本月学习笔记
前端优化
优先使用
webp
格式图片懒加载
在页面初始化后,只会加载页面视口内的图片,当我们滚动相应的位置才会加载对应的图片
CSS练习
二分查找如何定位左边界和右边界
const binary = (arr, val) => {
let left = 0,
right = arr.length - 1
while (left <= right) {
let mid = (right + left) >> 1
if (arr[mid] === val) {
if (mid === left) {
return mid
} else {
right = mid //如果找左边界,收缩右边界
// left = mid // 如果找右边界,收缩左边界
}
} else if (arr[mid] > val) {
right = mid - 1
} else {
left = mid + 1
}
}
return -1
}
console.log(binary([1, 2, 2,2,3,4, 2, 2, 2, 3], 2))
最小公倍数
const common = (a, b) => {
let c = a > b ? a : b
while (true) {
if (c % a === 0 && c % b === 0) {
return c
}
++c
}
}
console.log(common(6,10))
最大公约数
const common = (a, b) => {
let c = a < b ? a : b
for (let i = 0; i < c + 1; i++) {
if (a % (c - i) === 0 && b % (c - i) === 0) {
return c - i
}
}
}
console.log(common(8, 12))
for ... of
获取的是属性值不是属性名
不能遍历原型上公有的属性方法(哪怕自定义的)
只能遍历可迭代的数据(Symbol.iteratoer)
关于表单的选中
checked="checked" 选中 否则 checked='' 没选中 vue 内置的 单选框 同层不要有两个v-for // 单选 <div> <label v-for="(item,index) in radios" :key="index"> <input type="radio" :value="item.id" v-model="sex">{{item.text}} </label> </div> // 多选 <div> <label v-for="(item,index) in hobby" :key="index"> <input type="checkbox" :value="item.text" :checked="item.check===true?'checked':''" @click="adds(item.check,index)">{{item.text}} </label> </div> <script> let data={ radios: [ {text: '鞋子', id: 0}, {text: '袜子', id: 1}, {text: '裤子', id: 2}, ], hobby: [ {text: 'song', check: true}, {text: 'dance', check: true}, {text: 'read', check: false}, {text: 'javascript', check: false}, ] } adds(item, index) { this.hobby[index].check = !item } </script>
vue 计算属性
刚刚在看vue部分,计算属性我们常用的是get方法,但是写在input里面就会触发set方法
比如全选非全选 <input type="checkbox" v-model="filterS"> let computed={ //全选非全选 filterS: { get() { return this.hobby.every(val => val.check === true) }, set(val) { this.hobby.forEach(item => item.check = val ? true : false) } } }
插槽
// 父组件
<test2>
<template #footer>上面</template>
<template #header>下面</template>
</test2>
// 子组件
<div>
test2
<slot name="header"></slot>
<slot name="footer"></slot>
</div>
reduce 代替 map
let arr = [1, 2, 3, 4, 5];
// console.log(arr.map(val => val * 2))
console.log(arr.reduce((acc, val) => [...acc, val*2],[]))
代替some和every
const scores = [
{ score: 45, subject: "chinese" },
{ score: 90, subject: "math" },
{ score: 60, subject: "english" }
];
// 代替some:至少一门合格
const isAtLeastOneQualified = scores.reduce((t, v) => t || v.score >= 60, false); // true
// 代替every:全部合格
const isAllQualified = scores.reduce((t, v) => t && v.score >= 60, true); // false
promise 面试题
const promise = new Promise((resolve, reject) => {
console.log(1);
console.log(2);
});
promise.then(() => {
console.log(3);
});
console.log(4);
1 2 4
如果
promise
中并没有resolve
或者reject
因此promise.then
并不会执行
const promise = new Promise((resolve, reject) => {
resolve("success1");
reject("error");
resolve("success2");
});
promise
.then(res => {
console.log("then: ", res);
}).catch(err => {
console.log("catch: ", err);
})
`then: success1`
let a = new Promise((res, rej) => {
rej('error')
res('111')
}).then(res => {
console.log(res)
}).catch(rej => {
console.log(rej)
}).then(res => {
console.log(res)
})
`error
undefined
`
构造函数中的
resolve
或reject
只有第一次执行有效,多次调用没有任何作用再次
then
没有返回值,所以打印出来undefined
Promise.resolve(1)
.then(res => {
console.log(res);
return 2;
})
.catch(err => {
return 3;
})
.then(res => {
