输出流:程序(内存)--->外界设备
输⼊流:外界设备--->程序(内存)
字符流:处理字符相关,如处理⽂本数据(如txt⽂件), Reader/Writer
字节流: 处理字节相关,如声⾳或者图⽚等⼆进制,InputStream/OutputStream
字节流以字节(8bit)为单位,字符流以字符为单位,根据码表映射字符,⼀次可能读多个字节
字节流可以处理⼏乎所有⽂件,字符流只能处理字符类型的数据
字符流 Reader/Writer
字节流 InputStream/OutputStream
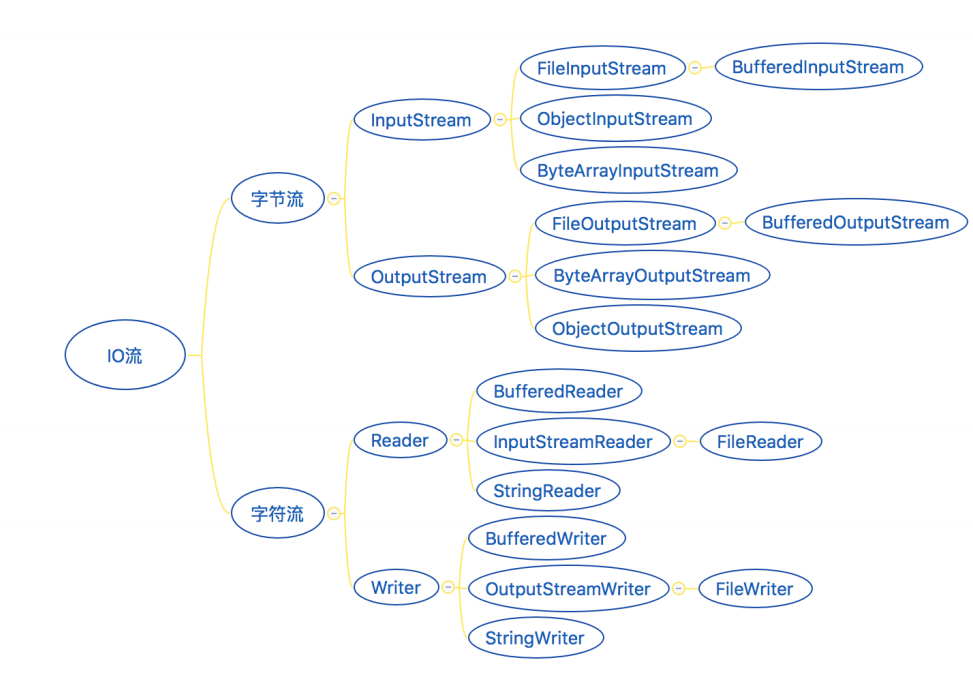
- InputStream是输⼊字节流的⽗类,它是⼀个抽象类(⼀般⽤他的⼦类)
int read()
从输⼊流中读取单个字节,返回0到255范围内的int字节值,字节数据可直接转换为int类 型, 如果已经到达流末尾⽽没有可⽤的字节,则返回-1
int read(byte[] buf)
从输⼊流中读取⼀定数量的字节,并将其存储在缓冲区数组buf中, 返回实际读取的字节数,如果已经到达流末尾⽽没有可⽤的字节,则返回-1
long skip(long n)
从输⼊流中跳过并丢弃 n 个字节的数据。
int available()
返回这个流中有多少个字节数,可以把buf数组⻓度定为这个
void close() throws IOException
关闭输⼊流并释放与该流关联的系统资源
抽象类InputStream⽤来具体实现类的创建对象, ⽂件字节输⼊流, 对⽂件数据以字节的形式进⾏读取操作
//传⼊⽂件所在地址
public FileInputStream(String name) throws FileNotFoundException
//传⼊⽂件对象
public FileInputStream(File file) throws FileNotFoundException
public class Main {
public static void main(String[] args)throws IOException {
String dir = "C:\\work";
String name = "test.txt";
File file = new File(dir,name);
InputStream inputStream = new FileInputStream(file);
testRead(inputStream);
testSkip(inputStream);
testReadByteArr(inputStream);
}
/**
* 读取所有字节
* @param inputStream
* @throws IOException
*/
public static void testReadByteArr(InputStream inputStream)throws IOException{
//如果buf的长度为0,则不读取任何字节并返回0;每次读取的字节数最多等于buf的长度
// 写法1
// byte[] buf = new byte[1024];
// 写法2
byte[] buf = new byte[inputStream.available()];
int length;
//循环读取文件内容,输入流中将最多的buf.length个字节数据读入一个buf数组中,返回类型是读取到的字节数
//如果这个缓冲区没有满的话,则返回真实的字节数
while ((length = inputStream.read(buf))!=-1){
//System.out.print(new String(buf,0,length)); // 从buf数组中读取,从0开始读取,读取到length
//中文乱码问题,换成GBK,或者UTF-8
System.out.print(new String(buf,0,length,"UTF-8"));
}
// 关闭流
inputStream.close();
}
/**
* 读取1个字节
* @param inputStream
* @throws IOException
*/
public static void testRead(InputStream inputStream)throws IOException{
//对于汉字等 unicode中的字符不能正常读取,只能以乱码的形式显示
int read = inputStream.read();
System.out.println(read);
System.out.println((char)read);
// 关闭流
inputStream.close();
}
/**
*
* @param inputStream
