react知识点
dva中通过connect函数注入dispatch,获取dispatch的方式有两种:
1)const {dispatch}= this.props;
2)通过全局变量:window.g_app._store.dispatch({ type: 'login/logout', });
ref总结:
1)字符串格式ref: <input ref=''input1"/> console.log(this.refs.input1.value) 2)回调函数格式ref: <input ref={c => this.input1 = c} /> console.log(this.input1.value) 3)内联函数格式ref:(会调用两次,每次更新首先会被赋值null,然后再赋值真正的ref,实现清空处理)官网说了,虽然调用两次但是无关紧要 <input ref={(c) => {this.input1=c;console.log('@',c)}}/> 4)类的绑定函数格式ref: saveInput = (c) => { this.input1=c; } <input ref={this.saveInput}/> 开发中最常用的是内联形式的ref 5)createRef格式的ref(react中最推荐的写法): myRef = React.createRef(); showData = () => { console.log(this.myRef.current.value) } <input ref={this.myRef}/> <Button onClick={this.showData}></Button>
受控组件与非受控组件:
给表单元素绑定ref属性,通过ref拿到表单元素的value值的,这种方式叫非受控组件
给表单元素绑定onChange时间,通过动态改变state的值,拿到input的value值,叫受控组件
把需要setState的属性名当变量传到方法中怎么赋值 saveData = (dataType) => { return (e) =>{ this.setState({ [dataType]:e.target.value }) } } <input onChange = {this.saveData('userName)}/>
高阶函数: 1、若A函数接受的参数是一个函数,那么A就可以称之为高阶函数 2、若A函数,调用的返回值依然是一个函数,那么A就可以称之为高阶函数 常见的高阶函数有哪些:promise (参数为一个函数),setTimeout setInterval arr.map等等 函数的柯里化:通过函数调用返回函数的方式,实现多次接收参数最后统一处理的函数编码形式
如:function sum(a){
return (b) => {
return (c) => {
return a+b+c
}
}
}
sum(1)(2)(3)
forceUpdate强制更新生命周期 调用this.forceUpdate()生命周期=》componentWillUpdate=>render=>componentDidUpdate
this.setState=>shouldComponentUpdate(如返回值为true则会往下执行,如果为false,到此结束)=》componentWillUpdate=》render=>componentDidUpdate
正常的生命周期执行流程 父组件render=>componentWillReceiveProps=>shouldComponentUpdate=>componentWillUpdate=>render=》componentDidUpdate
生命周期(旧版) 1、初始化阶段:由ReactDOM.render()触发初次渲染 1)constructor() 2)componentWillMount() 3)render() 4)componentDidMount()====一般在这里做初始化处理 2、更新阶段:由组件内部this.setState()或父组件render触发 1)shouldComponentUpdate() 2)componentWillUpdate() 3)render() 4)componentDidUpdate() 3、卸载组件:由ReactDOM.unmountComponentAtNode()触发 1)componentWillUnMount
生命周期(旧版) 1、初始化阶段:由ReactDOM.render()触发初次渲染 1)constructor() 2)componentWillMount() 3)render() 4)componentDidMount()====一般在这里做初始化处理 2、更新阶段:由组件内部this.setState()或父组件render触发 1)shouldComponentUpdate() 2)componentWillUpdate() 3)render() 4)componentDidUpdate() 3、卸载组件:由ReactDOM.unmountComponentAtNode()触发 1)componentWillUnMount
旧版本生命周期和新版本生命周期的区别:
旧版本生命周期即将废弃三个钩子 componentWillMount() componentWillUpdate() componentWillReceiveProps()
新版本生命周期增加了两个钩子 geDerivedStateFromProps() getSnapshotBeforeUpdate()
getDerivedStateFromProps用法(如果state的值什么时候都取决于props值。则用这个生命周期改变state)
static getDerivedStateFromProps(props,state){return {}}
顺序:constructor=》getDerivedStateFromProps=>render=>ComponentDidMount
getSnapshotBeforeUpdate://此生命周期可用于无缝滚动效果,在componentDidUpdate之前获取滚动列表的高度或scrollTop值,
getSnapshotBeforeUpdate(prevProps,prevState){
return snapshotValue,//此处return的值传给compnentDidUpdate生命周期
}
componentDidUpdate(prevProps,prevState,snapshot){
//此处的snapshot值为getSnapshotBeforeUpdate中返回的snapshotValue
}
新生命周期的总结 1、初始化阶段:由ReactDOM.render触发初次渲染 1)constructor() 2)getDerivedStateFromProps() 3)render() 4)componentDidMount() 2、更新阶段:由组件内部setState()或父组件重新render触发 1)getDerivedStateFromProps() 2)shouldComponentUpdate() 3)render() 4)getSnapshotBeforeUpdate() 5)comonentDidUpdate() 3、卸载组件:由React DOM.unmountComponentAtNode()触发 1)componentWillUnMount()
Key: 1、虚拟DOM中key的作用:当状态中数据发生变化时,react会根据新数据生成新的虚拟DOM,随后REACT进行新的虚拟DOM和旧的虚拟DOM进行diff比较,比较规则如下: a.旧虚拟DOM与新虚拟DOM中相同的key: 1)若虚拟DOM中内容没变,直接使用之前的真实DOM 2)若虚拟DOM中内容变了,则生成新的真实DOM,随后替换掉页面中之前的真实DOM b.旧虚拟DOM中未找到新虚拟DOM中相同的key,根据数据创建新的真实DOM,随后渲染到页面, 2、用index作为key可能会引发的问题 1)若对数据进行逆序添加、逆序删除等破环顺序操作,会产生没有必要的真实DOM更新=》界面没问题但是效率低 2)如果结构中包含输入类的DOM,会产生错误的DOM更新,界面有问题 3)注意,如果不存在对数据的逆序添加,逆序删除等破环顺序操作,仅用于渲染列表用于展示,用index作为key也是没问题的。 3、开发中如何选择key? 1)使用每条唯一的标识作为key,如id、学号等 2)如果只是简单的渲染数据,不存在添加删除操作,用索引index作为key也没问题
配置代理:
方法1:直接在package.json中添加一行:
"proxy":"http://localhost:50000" 重启
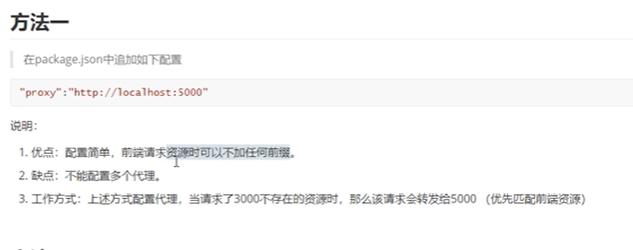
路由: let history = History.createBrowserHistory();//h5推出的History let history = History.createHashHistory();//路由后边带# history.push(path) history.replace(path) history.goback() history.goForward()前进 history.listen()