假装是商品列表
效果展示
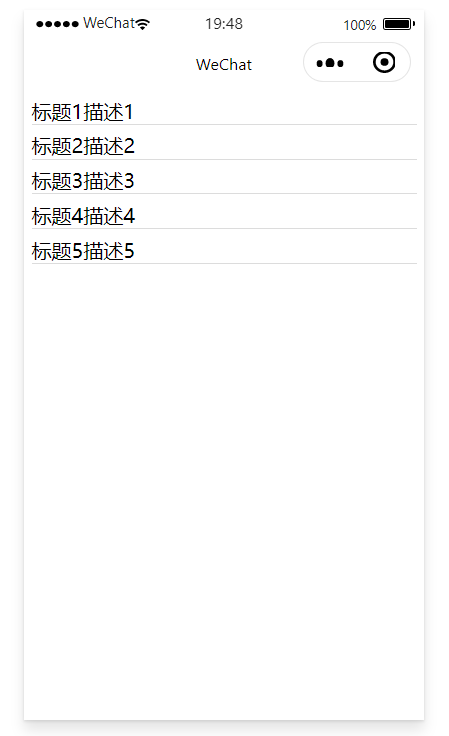
index.wxml
<block wx:for="{{dataList}}" wx:key="index">
<view class="itemRoot" bindtap="goDetail" data-item="{{item}}">
<text>{{item.title}}</text>
<text>{{item.desc}}</text>
</view>
</block>
index.js
Page({
data: {
dataList:[]
},
onLoad: function (options) {
wx.cloud.database().collection("homelist")
.get()
.then(res=>{
console.log("获取成功",res);
this.setData({
dataList:res.data
})
})
.catch(err=>{
console.log("获取失败",err);
})
},
//跳转到详情页
goDetail(event){
console.log("点击获取的数据",event.currentTarget.dataset.item._id);
wx.navigateTo({
url: '/pages/detail/detail?id='+event.currentTarget.dataset.item._id,
})
}
})
假装是商品详情
效果展示
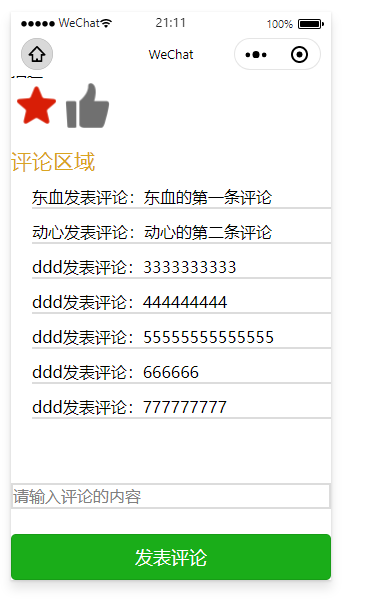
detail.wxml
点赞和收藏主要是绑定点赞和收藏的事件,评论区域获取pinglun数组里的内容,绑定输入框的内容,然后绑定发表事件
<view>
<view>{{detail.title}}</view>
<view>{{detail.desc}}</view>
</view>
<!-- 点赞和收藏 -->
<image class="image" src="{{shoucangUrl}}" bindtap="clickShouc"></image>
<image class="image" src="{{dianzanUrl}}" bindtap="clickDianzan"></image>
<!-- 评论 -->
<view class="tip">评论区域</view>
<block wx:for="{{pinglun}}" wx:key="index">
<view class="pinglunItem">
<text>{{item.name}}发表评论:</text>
<text>{{item.content}}</text>
</view>
</block>
<!-- 发表评论 -->
<input class="input" bindinput="getContent" placeholder="请输入评论的内容" value="{{content}}"></input>
<button type="primary" bindtap="fabiao">发表评论</button>
detail.wxss
.image{
width: 120rpx;
height: 120rpx;
}
.tip{
margin-top: 30rpx;
font-size: 50rpx;
color: goldenrod;
}
.pinglunItem{
border-bottom: 2px solid gainsboro;
margin-left: 50rpx;
margin-top: 30rpx;
}
.input{
border:2px solid gainsboro;
margin-top: 150rpx;
margin-bottom: 60rpx;
}
数据库展示图片
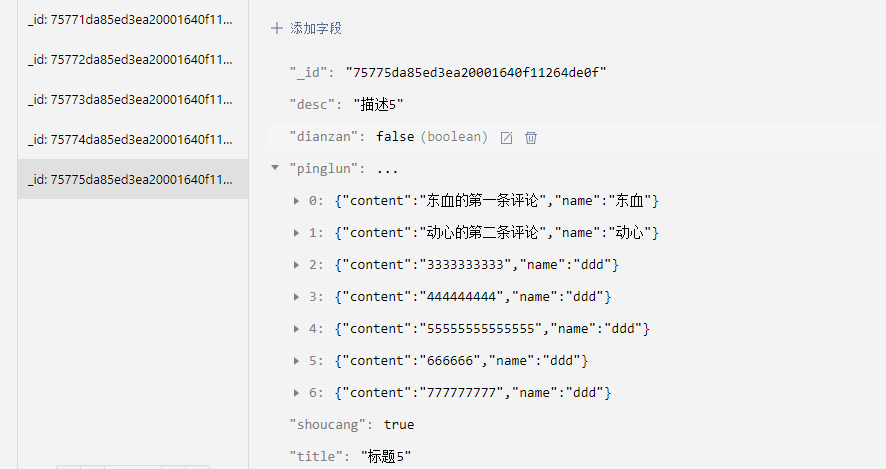
detail.js
开头onload将dianzan与shoucang添加到样式中,显示定义变量,然后刷入数据到小程序,收藏切换和点赞切换,在按钮点击之后就设置图片的显示,然后执行云函数,云函数改变云数据库里的收藏设置值。设置发表,将内容添加到原有的上。
//传送给数据库的
let ID = ''
let shoucang = false
let dianzan = false
Page({
data: {
detail: '',
shoucangUrl: "../../images/shoucang.png",
dianzanUrl: "../../images/dianzan.png",
pinglun:[], //评论数组
content:''
},
//收藏切换
clickShouc() {
//三元运算符方式
this.setData({
shoucangUrl: shoucang ? "../../images/shoucang.png" : "../../images/shoucanghave.png"
})
shoucang = !shoucang
wx.cloud.callFunction({
//调用的云函数名
name: "detailcaozuo",
data: {
action: "shoucang",
//要上传的数据,在云函数的event里
id: ID,
shoucang: shoucang
}
})
.then(res => {
console.log("改变收藏状态成功", res);
})
.catch(err => {
console.log("改变收藏状态失败", err);
})
},
//点赞切换
clickDianzan() {
this.setData({
dianzanUrl: dianzan ? "../../images/dianzan.png" : "../../images/dianzanhave.png"
})
