Springmvc框架--使用自定义编辑器来实现日期格式的转换
需求:使用自定义编辑器的方式来实现日期格式的转换
添加一个BaseController.java
package cn.smbms.controller; import java.sql.Date; import java.text.SimpleDateFormat; import org.springframework.beans.propertyeditors.CustomDateEditor; import org.springframework.web.bind.WebDataBinder; import org.springframework.web.bind.annotation.InitBinder; public class BaseController { @InitBinder public void initBinder(WebDataBinder dataBinder){ System.out.println("initBinder=================="); dataBinder.registerCustomEditor(Date.class, new CustomDateEditor(new SimpleDateFormat("yyyy-MM-dd"), true)); } }
让UserController.java继承BaseController
1 package cn.smbms.controller;
2
3 import java.io.File;
4 import java.util.Date;
5 import java.util.HashMap;
6 import java.util.List;
7
8 import javax.annotation.Resource;
9 import javax.servlet.http.HttpServletRequest;
10 import javax.servlet.http.HttpSession;
11 import javax.validation.Valid;
12
13 import org.apache.commons.io.FilenameUtils;
14 import org.apache.commons.lang.math.RandomUtils;
15 import org.apache.log4j.Logger;
16 import org.springframework.stereotype.Controller;
17 import org.springframework.ui.Model;
18 import org.springframework.validation.BindingResult;
19 import org.springframework.web.bind.annotation.ModelAttribute;
20 import org.springframework.web.bind.annotation.PathVariable;
21 import org.springframework.web.bind.annotation.RequestMapping;
22 import org.springframework.web.bind.annotation.RequestMethod;
23 import org.springframework.web.bind.annotation.RequestParam;
24 import org.springframework.web.bind.annotation.ResponseBody;
25 import org.springframework.web.multipart.MultipartFile;
26
27 import com.alibaba.fastjson.JSON;
28 import com.alibaba.fastjson.JSONArray;
29 import com.mysql.jdbc.StringUtils;
30
31 import cn.smbms.pojo.Role;
32 import cn.smbms.pojo.User;
33 import cn.smbms.service.role.RoleService;
34 import cn.smbms.service.user.UserService;
35 import cn.smbms.tools.Constants;
36 import cn.smbms.tools.PageSupport;
37
38 @Controller
39 @RequestMapping("/user")
40 public class UserController extends BaseController{
41 private Logger logger = Logger.getLogger(UserController.class);
42
43 @Resource
44 private UserService userService;
45 @Resource
46 private RoleService roleService;
47
48 @RequestMapping(value = "/login.html")
49 public String login() {
50 logger.debug("UserController welcome SMBMS==================");
51 return "login";
52 }
53
54 @RequestMapping(value = "/dologin.html", method = RequestMethod.POST)
55 public String doLogin(@RequestParam String userCode,
56 @RequestParam String userPassword, HttpServletRequest request,
57 HttpSession session) {
58 logger.debug("doLogin====================================");
59 // 调用service方法,进行用户匹配
60 User user = userService.login(userCode, userPassword);
61 if (null != user) {// 登录成功
62 // 放入session
63 session.setAttribute(Constants.USER_SESSION, user);
64 // 页面跳转(frame.jsp)
65 return "redirect:/user/main.html";
66 // response.sendRedirect("jsp/frame.jsp");
67 } else {
68 // 页面跳转(login.jsp)带出提示信息--转发
69 request.setAttribute("error", "用户名或密码不正确");
70 return "login";
71 }
72 }
73
74 @RequestMapping(value = "/main.html")
75 public String main(HttpSession session) {
76 if (session.getAttribute(Constants.USER_SESSION) == null) {
77 return "redirect:/user/login.html";
78 }
79 return "frame";
