2));
console.log(randTo(1.1255,3));
true的问题字符串和不带字符串
JSON.parse('true') === true
// true
JSON.parse(true) === true
// false
对象排序
const arr = [{age: 'a'},{age: 'c'}, {age: 'b'}]
// 不够完整
arr.sort((a, b) => a.age > b.age ? 1 : -1)
// 完整点
arr.sort((a, b) => {
if (a.age > b.age) {
return 1
}
return a.age < b.age ? -1 : 0;
})
arr.sort((a,b) => a.age.localeCompare(b.age))
//
for await
const add = async () =>{
for await (const item of
[
new Promise(res=> setTimeout(() => {
res('成功')
},2000)),
Promise.resolve('ch')
]){
console.log(item);
}
}
检测字符串是否是数字
const isNumeric = str => {
if (typeof str !== 'string') {
return false
}
return !isNaN(str) && !isNaN(parseFloat(str));
}
// isNaN要检查变量(包括字符串)是否是数字
// 不能用 + 强转,因为·, 会转化为8
// 不能用parseInt()10px’会转成10 ,'xx1Bpx' 会转成NaN
console.log 打印看不到完整的
const obj = {sex: 1, children: [{aa: 1, obj: {sex: 1}}]}
console.log('%j', obj)
页面是否活跃,切换浏览器标题栏
document.addEventListener('visibilitychange',e => {
if(document.hidden){
console.log('页面隐藏');
}else{
console.log('页面显示');
}, false)
Array.from
Array.from('abc')
// ['a','b','c']
禁用滚动
function disableScrolling() {
const x = window.scrollX;
const y = window.scrollY;
windon.onscroll = function (){
window.scrollTo(x,y);
}
}
// 解除禁用
function enableScrolling(){
windaw.onscroll = null;
}
去掉小数位,多余的0
+(1).toFiexd(2)
// 拿到值进行强转数字
不能选中的文字
css
p{
user-select:none;
}
// 正常呢
user-select:text
.yl-add{
content:attr(data-text);
}
<div class="yl-add" data-text="我是一个测试的选中"></div>
js
<div onselectstart="return false">我是一个不能选中的字段</div>
去掉生产环境中的console.log
直接把它设置成一个空函数
console.log=function(){}
ts技巧
interface Comparator<T> {
compareTo(value: T): number;
}
interface Obj {
name: string;
age: number;
}
class Rectangle implements Comparator<Obj>{
compareTo(value: Obj): number {
return 1
}
}
让滚动平滑点
window.scrollTo({
top:100,
behavior:'smooth'
})
点击的时候调用对象
<div onclick="clickMethod(this)">123</div>
t.addEventListener('click', e => {});
<button onclick="clickScroll();console.log(1)">Click</button>
取消调试模式
控制台Sources模式,右上角 等不于
符号选中就是取消调试模式
ctrl+ F8
键盘判断的时候不推荐使用 keyCode
MDN说 .keyCode
已经被弃用
推荐使用 event.key
和 event.code
在 <input type=number>
滚动时候,禁用
document.addEventListener('wheel', event => {
if (document.activeElement.type === 'number') {
