线性表(概念):零个或多个具有相同类型的数据元素的有限序列
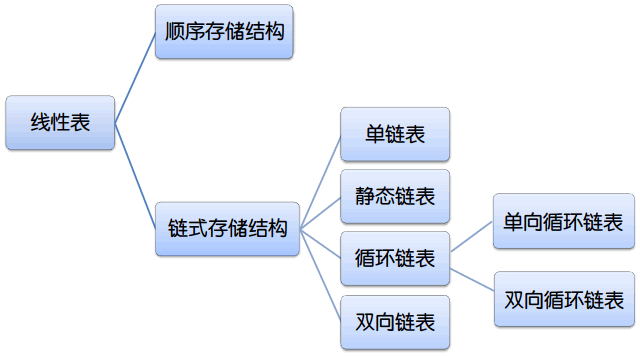
线性表的顺序存储结构:指的是用一段地址连续的存储单元依次存储线性表的数据元素。
通俗描述:在内存找了块地儿,把一定的内存空间给占了,然后把相同数据类型的数据元素依次存放在这块空地中。
描述顺序存储结构需要三个属性:
◆ 存储空间的起始位置:数组data,它的存储位置就是存储空间的存储位置
◆ 线性表的最大存储容量:数组长度MaxSize
◆ 线性表的当前长度:length
数组长度:存放线性表存储空间的长度,存储分配后这个量一般是不变的。
线性表长度:线性表中数据元素的个数,随着线性表插入和删除操作进行的,这个量是变化的。
002 | public class SequenceList<T> : IEnumerable<T> |
009 | private int elementCount; |
013 | get { return maxSize; } |
014 | set { maxSize = value; } |
019 | get { return this .tItems[i]; } |
022 | public int ElementCount |
024 | get { return this .elementCount; } |
025 | set { elementCount = value; } |
031 | /// <param name="size">线性表容量 |
032 | public SequenceList( int size) |
039 | this .tItems = new T[size]; |
041 | this .ElementCount = -1; |
045 | Console.WriteLine( "This Linear List Is Not Inital!" ); |
052 | /// <returns>true,超出容量</returns> |
055 | return this .ElementCount + 1 == this .MaxSize; |
061 | /// <returns>返回顺序线性表长度</returns> |
062 | public int ListCount() |
064 | return this .ElementCount + 1; |
069 | /// <param name="item">添加的项 |
070 | public void ListAdd(T item) |
074 | Console.WriteLine( "This Linear List Is Full,Can't Add Any Items!" ); |
079 | this .tItems[++ this .elementCount] = item; |
085 | /// <returns>true,线性表为空</returns> |
086 | public bool ListEmpty() |
088 | return this .elementCount == -1; |
093 | /// <param name="i">线性表位置 |
094 | /// <returns>返回线性表元素</returns> |
095 | public T GetElement( int i) |
097 | if ( this .elementCount == -1) |
099 | Console.WriteLine( "There Are No Elements In This Linear List!" ); |
102 | if (i < 0 || i > this .elementCount) |
104 | Console.WriteLine( "Exceed the capability!" ); |
107 | return this .tItems[i]; |
112 | /// <param name="item">线性表元素 |
113 | /// <returns>返回线性表位置</returns> |
114 | public int LocateElement(T item) |
116 | if ( this .ListCount() == 0) |
118 | Console.WriteLine( "There Are No Elements In This Linear List!" ); |
121 | for ( int i = 0; i < this .ListCount(); i++) |
124 | if ( this .tItems[i].Equals(item)) |
129 | Console.WriteLine( "Location Not Exist!" ); |
135 | public void ListClear() |
137 | this .elementCount = -1; |
141 | public IEnumerator<T> GetEnumerator() |
143 | for ( int elemIndex = 0; elemIndex < this .ListCount(); elemIndex++) |
145 | yield return this .tItems[elemIndex]; |
148 | IEnumerator System.Collections.IEnumerable.GetEnumerator() |
150 | return GetEnumerator(); |
顺序存储结构插入算法分析:
◆ 线性表是否已满、插入位置是否合理
◆ 从最后一个元素开始前向遍历到第 i 个元素位置,分别将它们都向后移动一个位置
◆ 将要插入元素填入位置 i 处
◆ 表长加1
///
/// 在指定元素后面插入元素
///
/// 线性表元素
/// 插入的元素
public void ListInsertAfter(T item, T newItem)
{
if (IsFull())
{
Console.WriteLine("This Linear List Is Full, Can't Insert Any New Items!");
return;
}
//获取线性表指定元素的位置
int location = LocateElement(item);
//插入的位置不在表尾
if (location < this.elementCount && location > 0)
{
//将指定元素后面所有元素向后移动一个位置
for (int i = this.elementCount; i >= location; i--)
{
this.tItems[i + 1] = this.tItems[i];
}
}
//插入元素
this.tItems[location] = newItem;
//表长增加1
this.elementCount++;
}
///
/// 在指定元素前面插入元素
///
/// 线性表元素
/// 插入的元素
public void ListInsertBefore(T item, T newItem)
{
if (IsFull())
{
Console.WriteLine("This Linear List Is Full, Can't Insert Any New Items!");
return;
}
//获取线性表指定元素的位置
int location = LocateElement(item);
//插入的位置不在表尾
if (location > 0)
{
ListInsert(newItem, location);
}
}
///
/// 插入操作
///
/// 插入的项
/// 插入的位置,从 1 开始
public void ListInsert(T item, int i)
{
// i 不在插入范围
if (i < 1 || i > this.elementCount + 1)
{
Console.WriteLine("This Inserting Location Is Wrong!");
return;
}
//顺序线性表已满
if (this.IsFull())
{
Console.WriteLine("This Linear List Is Full, Can't Insert Any New Items!");
return;
}
//插入的元素不在表尾
if (i <= this.elementCount)
{
//从最后一个元素向前遍历到第i个元素,分别将它们向后移动一个位置
for (int j = this.elementCount; j >= i - 1; j--)
{
this.tItems[j + 1] = this.tItems[j];
}
}
//将要插入的元素填入位置i处
tItems[i - 1] = item;
//表长加1
this.elementCount++;
}
顺序存储结构删除算法分析:
◆ 删除位置是否合理
◆ 从删除元素位置开始遍历到最后一个元素位置,分别将它们向将移动一个位置
◆ 取出删除元素
◆ 表长减1
///
/// 删除操作
///
/// 线性表位置,从 1 开始
/// 返回删除的项
public T ListDelete(int i)
{
if (this.elementCount == -1)
{
Console.WriteLine("There Are No Elements In This Linear List!");
return default(T);
}
if (i < 0 || i > this.elementCount)
{
Console.WriteLine("Delete Location Is Wrong!");
return default(T);
}
//删除的元素不在表尾
if (i < this.elementCount)
{
//从删除元素位置开始遍历到最后一个元素位置,分别将它们都向前移动一个位置
for (int j = i; j < this.elementCount; j++)
{
this.tItems[j] = this.tItems[j + 1];
}
}
//表长减1
this.elementCount--;
return this.tItems[i - 1];
}