80 }
81
82 @RequestMapping(value = "/logout.html")
83 public String logout(HttpSession session) {
84 // 清除session
85 session.removeAttribute(Constants.USER_SESSION);
86 return "login";
87 }
88
89 @RequestMapping(value = "/exlogin.html", method = RequestMethod.GET)
90 public String exLogin(@RequestParam String userCode,
91 @RequestParam String userPassword) {
92 logger.debug("exLogin====================================");
93 // 调用service方法,进行用户匹配
94 User user = userService.login(userCode, userPassword);
95 if (null == user) {// 登录失败
96 throw new RuntimeException("用户名或者密码不正确!");
97 }
98 return "redirect:/user/main.html";
99 }
100
101 /*
102 * @ExceptionHandler(value={RuntimeException.class}) public String
103 * handlerException(RuntimeException e,HttpServletRequest req){
104 * req.setAttribute("e", e); return "error"; }
105 */
106
107 @RequestMapping(value = "/userlist.html")
108 public String getUserList(
109 Model model,
110 @RequestParam(value = "queryname", required = false) String queryUserName,
111 @RequestParam(value = "queryUserRole", required = false) String queryUserRole,
112 @RequestParam(value = "pageIndex", required = false) String pageIndex) {
113 logger.info("getUserList ---- > queryUserName: " + queryUserName);
114 logger.info("getUserList ---- > queryUserRole: " + queryUserRole);
115 logger.info("getUserList ---- > pageIndex: " + pageIndex);
116 int _queryUserRole = 0;
117 List<User> userList = null;
118 // 设置页面容量
119 int pageSize = Constants.pageSize;
120 // 当前页码
121 int currentPageNo = 1;
122
123 if (queryUserName == null) {
124 queryUserName = "";
125 }
126 if (queryUserRole != null && !queryUserRole.equals("")) {
127 _queryUserRole = Integer.parseInt(queryUserRole);
128 }
129
130 if (pageIndex != null) {
131 try {
132 currentPageNo = Integer.valueOf(pageIndex);
133 } catch (NumberFormatException e) {
134 return "redirect:/user/syserror.html";
135 // response.sendRedirect("syserror.jsp");
136 }
137 }
138 // 总数量(表)
139 int totalCount = userService
140 .getUserCount(queryUserName, _queryUserRole);
141 // 总页数
142 PageSupport pages = new PageSupport();
143 pages.setCurrentPageNo(currentPageNo);
144 pages.setPageSize(pageSize);
145 pages.setTotalCount(totalCount);
146 int totalPageCount = pages.getTotalPageCount();
147 // 控制首页和尾页
148 if (currentPageNo < 1) {
149 currentPageNo = 1;
150 } else if (currentPageNo > totalPageCount) {
151 currentPageNo = totalPageCount;
152 }
153 userList = userService.getUserList(queryUserName, _queryUserRole,
154 currentPageNo, pageSize);
155 model.addAttribute("userList", userList);
156 List<Role> roleList = null;
157 roleList = roleService.getRoleList();
158 model.addAttribute("roleList", roleList);
159 model.addAttribute("queryUserName", queryUserName);
160 model.addAttribute("queryUserRole", queryUserRole);
161 model.addAttribute("totalPageCount", totalPageCount);
162 model.addAttribute("totalCount", totalCount);
163 model.addAttribute("currentPageNo", currentPageNo);
164 return "userlist";
165 }
166
167 @RequestMapping(value = "/syserror.html")
168 public String sysError() {
169 return "syserror";
170 }
171
172 @RequestMapping(value = "/useradd.html", method = RequestMethod.GET)
173 public String addUser(@ModelAttribute("user") User user) {
174 return "useradd";
175 }
176
177 /*
178 * @RequestMapping(value="/useradd.html",method=RequestMethod.GET) public
