<dependencies>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter</artifactId>
</dependency>
<dependency>
<groupId>com.h2database</groupId>
<artifactId>h2</artifactId>
<scope>runtime</scope>
</dependency>
<dependency>
<groupId>com.baomidou</groupId>
<artifactId>mybatis-plus-boot-starter</artifactId>
</dependency>
<dependency>
<groupId>com.fasterxml.jackson.core</groupId>
<artifactId>jackson-databind</artifactId>
</dependency>
<dependency>
<groupId>com.alibaba</groupId>
<artifactId>fastjson</artifactId>
</dependency>
<dependency>
<groupId>com.google.code.gson</groupId>
<artifactId>gson</artifactId>
<version>2.8.6</version>
</dependency>
</dependencies>
- 数据库
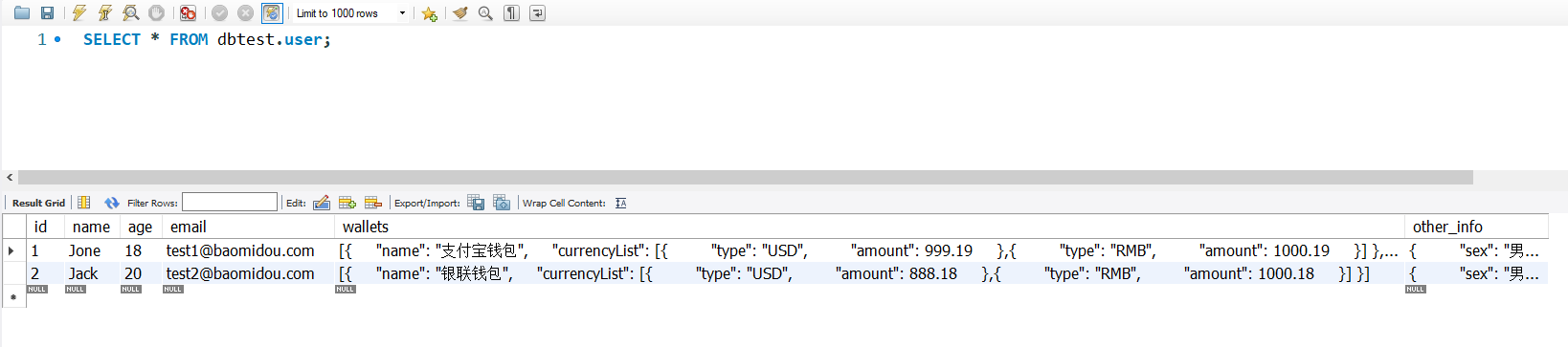
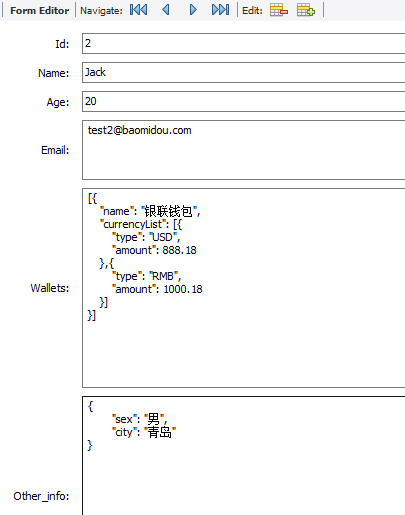
@Component
public class MpJsonConfig implements CommandLineRunner {
/**
* 可以set进去自己的
*/
@Override
public void run(String... args) throws Exception {
JacksonTypeHandler.setObjectMapper(new ObjectMapper());
GsonTypeHandler.setGson(new Gson());
}
@Bean
public MybatisPlusInterceptor mybatisPlusInterceptor() {
MybatisPlusInterceptor interceptor = new MybatisPlusInterceptor();
interceptor.addInnerInterceptor(new PaginationInnerInterceptor());
return interceptor;
}
}
@Data
@Accessors(chain = true)
@TableName(autoResultMap = true)
public class User {
private Long id;
private String name;
private Integer age;
private String email;
/**
* 注意!! 必须开启映射注解
*
* @TableName(autoResultMap = true)
* <p>
* 以下两种类型处理器,二选一 也可以同时存在
* <p>
* 注意!!选择对应的 JSON 处理器也必须存在对应依赖包
*/
@TableField(typeHandler = WalletListTypeHandler.class)
private List<Wallet> wallets;
@TableField(typeHandler = FastjsonTypeHandler.class)
private OtherInfo otherInfo;
}
public class WalletListTypeHandler extends JacksonTypeHandler {
public WalletListTypeHandler(Class<?> type) {
super(type);
}
@Override
protected Object parse(String json) {
try {
return getObjectMapper().readValue(json, new TypeReference<List<Wallet>>() {
});
} catch (IOException e) {
throw new RuntimeException(e);
}
}
}
/**
* UPDATE user SET age=?, wallets=? WHERE (id = ?)
* 99(Integer), [{"name":"Tom","currencyList":[{"type":"RMB","amount":1000.0}]}](String), 2(Long)
* SELECT id,name,age,email,wallets,other_info FROM user WHERE id=?
* 2(Integer)
*/
@Test
public void test2() {
// wrapper typeHandler 测试
LambdaUpdateWrapper<User> wrapper = Wrappers.<User>lambdaUpdate().set(User::getWallets, Arrays.asList(new Wallet("Tom",
Arrays.asList(new Currency("RMB", 1000d)))), "typeHandler=com.baomidou.mybatisplus.samples.typehandler.WalletListTypeHandler");
/**
* Arrays.asList() 将数组转为list集合
* --->[Wallet(name=Tom, currencyList=[Currency(type=RMB, amount=1000.0)])]
*/
System.out.println("--->" + Arrays.asList(new Wallet("Tom",
Arrays.asList(new Currency("RMB", 1000d)))));
wrapper.eq(User::getId, 2L);
// 更新id为2的数据
Assertions.assertEquals(userMapper.update(new User().setAge(99), wrapper), 1);
/**
* 查看是否修改成功
* User(id=2, name=Jack, age=99, email=test2@baomidou.com, wallets=[Wallet(name=Tom, currencyList=[Currency(type=RMB, amount=1000.0)])], otherInfo=OtherInfo(sex=男, city=青岛))
*/
System.err.println(userMapper.selectById(2));
}