console.log(res);
});
1 2
resolve(1)
走的第一个then
方法,并没有走catch
,所以第二个then
中res
得到的实际上是第一个then的返回值
const promise = new Promise((resolve, reject) => {
setTimeout(() => {
console.log('timer')
resolve('success')
}, 1000)
})
const start = Date.now();
promise.then(res => {
console.log(res, Date.now() - start)
})
promise.then(res => {
console.log(res, Date.now() - start)
})
`
'timer'
success 1001
success 1002
`
Promise
的.then
或者.catch
可以被调用多次,但这里Promise
构造函数只执行一次。或者说promise
内部状态一经改变,并且有了一个值,那么后续每次调用.then
或者.catch
都会直接拿到该值。
Promise.resolve(1)
.then(2)
.then(Promise.resolve(3))
.then(console.log)
1
.then
或者.catch
的参数期望是函数,传入非函数则会发生值穿透一个数字,一个对象,因此发生了穿透,所以到了最后一个
then
里
finally 一道有趣的题目
不管
Promise
对象最后的状态如何都会执行
finally()
方法的回调函数不接受任何的参数
function promise1 () {
let p = new Promise((resolve) => {
console.log('promise1');
resolve('1')
})
return p;
}
function promise2 () {
return new Promise((resolve, reject) => {
reject('error')
})
}
promise1()
.then(res => console.log(res))
.catch(err => console.log(err))
.finally(() => console.log('finally1'))
promise2()
.then(res => console.log(res))
.catch(err => console.log(err))
.finally(() => console.log('finally2'))
'promise1'
'1'
'error'
'finally1'
'finally2'
简单的说就是 等
promise1().then()
执行完才会将finally()
加入微任务队列同理把
finally()
改成then()
一样的道理
function promise1 () {
let p = new Promise((resolve) => {
console.log('promise1');
resolve('1')
})
return p;
}
function promise2 () {
return new Promise((resolve, reject) => {
reject('error')
})
}
promise1()
.then(res => console.log(res))
.catch(err => console.log(err))
.then(() => console.log('finally1'))
promise2()
.then(res => console.log(res))
.catch(err => console.log(err))
.then(() => console.log('finally2'))
promise1
1
error
finally1
finally2
Promise.resolve(1).finally(res=>{
console.log(333)
}).then(res=>{
console.log(res)
}).finally(()=>{
console.log(444)
})
333
1
444
race
它只会获取最先执行完成的那个结果,其它的异步任务虽然也会继续进行下去,不过
race
已经不管那些任务的结果了。
Promise.all()
的作用是接收一组异步任务,然后并行执行异步任务,并且在所有异步操作执行完后才执行回调
Promise.all().then()
结果中数组的顺序和Promise.all()
接收到的数组顺序一致。
function runAsync (x) {
const p = new Promise(r => setTimeout(() => r(x, console.log(x)), 1000))
return p
}
Promise.race([runAsync(1), runAsync(2), runAsync(3)])
.then(res => console.log('result: ', res))
.catch(err => console.log(err))
结果有点随机
1
'result: ' 1
2
3
async await
async function async1 () {
console.log('async1 start');
await new Promise(resolve => {
console.log('promise1')
})
console.log('async1 success');
return 'async1 end'
}
console.log('srcipt start')
async1().then(res => console.log(res))
console.log('srcipt end')
`
'script start'
'async1 start'
'promise1'
'script end'
`
在
async1
中await
后面的Promise
是没有返回值的,也就是它的状态始终是pending
状态,因此相当于没有响应所以再await之后的内容不执行,也包括
async1
后面的.then
async function async1 () {
console.log('async1 start');
await new Promise(resolve => {
console.log('promise1')
resolve('promise resolve')
})
console.log('async1 success');
return 'async1 end'
}
console.log('srcipt start')
async1().then(res => {
console.log(res)
})
new Promise(resolve => {
console.log('promise2')
setTimeout(() => {
console.log('timer')
})
})
这个只需要注意
await
后面的Promise
是有返回值的,返回没有then
是没有打印的srcipt start async1 start promise1 promise2 async1 success async1 end timer
async function async2 () {
return new Promise((resolve, reject) => {
console.log('async2')
reject('error')
})
}
async2()
async2
报错...
async函数中抛出了错误,则终止错误结果,不会继续向下执行。
this
let ,const
变量不会绑定在window
forEach、map、filter
函数的第二个参数也是能显式绑定this
的[1,2,3].map(function (val) { console.log(this) },{a:1}) { a: 1 } { a: 1 } { a: 1 }
决定自己的高度的是你的态度,而不是你的才能
记得我们是终身初学者和学习者
总有一天我也能成为大佬
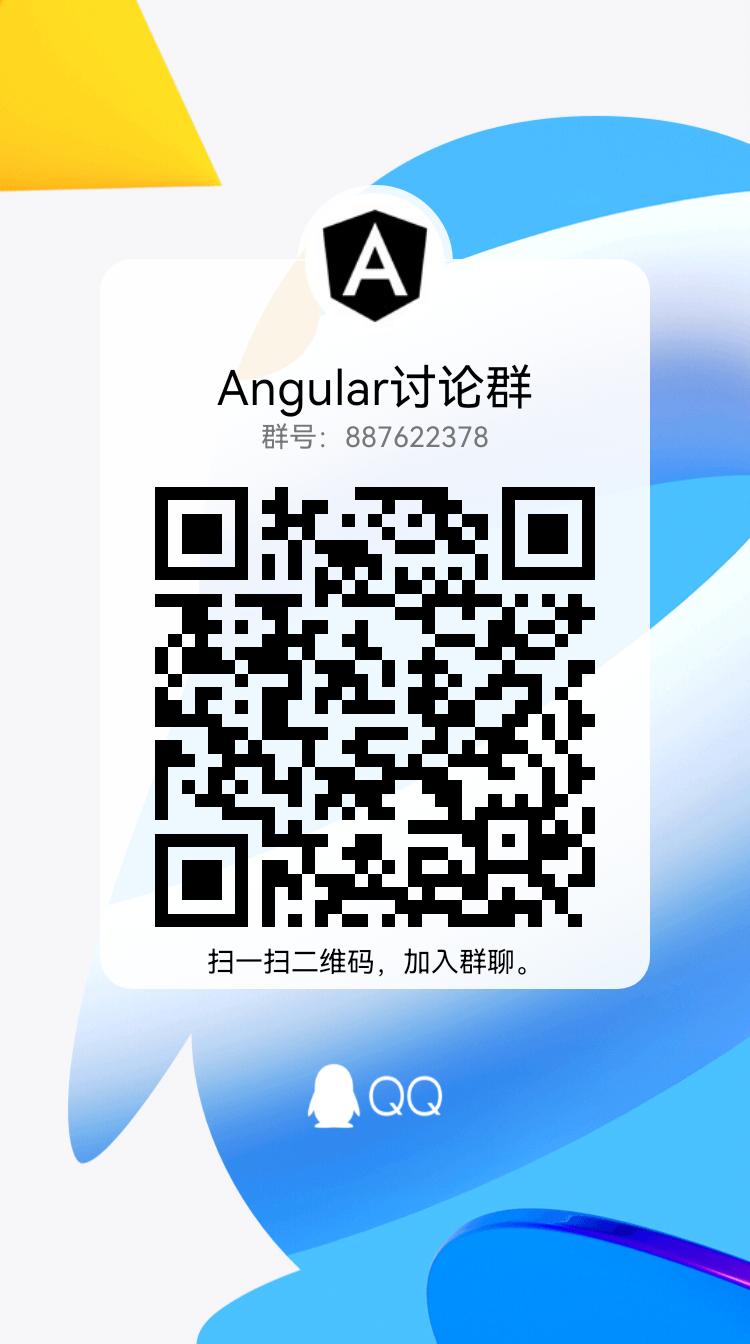