javascript原生技巧篇
鼠标悬停时加粗文本出现布局偏移
解决方案:
使用相同的文本字符添加隐藏的伪元素中,并且设置为粗体
<div class="bbb" data-text="我是谁">我是谁</div> &::after { content: attr(data-text); height: 0; visibility: hidden; overflow: hidden; font-weight:800; }
javascript 页面生命周期如何工作
HTML 页面的生命周期包含三个重要事件
DOMContentLoaded
- 浏览器已安全加载HTML,已构建DOM树,但是
img
可能尚未加载外部资源(图片和样式表)- 用途: DOM准备就绪,用于查找DOM节点,初始化接口
load
- 浏览器加载了所有资源(图像,样式)
- 用途:获取图片大小等
beforeunload/unload
- 用户离开页面时
- 用途: 清除定时器等
语法
document.addEventListener("DOMContentLoaded", ()=>{
});
// 所有资源加载完成
window.onload=function(){
}
// 离开页面的时候,
window.addEventListener('unload',function(){
return false
})
// 如果访问试图离开页面的导航或试图关闭窗口,给个提示什么的
window.onbeforeunload = function() {
return false;
};
背景图片
background: url("https://desk-fd.zol-img.com.cn/t_s960x600c5/g5/M00/0D/09/ChMkJ1f8lj-IWVrlABC5cMUqlPoAAWzfADes68AELmI907.jpg") center center/300px 300px no-repeat;
/* 背景图片 背景位置 背景大小 不重复*/
背景位置(background-position)
-
关键字(
top,right,button,left,center
)例如
top right
-
边缘偏移值
top 20px left 10px
背景大小(background-size)
- 两个值(宽 高)
- 一个值(如果仅给出一个值,则第二个将被认为是自动的)
- 可以使用百分比
background-size:conver;
平铺塞满整个空间,多的部分被裁剪
设置多个背景
background: url("https://desk-fd.zol-img.com.cn/t_s960x600c5/g5/M00/0D/09/ChMkJ1f8lj-IWVrlABC5cMUqlPoAAWzfADes68AELmI907.jpg") top right/40% 40% no-repeat,url("https://desk-fd.zol-img.com.cn/t_s960x600c5/g5/M00/0D/09/ChMkJ1f8lj-IWVrlABC5cMUqlPoAAWzfADes68AELmI907.jpg") top left/40% 40% no-repeat;
具有叠加效应
后面的如果比前面的大,会遮挡到部分前面的
加上渐变效果
<div class="bbb"></div>
<div class="aaa"></div>
.aaa{
width: 500px;
height: 400px;
background:linear-gradient(rgba(0,0,0,0.2), #000), url("https://desk-fd.zol-img.com.cn/t_s960x600c5/g5/M00/0D/09/ChMkJ1f8lj-IWVrlABC5cMUqlPoAAWzfADes68AELmI907.jpg") center/cover no-repeat;
}
.bbb{
width: 500px;
height: 400px;
background:url("https://desk-fd.zol-img.com.cn/t_s960x600c5/g5/M00/0D/09/ChMkJ1f8lj-IWVrlABC5cMUqlPoAAWzfADes68AELmI907.jpg") center/cover no-repeat;
}
高级案例
有趣的东西
Promise 和async/await 区别
getDate(a=>{
