原生技巧篇
图片优化
<img src="image.jpg" loading="lazy" alt="">
lazy
延迟加载图像,直到它和视口接近到一个计算得到的距离,由浏览器定义。
可以直接在img mdn
中查看
滑动注册动态
https://dev.to/haycuoilennao19/22-sliding-form-for-website-3m8k
angular.js 想用vue,react怎么办
https://github.com/ngVue/ngVue
https://github.com/ngReact/ngReact
代码片段
* Codepen * Codesandbox * CSS-Tricks * Hakim .se * Code My UI * CreativesFeed * Bootsnipp * 30 seconds of code * 30 Seconds of CSS * Stackoverflow * Code to go * Tweetsnippet * GitHub * W3 Schools * EnjoyCSS * Web Code Tool
30s
位置分组
[ [1, 2], [2, 3], [4, 5]]
转化
[[1,2,4],[2,3,5]] 并且求和
操作
fn=(a,b,c)=>a+b+c
案例
let arr = [[1, 2], [2, 3], [4, 5]];
let fn = (a, b, c) => a + b + c
let b = Array.from(
{length: Math.max(...arr.map(v => v.length))},
(_, i) => fn(...arr.map(v => v[i])));
解析 Array.from 的妙用
Array.from(
{length: 2},
(_,i)=>arr.map(v=>v[i])
);
// [ [ 1, 2, 4 ], [ 2, 3, 5 ] ]
其实解析的结果类似
for (let i = 0; i < 2; i++) {
for (let j = 0; j < arr.length; j++) {
console.log(i);
}
}
// 0 0 0 1 1 1
fn(...[1,2,3])
等于
fn(1,2,3)
前一天
setDate() 指定一个日期对象的天数。
getDate() 查询天数
let d = new Date();
d.setDate(d.getDate()-1);
console.log(d.toISOString().split('T')[0]);
// 2021-02-16
truncateString
格式化JSON
console.log(JSON.stringify({name:'xxx',age:3},null,'\t'))
tap 事件出现的bug
touch-action: none;
// throttle 是节流事件
clickMore: throttle(function(){
this.stop = !this.stop;
},1000)
ios fixed兼容性问题
html,body{
scroll-behavior: smooth;
margin:0;
padding:0;
width: 100%;
height: 100%;
overflow: scroll;
}
arguments
剩余参数、默认参数和解构赋值参数的存在不会改变 arguments
对象的行为
function func(a = 55) {
a = 99; // updating a does not also update arguments[0]
console.log(arguments[0]);
}
func(10); // 10
自执行函数
// var a = 1;
(function a () {
a = 2;
console.log(a);
})();
// 只执行函数,如果函数名和全局变量冲突,应该打印自身函数
function add(){
add=10
console.log(add);
}
add()
//我们发现这种情况打印的是10
函数表达式
var funcName = function abc() {};
console.log(funcName); // 打印函数体function abc(){}
console.log(abc); // 报错(abc is not defined)
在这个函数表达式中,函数名称为abc,其实上,这个名称abc变成了函数内部的一个局部变量,并且指代函数对象本身,在abc函数内部打印abc的打印结果是abc函数体本身。
var funcName = function abc() {