* @throws IOException
*/
public static void testSkip(InputStream inputStream)throws IOException{
// 跳过2个字节,读取第3个字节
long skipSize = inputStream.skip(2);
System.out.println(skipSize);
int read = inputStream.read();
System.out.println(read);
System.out.println((char)read);
// 关闭流
inputStream.close();
}
}
- OutputStream是输出字节流的⽗类,它是⼀个抽象类
void write(int b)
将指定的字节写⼊输出流
void write(byte[] b)throws IOException
将b.length个字节的byte数组写⼊当前输出流
void flush() throws IOException
write是写到缓冲区中,可以认为是内存中,当缓冲区满时系统会⾃动将缓冲区的内容写⼊⽂件,但是⼀般还有⼀部分有可能会留在内存这个缓冲区中, 所以需要调⽤flush空缓冲区数据。
void close() throws IOException
关闭输⼊流并释放与该流关联的系统资源
- 抽象类OutputStream⽤来具体实现类的创建对象, ⽂件字节输出流, 对⽂件数据以字节的形式进⾏输出的操作
//传⼊输出的⽂件地址
public FileOutputStream(String name)
//传⼊⽬标输出的⽂件对象
public FileOutputStream(File file)
//传⼊⽬标输出的⽂件对象, 是否可以追加内容
public FileOutputStream(File file, boolean append)
public class Main {
public static void main(String[] args)throws IOException {
String dir = "C:\\work";
String name = "test.txt";
String target = "b.txt";
File file = new File(dir,name); // 拼接文件的路径
InputStream inputStream = new FileInputStream(file); // 读取文件
// 写法1,写入数据,会自动创建文件,但是不会创建多级目录下的文件
// OutputStream outputStream = new FileOutputStream(dir+File.separator+target);
// 写法2,写入数据,不覆盖文件,追加数据
OutputStream outputStream = new FileOutputStream(dir+File.separator+target,true);
// 测试
testOut(inputStream,outputStream);
testOutBuf(inputStream,outputStream);
}
/**
* 将inputStream中的数据写入outputStream
* @param inputStream
* @param outputStream
* @throws IOException
*/
public static void testOutBuf(InputStream inputStream,OutputStream outputStream)throws IOException {
byte [] buf = new byte[1024];
int length ;
while (( length = inputStream.read(buf))!=-1){
outputStream.write(buf,0,length);
}
//关闭流
inputStream.close();
outputStream.close();
}
// 写入文件测试1,单个字节读取,中文会有问题
public static void testOut(InputStream inputStream,OutputStream outputStream)throws IOException{
int value = 0;
while (value!=-1){
value = inputStream.read();
outputStream.write(value);
}
//最后记得关闭流
inputStream.close();
outputStream.close();
