react复习(一)
- Neutrino 把 webpack 的强大功能和简单预设结合在一起。并且包括了 React 应用和 React 组件的预设。
- Nx 是针对全栈 monorepo 的开发工具包,其内置了 React,Next.js,Express 等。
- Parcel 是一个快速的、零配置的网页应用打包器,并且可以搭配 React 一起工作。
- Razzle 是一个无需配置的服务端渲染框架,但它提供了比 Next.js 更多的灵活性。
- Gatsby 是用 React 创建静态网站的最佳方式
操作dom
componentDidMount() { }
离开页面
componentWillMount() { }
State
的更新可能是异步的
this.setState({
[name]: value
});
this.setState({
counter: this.state.counter + this.props.increment,
});
this.setState((state, props) => ({
counter: state.counter + props.increment
}));
事件处理
clickMethodsOne(){
console.log(1);
}
<button onClick={this.clickMethodsOne}>Click</button>
---------------
handleClick = () => {
console.log('this is:', this);
}
<button onClick={this.clickMethodsOne}>Click</button>
---------------
<button onClick={() => this.handleClick()}>
Click me
</button>
阻止组件渲染
可以让 render 方法直接返回 null,而不进行任何渲染。
循环
{a.map((v,i)=>{
return <li key={i}>{v}</li>
})}
idea插件
React snippets
rcc class rcf 比较完整的 rsc 函数表达式 rsf cref refs 用
插槽
<Two >
<p>我是一个p</p>
</Two>
这个是不是默认插槽
{this.props.children}
是不是特别像插槽
插槽函数形式
<Seven >
{
()=>{
return <h1>我是函数里面的</h1>
}
}
</Seven>
{this.props.children()}
子传递参数进行判断
传递参数
{
(num) => {
if (num == 1) {
return <h1>我是函数里面的1</h1>
}
return <h1>我是函数默认的</h1>
}
}
{this.props.children(1)}
{this.props.children()}
传递参数到子组件
<Seven render={num => {
return <div>
<Two two={num}/>
</div>
}} />
{this.props.render(10)}
其实可以自定义属性传递
<Amount
renderAmountOne={amount => (
<div>
<h2>My one Amount</h2>
<Pound amount={amount} />
<Euro amount={amount} />
</div>
)}
renderAmountTwo={amount => (
<div>
<h2>My other Amount</h2>
<Pound amount={amount} />
<Euro amount={amount} />
</div>
)}
/>
{this.props.renderAmountTwo(10)}
{this.props.renderAmountOne(20)}
命名插槽
<SplitPane
left={
<Contacts />
}
right={
<Chat />
} />
{props.left}
{props.right}
Fragment
<Fragment> sss </Fragment>
{a.map((v,i)=>{
return <Fragment key={i}>
{v}
</Fragment>
})}
<> </>
请注意 for
在 JSX 中应该被写作 htmlFor
<label htmlFor="namedInput">Name:</label>
<input id="namedInput" type="text" name="name"/>
ref
constructor(props) {
super(props);
this.textP = createRef();
}
<input type="text" ref={this.textP}/>
componentDidMount() {
// 获取Dom失去焦点
this.textP.current.focus()
}
a链接要这样拿
let anchorRef = createRef()
<Link to="/" innerRef={anchorRef} />
动态分割
import("./math").then(math => {
console.log(math.add(16, 26));
});
const OtherComponent = React.lazy(() => import('./OtherComponent'));
异步加载添加加载的状态
懒加载组件
const OtherComponent = React.lazy(() => import('./OtherComponent'));
<Suspense fallback={<div>Loading...</div>}>
<OtherComponent />
</Suspense>
可以包裹多个组件
<Suspense fallback={<div>Loading...</div>}>
<section>
<OtherComponent />
<AnotherComponent />
</section>
</Suspense>
点语法
const MyComponents = {
DatePicker: function DatePicker(props) {
return <div>Imagine a {props.color} datepicker here.</div>;
}
}
function BlueDatePicker() {
return <MyComponents.DatePicker color="blue" />;
}
定义的组件必须以大写字母开头
props 默认true
<MyTextBox autocomplete />
等价
<MyTextBox autocomplete={true} />
-----
扩展语法
function App1() {
return <Greeting firstName="Ben" lastName="Hector" />;
}
function App2() {
const props = {firstName: 'Ben', lastName: 'Hector'};
return <Greeting {...props} />;
}
这样传递参数
function Hello(props) {
return <div>Hello {props.addressee}!</div>;
}
添加一个弹框
class Modal extends Component {
constructor(props) {
super(props);
this.el = document.createElement('div')
this.dom = document.querySelector('#root')
}
componentDidMount() {
this.dom.appendChild(this.el)
}
componentWillUnmount() {
this.dom.removeChild(this.el)
}
render() {
return ReactDOM.createPortal(this.props.children, this.el)
}
}
<Modal>
<h1>232332</h1>
<h1>232332</h1>
<h1>232332</h1>
</Modal>
props 声明默认属性
class Greeting extends React.Component {
// ...