getMoreData(a,b=>{
getMoreData(b,c=>{
getMoreData(c,d=>{
getMoreData(d,e=>{
console.log(e);
})
})
})
})
})
=====
getDate()
.then(a=>getMoreData(a))
.then(b=>getMoreData(b))
.then(c=>getMoreData(c))
.then(d=>getMoreData(d))
.then(e=>console.log(e))
----
(async ()=>{
const a=await getDate();
const b=await getDate(a);
const c=await getDate(b);
const d=await getDate(c);
const e=await getDate(d);
console.log(e);
})()
总结
时代的进步
看来我控制台玩的不够好
console.log('I am a %cbutton', 'color: white; background-color: red; padding: 3px;');
无限值生成器
function* add() {
let n = 0;
while (true) {
yield n++
}
}
let a = add();
console.log(a.next().value); // 0
console.log(a.next().value); // 1
console.log(a.next().value); // 2
Promise和await/async深入理解
const greet = () => new Promise((res, rej) => {
setTimeout(() => {
res('成功')
}, 2000)
});
async function myFunc() {
let a = await greet()
console.log(a);
console.log(2);
};
myFunc().then(console.log)
//两个后执行
// 成功
// 2
// undefined
首先我们知道在函数前面加上
async
就是一个promise
因为没有
return
所以返回undefined
字符串操作混淆的点
substring
输入的负值将负值变成0,哪有较小作为开始位置
var str='abcdefg';
str.substing(-1,1) =>str.substring(0,1) //a
str.substring(1,-2) =>str.substring(0,1) //a
slice
输入的负值大于字符串的长度变成0
var str='abcdefg';
str.slice(-22) =>str.substring(0) //abcdefg
substr(start开始位置,end 字符个数)
end 为负数参数变成0
'abcd'.substr(0,-1) // '' 因为字符个数是0
发现一个跟mdn类型的教程
GSAP 动画
这个要不要深入研究下
https://greensock.com/
有趣的代码
初级
let a = [1, 2, 3, 4, 5];
let b = a['map'](v => v * 2);
console.log(b);
// [ 2, 4, 6, 8, 10 ]
升级版
Promise.resolve([1,2,3]).then(res=>res['map'](v=>v*2)).then(res=>{
console.log(res);
})
[ 2, 4, 6 ]
通用版
const call=(key,...args)=>arr=>arr[key](...args)
Promise.resolve([1,2,3,4]).then(call('map',v=>v*2)).then(res=>{
console.log(res);
})
// 第二种
const map=call.bind(null,'map')
Promise.resolve([1,2,3,4]).then(map(v=>v*2)).then(res=>{
console.log(res);
})
决定自己的高度的是你的态度,而不是你的才能
记得我们是终身初学者和学习者
总有一天我也能成为大佬
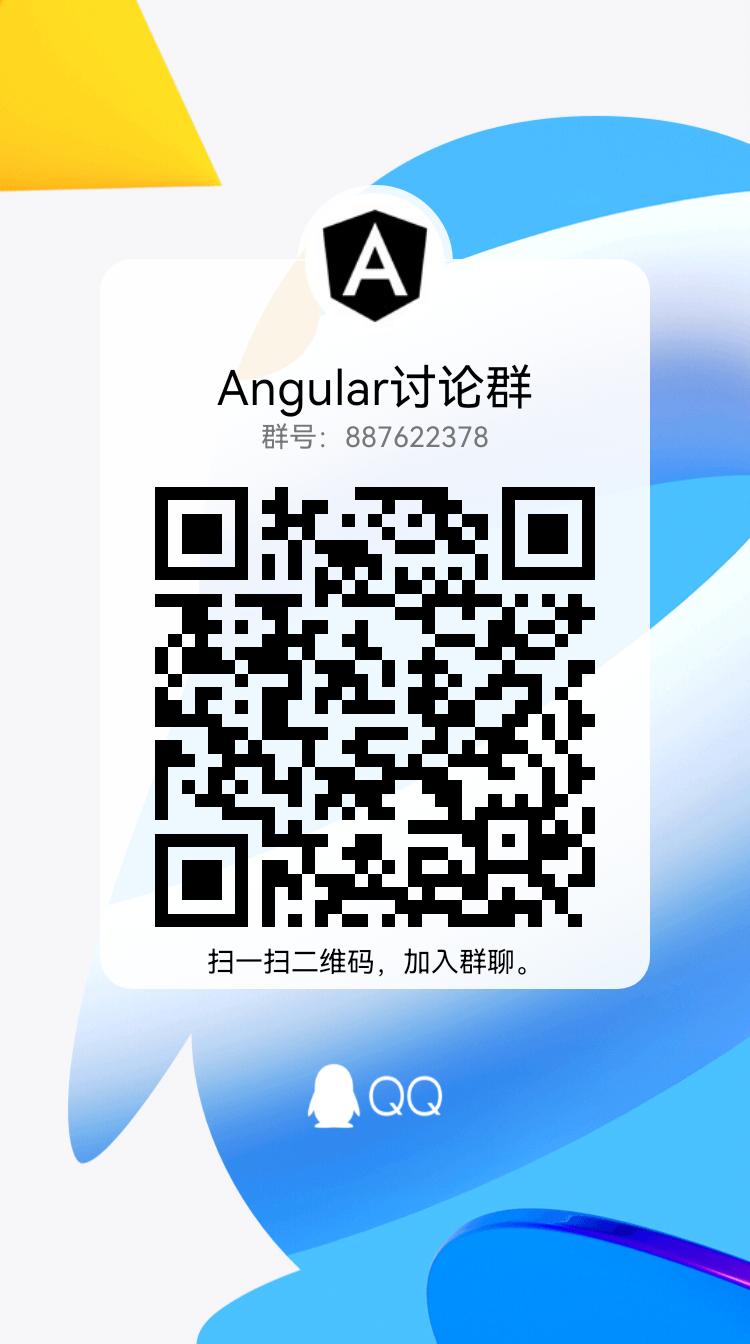