console.log(abc); // 打印结果是function abc() {console.log(abc)}
};
匿名函数表达式顾名思义就是没有名字的函数表达式,一般情况下,我们所说函数表达式就指匿名函数表达式,因为函数表达式会忽略函数的名称,会变成匿名函数表达式,不如直接写成匿名函数表达式。
Firefox
Firefox 有更好的css 开发工具,其他的功能还是Chrome比较好
max-content
内容撑开宽度
.content{
max-width: max-content;
margin:0 auto;
background-color: khaki;
}
<div class="content">
<div class="content-item">1ddddsdssdsdd</div>
<div class="content-item">2dddd</div>
<div class="content-item">3dddd</div>
</div>
git-tip
input
.wide {
.header-menu,
.header-profile {
display: none;
}
.search-bar {
max-width: 600px;
margin: auto;
transition: 0.4s;
box-shadow: 0 0 0 1px var(--border-color);
padding-left: 0;
}
}
当获取焦点的时间我们把其他dom隐藏,然后过渡过去,失去焦点再添加,我觉得这种设计挺好的
scss
.aaa{
//.add-l 其实可以这样写,让我们省去了前缀
&-l{
}
}
选中切换的
参考
https://codepen.io/JavaScriptJunkie/pen/YzzNGeR
<template>
<div>
<div class="card">
<div class="-front">
<div class="img"></div>
<label for="one" class="cardNumber" ref="one">
<span>###################</span>
</label>
<div class="card-box">
<label for="two" class="card-box-l" ref="two" @focus="focusInput" @blur="blurInput" data-ref="two">
<span>FuLL NaMe</span>
</label>
<label for="three" class="card-box-r" ref="three" @focus="focusInput" @blur="blurInput" data-ref="three">
<span>MM/YY</span>
</label>
</div>
<div class="card-focus" :class="{'-active' : focusElementStyle }"
:style="focusElementStyle" ref="focusElement"></div>
</div>
</div>
<input type="text" id="one" @focus="focusInput" @blur="blurInput" data-ref="one">
<input type="text" id="two" @focus="focusInput" @blur="blurInput" data-ref="two">
<input type="text" id="three" @focus="focusInput" @blur="blurInput" data-ref="three">
</div>
</template>
<script>
export default {
name: "home-three",
data() {
return {
focusElementStyle: null,
//焦点
isInputFocused: false,
}
},
mounted() {
document.querySelector('#one').focus();
},
methods: {
//获取焦点
focusInput(e) {
console.log(e.target.dataset.ref);
this.isInputFocused = true;
let targetRef = e.target.dataset.ref;
let target = this.$refs[targetRef];
this.focusElementStyle = {