}
}
它是内存空间的⼀部分,在内存空间中预留了⼀定的存储空间,这些存储空间⽤来缓冲输⼊或输出的数据,这部分空间就叫做缓冲区,缓冲区是具有⼀定⼤⼩的
# 缓冲,缓和冲击,例如操作磁盘⽐内存慢的很多,所以不⽤缓冲区效率很低
# 数据传输速度和数据处理的速度存在不平衡,⽐如你每秒要写100次硬盘,对系统冲击很⼤,浪费了⼤量时间在忙着处理开始写和结束写这两个事件,⽤buffer暂存起来,变成每10秒写⼀次硬盘,数据可以直接送往缓冲区,⾼速设备不⽤再等待低速设备,对系统的冲击就很⼩,写⼊效率⾼了
BufferInputStream
BufferOutputStream
- BufferInputStream 缓冲字节输⼊流
# BufferedInputStream 通过预先读⼊⼀整段原始输⼊流数据⾄缓冲区中,⽽外界对BufferedInputStream的读取操作实际上是在缓冲区上进⾏,如果读取的数据超过了缓冲区的范围,那么BufferedInputStream负责重新从原始输⼊流中载⼊下⼀截数据填充缓冲区,然后外界继续通过缓冲区进⾏数据读取
# 好处:避免了⼤量的磁盘IO,原始的InputStream类实现的read是即时读取的,每⼀次读取都会是⼀次磁盘IO操作(哪怕只读取了1个字节的数据),如果数据量巨⼤,这样的磁盘消耗⾮常可怕
# 缓冲区的实现: 读取可以读取缓冲区中的内容,当读取超过缓冲区的内容后再进⾏⼀次磁盘IO,载⼊⼀段数据填充缓冲,下⼀次读取⼀般情况就直接可以从缓冲区读取,减少了磁盘IO
# 默认缓冲区⼤⼩是8k, int DEFAULT_BUFFER_SIZE = 8192
//对输⼊流进⾏包装,⾥⾯默认的缓冲区是8k
public BufferedInputStream(InputStream in);
//对输⼊流进⾏包装,指定创建具有指定缓冲区⼤⼩的
public BufferedInputStream(InputStream in,int size);
/从输⼊流中读取⼀个字节
public int read();
//从字节输⼊流中给定偏移量处开始将各字节读取到指定的 byte 数组中。
public int read(byte[] buf,int off,int len);
//关闭释放资源,关闭的时候这个流即可,InputStream会在⾥⾯被关闭
void close();
- BufferOutputStream 缓冲字节输出流
//对输出流进⾏包装,⾥⾯默认的缓冲区是8k
public BufferedOutputStream(OutputStream out);
//对输出流进⾏包装,指定创建具有指定缓冲区⼤⼩的
public BufferedOutputStream(OutputStream out,int size);
# 常⽤的三个⽅法
//向输出流中输出⼀个字节
public void write(int b);
//将指定 byte 数组中从偏移量 off 开始的 len 个字节写⼊缓冲的输出流。
public void write(byte[] buf,int off,int len);
//刷新此缓冲的输出流,强制使所有缓冲的输出字节被写出到底层输出流中。
public void flush();
//关闭释放资源,关闭的时候这个流即可,OutputStream会在⾥⾯被关闭, JDK7新特性try(在这⾥声明的会⾃动关闭){}
void close();
public class BufferMain {
public static void main(String [] args){
try{
FileInputStream fis = new FileInputStream("C:\\Users\\79466\\Desktop\\test\\a.txt");
BufferedInputStream bis = new BufferedInputStream(fis);
FileOutputStream fos = new FileOutputStream("C:\\Users\\79466\\Desktop\\test\\copy.txt");
BufferedOutputStream bos = new BufferedOutputStream(fos);
int size;
byte [] buf = new byte[1024];
while ( (size =bis.read(buf))!=-1){
bos.write(buf,0,size);
}
//刷新此缓冲区的输出流,才可以保证数据全部输出完成,
//bos.flush();
bis.close();
bos.close();
}catch (Exception e){
}
}
}