dianzan = !dianzan
//云函数方式操作
wx.cloud.callFunction({
//调用的云函数名
name: "detailcaozuo",
data: {
action: "dianzan",
//要上传的数据,在云函数的event里
id: ID,
dianzan: dianzan
}
})
.then(res => {
console.log("改变点赞状态成功", res);
})
.catch(err => {
console.log("改变点赞状态失败", err);
})
},
onLoad(options) {
ID = options.id;
console.log("详情页接收的id", options.id);
//doc匹配,where匹配
wx.cloud.database().collection("homelist")
.doc(options.id)
.get()
.then(res => {
console.log("详情页成功", res);
//将收藏添加到数据库
shoucang = res.data.shoucang
dianzan = res.data.dianzan
console.log(shoucang, dianzan);
//再次显示数据
this.setData({
detail: res.data,
shoucangUrl: shoucang ? "../../images/shoucanghave.png" : "../../images/shoucang.png",
dianzanUrl: dianzan ? "../../images/dianzanhave.png" : "../../images/dianzan.png",
pinglun:res.data.pinglun
})
})
.catch(err => {
console.log("详情页失败", err);
})
},
//获取输入的值
getContent(event){
this.setData({
content:event.detail.value
})
},
//发表评论
fabiao(){
let content=this.data.content
if(content.length<4){
wx.showToast({
icon:"none",
title: '评论太短了',
})
return
}
let pinglunItem={}
pinglunItem.name="ddd"
pinglunItem.content=content
let pinglunArr=this.data.pinglun
pinglunArr.push(pinglunItem)
console.log("添加后的评论数组",pinglunArr)
wx.showLoading({
title: '发表中',
})
wx.cloud.callFunction({
name:"detailcaozuo",
data:{
action:"fabiao",
id: ID,
pinglun:pinglunArr
}
}).then(res=>{
console.log("发表成功",res);
this.setData({
pinglun:pinglunArr,
content:''
})
wx.hideLoading()
}).catch(err=>{
console.log("发表失败",err);
wx.hideLoading()
})
}
})
云函数detailcaozuo.js
data里:前面为数据库字段,后面为修改之后的值
// 云函数入口文件
const cloud = require('wx-server-sdk')
//小技巧可以动态改变环境
cloud.init({
env: cloud.DYNAMIC_CURRENT_ENV
})
// 云函数入口函数
exports.main = async (event, context) => {
if (event.action == 'shoucang') {
//异步操作
return await cloud.database().collection("homelist").doc(event.id)
.update({
data: {
shoucang: event.shoucang
}
})
.then(res => {
console.log("改变收藏状态", res);
return res
})
.catch(err => {
console.log("改变收藏状态失败", err);
return err
})
} else if(event.action=='dianzan'){
//异步操作
return await cloud.database().collection("homelist").doc(event.id)
.update({
data: {
dianzan: event.dianzan
}
})
.then(res => {
console.log("改变点赞状态成功", res);
return res
})
.catch(err => {
console.log("改变点赞状态失败", err);
return err
})
}else if(event.action=='fabiao'){
//异步操作
return await cloud.database().collection("homelist").doc(event.id)
.update({
data: {
// 前面为数据库字段,后面为修改之后的值
pinglun: event.pinglun
}
})
.then(res => {
console.log("添加评论成功", res);
return res
})
.catch(err => {
console.log("添加评论失败", err);
return err
})
}
}