width: `${target.offsetWidth}px`,
height: `${target.offsetHeight}px`,
transform: `translateX(${target.offsetLeft}px) translateY(${target.offsetTop}px)`
}
},
//失去焦点
blurInput() {
let vm = this;
setTimeout(() => {
if (!vm.isInputFocused) {
vm.focusElementStyle = null;
}
}, 300);
vm.isInputFocused = false;
}
}
}
</script>
<style scoped lang="scss">
* {
box-sizing: border-box;
}
.-active {
opacity: 1 !important;
&:after {
content: "";
position: absolute;
top: 0;
left: 0;
width: 100%;
background: rgb(8, 20, 47);
height: 100%;
border-radius: 5px;
filter: blur(25px);
opacity: 0.5;
z-index: 100;
}
}
.card-focus {
position: absolute;
z-index: 3;
border-radius: 5px;
left: 0;
top: 0;
width: 100%;
height: 100%;
transition: all 0.35s cubic-bezier(0.71, 0.03, 0.56, 0.85);
opacity: 0;
pointer-events: none;
overflow: hidden;
border: 2px solid rgba(255, 255, 255, 0.65);
}
.card {
max-width: 430px;
height: 270px;
margin-left: auto;
margin-right: auto;
margin-top: 50px;
position: relative;
z-index: 2;
width: 100%;
//background-color: #000;
color: #fff;
padding: 40px;
.cardNumber {
font-weight: 500;
line-height: 1;
font-size: 27px;
margin-bottom: 35px;
display: inline-block;
padding: 10px 15px;
cursor: pointer;
span {
font-size: 28px;
}
}
.card-box {
display: flex;
justify-content: space-between;
cursor: pointer;
&-l {
color: #fff;
width: 100%;
max-width: calc(100% - 85px);
padding: 10px 15px;
font-weight: 500;
display: block;
cursor: pointer;
}
&-r {
flex-wrap: wrap;
font-size: 18px;
margin-left: auto;
padding: 10px;
display: inline-flex;
width: 80px;
white-space: nowrap;
flex-shrink: 0;
cursor: pointer;
}
}
.img {
height: 100%;
background-color: #1c1d27;
position: absolute;
left: 0;
top: 0;
width: 100%;
border-radius: 15px;
overflow: hidden;
background-image: url("https://raw.githubusercontent.com/muhammederdem/credit-card-form/master/src/assets/images/22.jpeg");
object-fit: cover;
max-width: 100%;
display: block;
max-height: 100%;
z-index: -1;
}
}
</style>
播放的样式
决定自己的高度的是你的态度,而不是你的才能
记得我们是终身初学者和学习者
总有一天我也能成为大佬
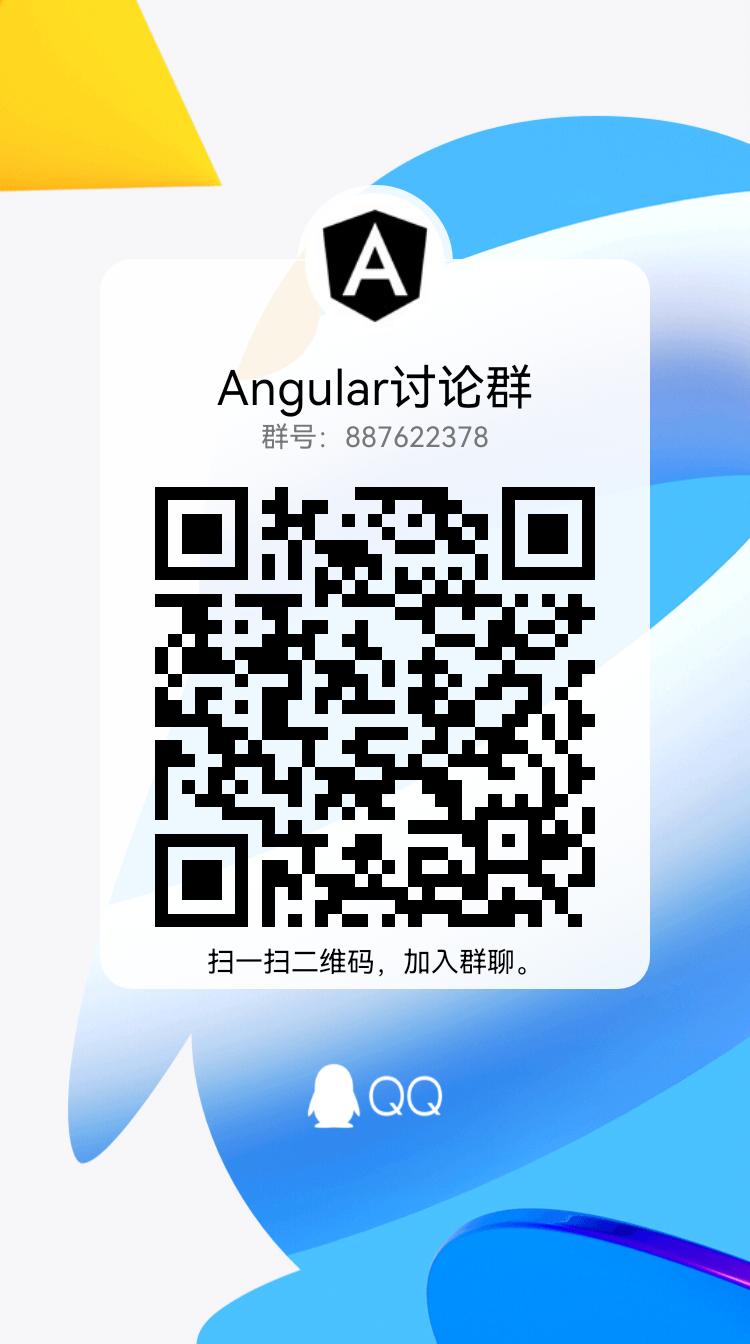