answer = sum(marbles, SIZE);
printf("The totla number of marbles is %ld.\n",answer);
printf("The size of marbles is %zd bytes.\n",sizeof marbles);
return 0;
}
int sum(int ar[], int n)
{
int i;
int total = 0;
for(i = 0; i < n; i++)
total += ar[i];
printf("The size of ar is %u bytes.\n", sizeof ar);
return total;
}
||=== Build file: "no target" in "no project" (compiler: unknown) =|
C:\Users*\Desktop\1231.c||In function 'sum'😐
C:\Users*\Desktop\1231.c|22|warning: 'sizeof' on array function parameter 'ar' will return size of 'int *' [-Wsizeof-array-argument]|
C:\Users***\Desktop\1231.c|15|note: declared here|
||= Build finished: 0 error(s), 1 warning(s) (0 minute(s), 1 second(s)) ===|

#Bx_Pointer formal parameter
<font size = 9 color =#32CD32>C ensures that when space is allocated to an array, a pointer to the first
<br> position after the array is still a valid pointer.</font>
<center>
<font align=center color=gold size = 7>Reasonable "crossing the line"</font>
</center>
include <stdio.h>
define SIZE 10
int sump(int * start, int * end);
int main(void)
{
int marbles[SIZE] = { 20, 10, 5, 39, 4, 16, 19, 26, 31, 20 };
long answer;
int en = marbles;
// printf("%d\n",(en + SIZE - 1)); // 20
answer = sump(marbles,marbles+SIZE);
printf("The total number of marbles is %ld.\n", answer);
return 0;
}
int sump(int * start, int * end)
{
int total = 0;
int i=0;
while (start < end)
{
int a;
a = * (end -1);
// total = total + *start;
// start++;
// //// update /////
total += *start++;
++i;
printf("%d.Bitter:%ld\n",i,total);
}
return total;
}
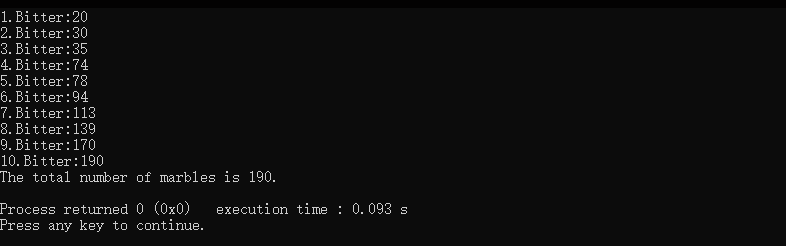
#Cx_Pointer priority
<font size = 12 color= #00FF7F >
<i>“*”</i> has the same priority as <i>‘++’</i>, but starts from right to left
</font>
include<stdio.h>
int data[2] = { 100, 200};
int moredata[2] = {300, 400};
int main(void)
{
int * p1, p2 ,p3;
p1 = p2 = data; // 100
p3 = moredata; // 300
printf(" *p1 = %d, *p2 = %d, *p3 = %d\n",*p1, *p2, *p3);
printf(" *p1++ = %d, *++p2 = %d,(*p3)++ = %d (*p3)++ = %d\n",*p1++, *++p2, *(p3++), (*p3)++);
printf(" *p1 = %d, *p2 = %d, *p3 = %d\n",*p1, *p2, *p3);
return 0;
}
---