document.activeElement.blur();
}
})
刷新页面回到顶部
window.onbeforeunload=()=>{
window.scrollTo(0,0)
}
flatMap
const arr=[
{id:1,country:[
{id:'111'},
{id:'333'}
]},
{id:2,country:[
{id:'222'},
{id:'333'}
]},
{id:1,country:[
{id:'444'},
{id:'555'}
]},
{id:2,country:[
{id:'666'},
{id:'777'}
]}
l
去掉小数部分
const X=12.5012
console.log(~~X)
.toFixed()
//不过会四舍五入
平方每一个数字
const squareDigits = n => {
return String(n).replace(/./g, s => {
return s**2
})
}
Array.from(String(n),v =>{
return v ** 2
}).join('')
硬币问题
父亲把一堆硬币分配给三个小孩,如果都京到相同金额的钱,返回为true,否则为false
const coinsDiv = arr => {
arr.sort((a,b) => a - b);
const sum = arr.reduce((acc, val) => ace + val, 0);
const item = sum / 3;
const itemArr = Array(3).fill(item);
for (let i=0;i<3;i++){
while (itemArr[i]) {
// 最大值
let index = arr.findIndex(val => val === itemArr[i]);
//最小值
if(index===-1) index = arr.findIndex(val => val < itemArr[i]);
//如用还没有就反回false
if(index==--1) return false
itemArr[i] -= arr.splice(index,1);
}
}
return true
}
一种奇特的转化为二进制
const n=10;
console.log(n.toString(2));
// 1010
parseInt(1010,2)
// 10
+('0b' +'1010')
// 18
数组作为字符串列表保留引号
const arr=['a','b','c']
const str = `'` + arr.join(`','`)+ `'`;
undefined
undefined 在压缩的时候会编译成 void 0
筛选空格
'a,b,c!'.split(/\s*, \s*/)
几道有趣的面试题
设计一个函数 f(f(n)) 结果等于 -n
const fn = (n) => {
if (typeof n === 'function') {
return n();
}
return O => {
return -n
}
}
console.log(fn(fn(10)));
为什么x(x=y)和(x=y)x 不同
括号并不表示求值顺序,求值顺序是从左到右,与括号无关
运算符的规则 ==
是: 计算左边产生一个值,计算右边产生一个只,比较值
(x=y)==x
左侧的规则发生在所以评估右侧的规则之前
交换两个数字
const swarp = (x, y) => {
x^=y;
y^=x;
x^=y;
}
折叠动画
修复延迟方案
<style>
.text {
overfLow: hidden;
max-height: 0;
transition: max-height .5s cubic-bezier(0, 1, 0,1);
}
.text.full {
max-height: 1000px;
transition: max-height is ease-in-out;
}
</style>
<div id="aaa" class='text'>
<div style="height: 400vh;background-color: firebrick;"></div>
</div>
CSS
边框图像源
.container {
width: 200px;
height: 200px;
border-width:2rem;
border-style:dotted;
border-color:grey;
// 边框的图像文件
border-image-source:url("./阔景(10).jpg");
// 重复图像
border-image-repeat: repeat;
//用于间隔和调整图像大小的区域
border-image-slice: 100;
}
决定自己的高度的是你的态度,而不是你的才能
记得我们是终身初学者和学习者
总有一天我也能成为大佬
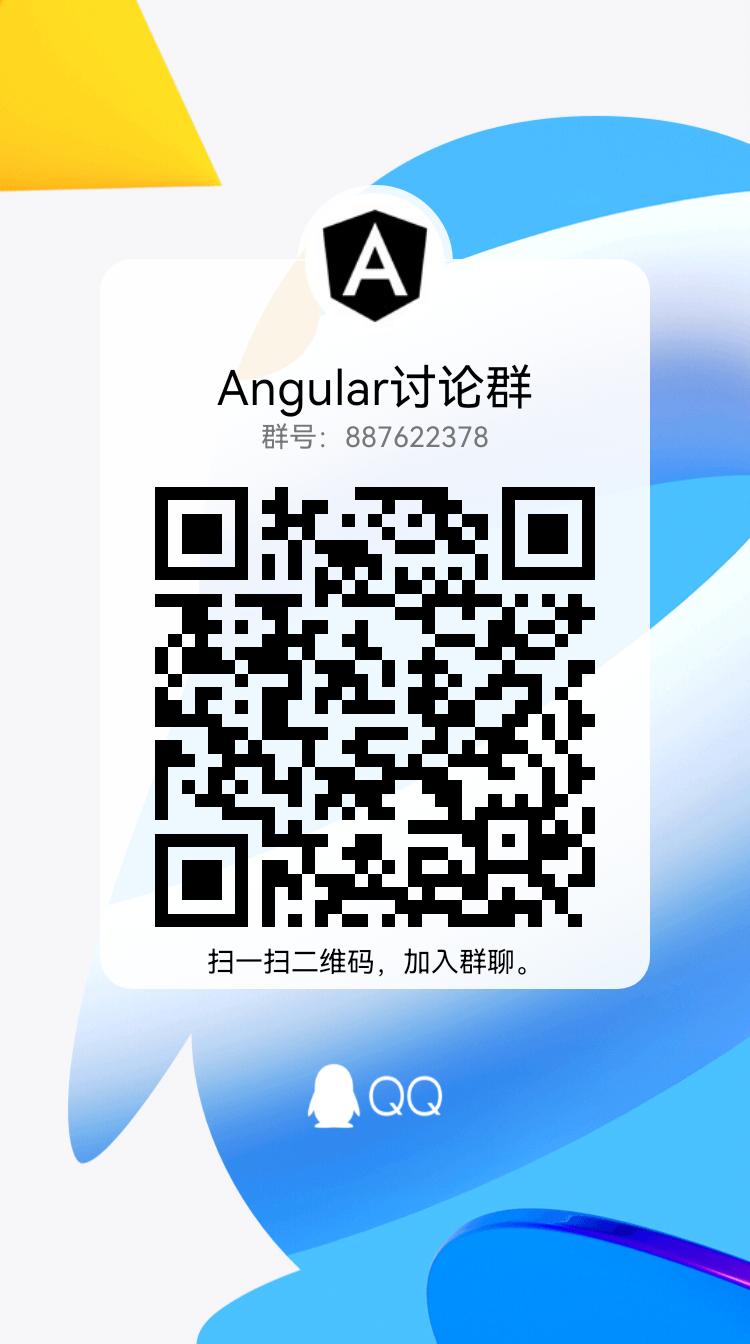