179 * String addUser(User user,Model model){ model.addAttribute("user", user);
180 * return "useradd"; }
181 */
182 // 其中request对象主要用来存放错误信息,便于前台获取进行相应的提示,现在是多文件上传
183 @RequestMapping(value = "/useraddsave.html", method = RequestMethod.POST)
184 public String addUserSave(
185 User user,
186 HttpSession session,
187 HttpServletRequest request,
188 @RequestParam(value = "attachs", required = false) MultipartFile[] attachs) {
189 // 编写文件上传的代码
190 // 1.判断上传的文件是否为空
191 String idPicPath = null;
192 String workPicPath = null;
193 String errorInfo = null;// 定义统一的错误信息提示
194 boolean flag = true;
195 String path = request.getSession().getServletContext()
196 .getRealPath("statics" + File.separator + "uploadfiles");
197 logger.info("uploadFile path ============== > " + path);
198
199 // 现在传过来的是多文件,使用的是数组,要进行循环遍历
200 for (int i = 0; i < attachs.length; i++) {
201 MultipartFile attach = attachs[i];
202 // 如果上传的文件不为空 与系统有关的默认名称分隔符。此字段被初始化为包含系统属性 file.separator 的值的第一个字符。在
203 // UNIX 系统上,此字段的值为 '/';在 Microsoft Windows 系统上,它为 '\\'。
204 if (i == 0) {
205 errorInfo = "uploadFileError";
206 } else if (i == 1) {
207 errorInfo = "uploadwpFileError";
208 }
209
210 String oldFileName = attach.getOriginalFilename();// 原来你存在电脑盘符的文件名
211 logger.info("原来的文件名 ============== > " + oldFileName);
212 String prefix = FilenameUtils.getExtension(oldFileName);
213 logger.info("上传文件的后缀:" + prefix);
214 int filesize = 500000;// 表示文件大小是500k
215 if (!attach.isEmpty()) {
216 if (attach.getSize() > filesize) {
217 request.setAttribute("errorInfo", "上传的文件大小不得超过500k");
218 flag = false;
219 // 判断文件的上传文件的格式
220 } else if (prefix.equalsIgnoreCase("jpg")
221 || prefix.equalsIgnoreCase("png")
222 || prefix.equalsIgnoreCase("pneg")) {
223 String fileName = System.currentTimeMillis()
224 + RandomUtils.nextInt(10000000) + "Personal.jpg";
225 logger.debug("new fileName======== " + attach.getName());
226 File targetFile = new File(path, fileName);
227 logger.info("上传到服务器的文件名是:" + targetFile.toString());
228 // 如果服务器的文件路径存在的话,就进行创建
229 if (!targetFile.exists()) {
230 /*
231 * mkdirs()可以建立多级文件夹, mkdir()只会建立一级的文件夹, 如下:
232 * new File("/tmp/one/two/three").mkdirs();
233 * 执行后, 会建立tmp/one/two/three四级目录
234 * new File("/tmp/one/two/three").mkdir();
235 * 则不会建立任何目录, 因为找不到/tmp/one/two目录, 结果返回false
236 */
237 targetFile.mkdirs();
238 }
239 try {
240 attach.transferTo(targetFile);
241 } catch (Exception e) {
242 // TODO Auto-generated catch block
243 e.printStackTrace();
244 request.setAttribute("errorInfo", "上传文件失败");
245 flag = false;
246 }
247 // 文件路径
248 if (i == 0) {
249 idPicPath = path + File.separator + fileName;
250 } else if (i == 1) {
251 workPicPath = path + File.separator + fileName;
252 }
253 logger.debug("idPicPath: " + idPicPath);
254 logger.debug("workPicPath: " + workPicPath);
255 } else {
256 request.setAttribute("errorInfo", " * 上传图片格式不正确");
257 flag = false;
258 }
259 }
260 }
261
262 if (flag) {
263 user.setCreatedBy(((User) session
264 .getAttribute(Constants.USER_SESSION)).getId());
265 user.setCreationDate(new Date());
266 user.setIdPicPath(idPicPath);
267 user.setWorkPicPath(workPicPath);
268 if (userService.add(user)) {
269 return "redirect:/user/userlist.html";
270 }
271 }
272 return "useradd";
273 }
274
275 // 练习
276 @RequestMapping(value = "/add.html", method = RequestMethod.GET)
277 public String add(@ModelAttribute("user") User user) {