}
Greeting.defaultProps = {
name: 'Mary'
};
props 校验
https://zh-hant.reactjs.org/docs/typechecking-with-proptypes.html
yarn add prop-types
class Greeting extends React.Component {
render() {
return (
<h1>....</h1>
);
}
}
Greeting.propTypes = {
name: PropTypes.string
};
解构的形式拿到值
<Amount
amount={this.state.amount}
onIncrement={this.onIncrement}
onDecrement={this.onDecrement}
/>
const Amount = ({ amount, onIncrement, onDecrement }) => (
<div>
<span>US Dollar: {amount} </span>
<button type="button" onClick={onIncrement}>
+
</button>
<button type="button" onClick={onDecrement}>
-
</button>
</div>
);
高阶组件
const withAmount = currencyComponents =>
class Amount extends Component {
constructor(props) {
super(props);
this.state = {
amount: 0,
};
}
onIncrement = () => {
this.setState(state => ({amount: state.amount + 1}));
};
onDecrement = () => {
this.setState(state => ({amount: state.amount - 1}));
};
render() {
return (
<div>
<span>US Dollar: {this.state.amount} </span>
<button type="button" onClick={this.onIncrement}>
+
</button>
<button type="button" onClick={this.onDecrement}>
-
</button>
{currencyComponents.map(CurrencyComponent => (
<CurrencyComponent amount={this.state.amount}/>
))}
</div>
);
}
};
const Euro = ({amount}) => <p>Euro: {amount * 0.86}</p>;
const Pound = ({amount}) => <p>Pound: {amount * 0.76}</p>;
