1 //µÝ¹éºÍ·ÇµÝ¹é±éÀúTree 2 // 1 3 // / \ 4 // 2 3 5 // / \ / \ 6 // 4 5 6 7 7 //Pre-Order: 1 2 4 5 3 6 7 8 //In-Order: 4 2 5 1 6 3 7 9 //Post-Order:4 5 2 6 7 3 1 10 11 #include <iostream> 12 #include <cstdio> 13 #include <stack> 14 15 using namespace std; 16 17 typedef struct node 18 { 19 int val; 20 struct node *lc; 21 struct node *rc; 22 node(int v1, struct node* _lc, struct node* _rc):val(v1),lc(_lc),rc(_rc){}; 23 }Node; 24 25 Node* createTree() 26 { 27 Node *n4 = new Node(4,NULL,NULL); 28 Node *n5 = new Node(5,NULL,NULL); 29 Node *n6 = new Node(6,NULL,NULL); 30 Node *n7 = new Node(7,NULL,NULL); 31 Node *n2 = new Node(2,n4,n5); 32 Node *n3 = new Node(3,n6,n7); 33 Node *n1 = new Node(1,n2,n3); 34 return n1; 35 } 36 37 int treeHeight; 38 void treeHigh(Node *p, int depth) 39 { 40 if(!p) 41 { 42 if(depth>treeHeight) 43 { 44 treeHeight=depth; 45 } 46 return; 47 } 48 treeHigh(p->lc,depth+1); 49 treeHigh(p->rc,depth+1); 50 } 51 52 int treeHigh1(Node *p) 53 { 54 int hl,hr; 55 if(!p) 56 { 57 return 0; 58 } 59 hl=treeHigh1(p->lc); 60 hr=treeHigh1(p->rc); 61 return (hl>hr?hl:hr)+1; 62 } 63 64 void preOrder(const Node * p) 65 { 66 if(!p) 67 { 68 return; 69 } 70 printf("%d\n",p->val); 71 preOrder(p->lc); 72 preOrder(p->rc); 73 } 74 75 void preOrder1(Node *p) 76 { 77 stack<Node*> ns; 78 79 while(!ns.empty() || p) 80 { 81 while(p) 82 { 83 printf("%d\n",p->val); 84 ns.push(p); 85 p=p->lc; 86 } 87 if(!ns.empty()) 88 { 89 p=ns.top(); 90 ns.pop(); 91 p=p->rc; 92 } 93 } 94 } 95 96 void inOrder(const Node *p) 97 { 98 if(!p) 99 { 100 return; 101 } 102 inOrder(p->lc); 103 printf("%d\n",p->val); 104 inOrder(p->rc); 105 } 106 107 void inOrder1(Node *p) 108 { 109 stack<Node*> ns; 110 111 while(!ns.empty()||p) 112 { 113 while(p) 114 { 115 ns.push(p); 116 p=p->lc; 117 } 118 if(!ns.empty()) 119 { 120 p=ns.top(); 121 printf("%d\n",p->val); 122 ns.pop(); 123 p=p->rc; 124 } 125 } 126 } 127 128 void postOrder(const Node *p) 129 { 130 if(!p) 131 { 132 return; 133 } 134 postOrder(p->lc); 135 postOrder(p->rc); 136 printf("%d\n",p->val); 137 } 138 139 void postOrder1(Node *p) 140 { 141 stack<Node *> ns; 142 bool tag[8] = {false}; 143 144 while(!ns.empty() || p) 145 { 146 while(p) 147 { 148 ns.push(p); 149 p=p->lc; 150 } 151 while(!ns.empty()&&tag[ns.top()->val]==true) 152 { 153 p=ns.top(); 154 printf("%d\n",p->val); 155 ns.pop(); 156 } 157 if(!ns.empty()) 158 { 159 p=ns.top(); 160 tag[p->val]=true; 161 p=p->rc; 162 } 163 else 164 { 165 p=NULL; 166 } 167 } 168 } 169 170 int main() 171 { 172 Node *root; 173 174 root=createTree(); 175 176 printf("preOrder Travel:\n"); 177 preOrder(root); 178 printf("\n\n"); 179 180 printf("InOrder Travel:\n"); 181 inOrder(root); 182 printf("\n\n"); 183 184 printf("PostOrder Travel:\n"); 185 postOrder(root); 186 printf("\n\n"); 187 188 //with stack, non-recursive travel 189 printf("preOrder1 Travel:\n"); 190 preOrder1(root); 191 printf("\n\n"); 192 193 printf("InOrder1 Travel:\n"); 194 inOrder1(root); 195 printf("\n\n"); 196 197 printf("PostOrder1 Travel:\n"); 198 postOrder1(root); 199 printf("\n\n"); 200 201 treeHeight=0; 202 treeHigh(root,0); 203 printf("treeHeight:%d\n",treeHeight); 204 printf("treeHeight1:%d\n",treeHigh1(root)); 205 206 return 0; 207 }
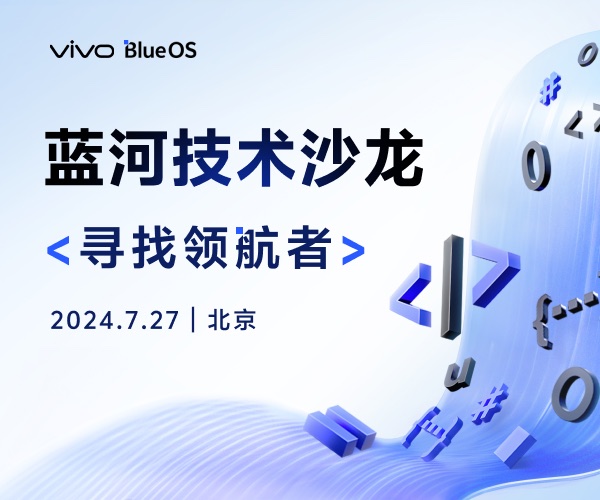