278 return "user/useradd";
279 }
280
281 // 添加相应的保存方法
282 @RequestMapping(value = "/add.html", method = RequestMethod.POST)
283 // 添加相应的注解,调用验证框架,如果发现错误,会将错误保存在紧挨着的对象中bindingResult 或者是对应的error对象中
284 public String addSave(@Valid User user, BindingResult bindingResult,
285 HttpSession session) {
286 // 对bindingResult对象进行验证
287 if (bindingResult.hasErrors()) {
288 logger.debug("数据验证的时候发生错误");
289 return "user/useradd";
290 }
291 user.setCreatedBy(((User) session.getAttribute(Constants.USER_SESSION))
292 .getId());
293 user.setCreationDate(new Date());
294 if (userService.add(user)) {
295 return "redirect:/user/userlist.html";
296 }
297 return "user/useradd";
298 }
299
300 // 根据用户id获取用户信息,跳转到用户修改信息列表页面
301 @RequestMapping(value = "/userModify.html", method = RequestMethod.GET)
302 public String getUserById(@RequestParam String uid, Model model) {
303 User user = userService.getUserById(uid);
304 model.addAttribute("user", user);
305 return "usermodify";
306 }
307
308 // 修改用户信息,提交表单的操作
309 @RequestMapping(value = "/userModifySave.html", method = RequestMethod.POST)
310 public String modifyUserSave(User user, HttpSession httpSession) {
311 user.setModifyBy(((User) httpSession
312 .getAttribute(Constants.USER_SESSION)).getId());
313 user.setModifyDate(new Date());
314 // 判断有没有添加成功
315 if (userService.modify(user)) {
316 // 想:添加成功了之后,要返回到用户列表页面中去,并且从新刷新页面,客户端从新发起请求,使用重定向
317 return "redirect:/user/userlist.html";
318 }
319 return "usermodify";
320
321 }
322
323 // 根据用户id查询用户的详细信息
324 @RequestMapping(value = "/view/{id}", method = RequestMethod.GET)
325 public String view(@PathVariable String id, Model model) {
326 User user = userService.getUserById(id);
327 model.addAttribute("user", user);
328 return "userview";
329
330 }
331
332 //添加相应验证控制器 ResponseBody的作用就是将异步请求返回来的结果绑定到ResponseBody对象上面
333 @RequestMapping(value="ucexist.html")
334 @ResponseBody
335 public Object userCodeIsExist(@RequestParam String userCode){
336 HashMap<String, String> resultMap=new HashMap<String, String>();
337 if(StringUtils.isNullOrEmpty(userCode)){
338 resultMap.put("userCode", "exist");
339 }else{
340 User user = userService.selectUserCodeExist(userCode);
341 if(user!=null){
342 resultMap.put("userCode","exist");
343 }else{
344 resultMap.put("userCode", "noexist");
345 }
346 }
347 return JSONArray.toJSONString(resultMap);
348 }
349
350 //添加相应的控制器,实现异步请求 一开始是value="/view.html"会报406的错误
351 @RequestMapping(value="/view",method= RequestMethod.GET/*,produces={"application/json;charset=UTF-8"}*/)
352 @ResponseBody
353 public User view(@RequestParam String id){
354 User user=new User();
355 try {
356 user=userService.getUserById(id);
357
358 } catch (Exception e) {
359 // TODO: handle exception
360 e.printStackTrace();
361
362 }
363 return user;
364 }
365
366 }
修改springmvc-servlet.xml配置文件,将mvc:annotation-driven中引用的自定义的转换器去掉就可以了
1 <?xml version="1.0" encoding="UTF-8"?> 2 <beans xmlns="http://www.springframework.org/schema/beans" 3 xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" 4 xmlns:mvc="http://www.springframework.org/schema/mvc" 5 xmlns:p="http://www.springframework.org/schema/p" 6 xmlns:context="http://www.springframework.org/schema/context" 7 xsi:schemaLocation=" 8 http://www.springframework.org/schema/beans 9 http://www.springframework.org/schema/beans/spring-beans.xsd 10 http://www.springframework.org/schema/context 11 http://www.springframework.org/schema/context/spring-context.xsd 12 http://www.springframework.org/schema/mvc 13 http://www.springframework.org/schema/mvc/spring-mvc.xsd"> 14 15 <context:component-scan base-package="cn.smbms.controller"/> 16 <!