//合并
const CurrenciesWithAmount = withAmount([Euro, Pound]);
//使用
<CurrenciesWithAmount/>
钩子
useState
const [count, setCount] = useState(0);
const [obj, setObj] = useState({sex: 10})
<button onClick={() => setCount(count + 1)}>
Click me
</button>
setObj({sex: obj.sex + 1})
useEffect
跟componentDidMount 和 componentDidUpdate 类似:
useEffect(()=>{
document.title='你好_>_'
})
useEffect(() => {
const subscription = props.source.subscribe();
return () => {
// 清除订阅,或者定时器
subscription.unsubscribe();
};
});
useEffect(
() => {
console.log(1)
},
[props.source],
);
只有当props.source 改变后才会重新创建订阅
生命周期
基本流程
constructor 创建一个组件
componentWillMout 第一次渲染之前
render 第一次渲染
componentDidMout 第一次渲染之后
修改流程:当组件的状态数据发生改变(setState)或者传递给组件的属性发生改变
shouldComponentUpdate 是否允许组件重新渲染(允许则执行后面函数,不允许直接结束即可)
componentWillUpdate 重新熏染之前
render 第二次以后重新渲染
componentDidUpdate 重新渲染之后
属性改变:
componentWillReceiveProps(nextProps/nextState):父组件把传递给组组建的属性发生改变后出发的钩子函数
接受最新属性之前,基于this.props.xxx 获取的是原有的属性信息,nextProps存储的是最新传递的属性信息
shouldComponentUpdate
是否允许组件更新, 返回true是允许,返回false 是不在继续走
componentWillUpdate:
更新之前: 和should一样,方法中通过this.state.xxx 获取的还是更新前的状态信息,方法有两个参数:nextProps/nextState存储的是最新的属性和状态
render 更新
componentDidUpdate 更新之后
卸载
componentWillUnmount :卸载组件之前(一般不用)
决定自己的高度的是你的态度,而不是你的才能
记得我们是终身初学者和学习者
总有一天我也能成为大佬
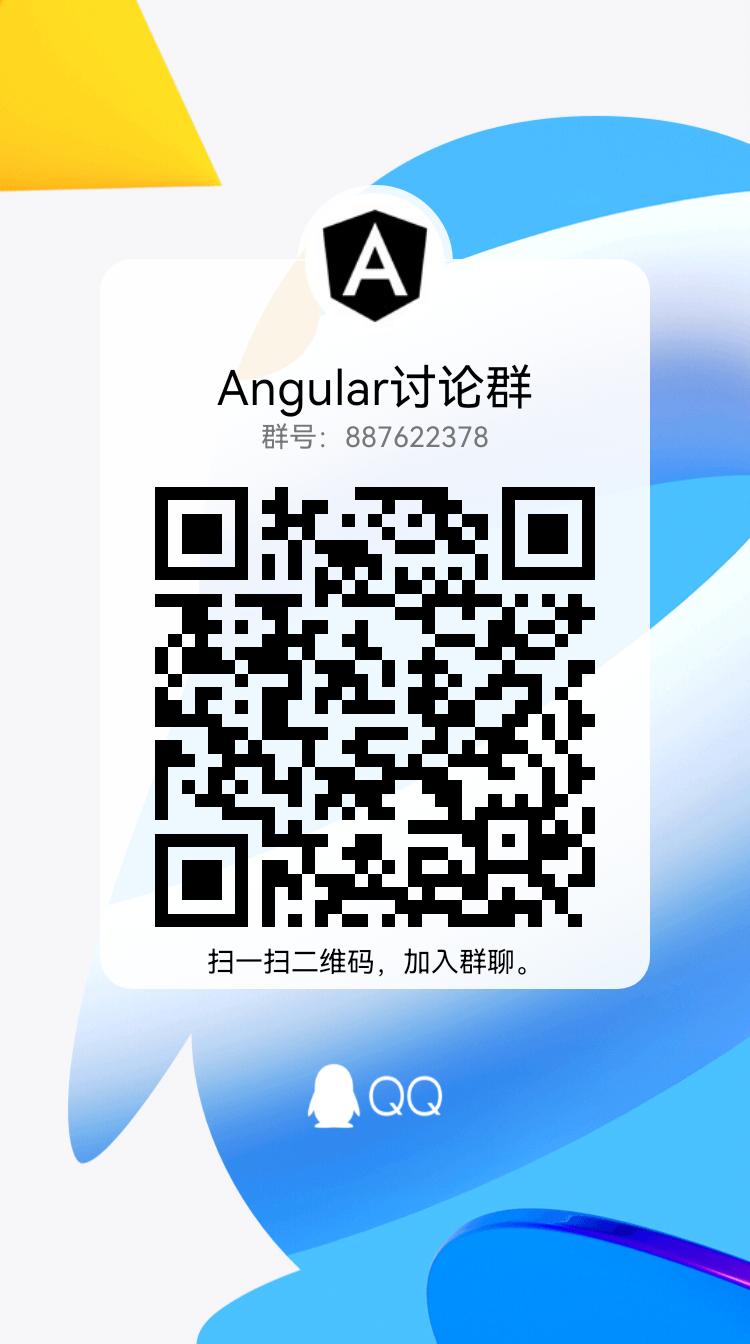