-- 指定mvc:annotation-driven的默认实现,现在使用的是自己自定义的转化器 --> 17 <mvc:annotation-driven> 18 <mvc:message-converters> 19 <bean class="org.springframework.http.converter.StringHttpMessageConverter"> 20 <property name="supportedMediaTypes"> 21 <list> 22 <value>application/json;charset=UTF-8</value> 23 </list> 24 </property> 25 </bean> 26 <bean class="com.alibaba.fastjson.support.spring.FastJsonHttpMessageConverter"> 27 <property name="supportedMediaTypes"> 28 <list> 29 <value>text/html;charset=UTF-8</value> 30 <value>application/json</value> 31 </list> 32 </property> 33 <property name="features"> 34 <list> 35 <!-- Date的日期转换器 --> 36 <value>WriteDateUseDateFormat</value> 37 </list> 38 </property> 39 </bean> 40 </mvc:message-converters> 41 </mvc:annotation-driven> 42 <!--创建conversionService实例 当我们在遇到前后台日期类型转换的问题的时候,其实有mvc:annotation-driven一键式配置好了,所以conversionService实例不用也是可以的--> 43 <!-- <bean id="conversionService" class="org.springframework.context.support.ConversionServiceFactoryBean"></bean> --> 44 45 <!-- 引用自己自定义的转化器 --> 46 <bean id="myConversionService" class="org.springframework.context.support.ConversionServiceFactoryBean"> 47 <property name="converters"> 48 <list> 49 <bean class="cn.smbms.tools.StringToDateConvertor"> 50 <!-- 构造注入 --> 51 <constructor-arg type="java.lang.String" value="yyyy-MM-dd"></constructor-arg> 52 53 </bean> 54 55 56 57 </list> 58 59 60 </property> 61 </bean> 62 63 <mvc:resources mapping="/statics/**" location="/statics/" /> 64 <!-- 完成视图的对应 --> 65 <!-- 对转向页面的路径解析。prefix:前缀, suffix:后缀 --> 66 <!-- <bean class="org.springframework.web.servlet.view.InternalResourceViewResolver" > 67 <property name="prefix" value="/WEB-INF/jsp/"/> 68 <property name="suffix" value=".jsp"/> 69 </bean> --> 70 71 <!-- 配置多视图解析器:允许同样的内容数据呈现不同的view --> 72 <bean class="org.springframework.web.servlet.view.ContentNegotiatingViewResolver"> 73 <property name="favorParameter" value="true"/> 74 <property name="defaultContentType" value="text/html"/> 75 <property name="mediaTypes"> 76 <map> 77 <entry key="html" value="text/html;charset=UTF-8"/> 78 <entry key="json" value="application/json;charset=UTF-8"/> 79 <entry key="xml" value="application/xml;charset=UTF-8"/> 80 </map> 81 </property> 82 <!--配置网页的视图解析器 --> 83 <property name="viewResolvers"> 84 <list> 85 <bean class="org.springframework.web.servlet.view.InternalResourceViewResolver" > 86 <property name="prefix" value="/WEB-INF/jsp/"/> 87 <property name="suffix" value=".jsp"/> 88 </bean> 89 </list> 90 </property> 91 </bean> 92 93 <!-- 全局异常处理 --> 94 <bean class="org.springframework.web.servlet.handler.SimpleMappingExceptionResolver"> 95 <property name="exceptionMappings"> 96 <props> 97 <prop key="java.lang.RuntimeException">error</prop> 98 </props> 99 </property> 100 </bean> 101 102 <!-- 配置MultipartResolver,用于上传文件,使用spring的CommonsMultipartResolver --> 103 <bean id="multipartResolver" class="org.springframework.web.multipart.commons.CommonsMultipartResolver"> 104 <property name="maxUploadSize" value="5000000"/> 105 <property name="defaultEncoding" value="UTF-8"/> 106 </bean> 107 108 </beans>
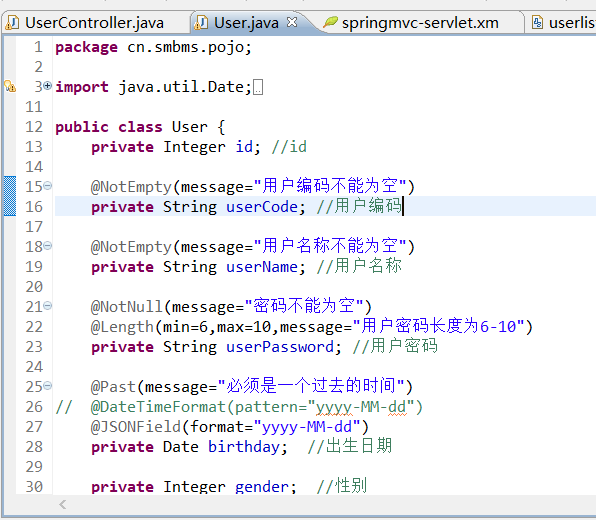
运行结果: