url=jdbc:mysql://127.0.0.1:3306/jdbc?useUnicode=true&characterEncoding=utf-8&useSSL=false
username=root
password=123456
driver=com.mysql.cj.jdbc.Driver
public class CustomDBUtil {
private static String url;
private static String username;
private static String password;
private static String driver;
static {
try {
Properties properties = new Properties();
properties.load(CustomDBUtil.class.getClassLoader().getResourceAsStream("db.properties"));
url = properties.getProperty("url");
username = properties.getProperty("username");
password = properties.getProperty("password");
driver = properties.getProperty("driver");
//加载JDBC驱动程序
Class.forName(driver);
}catch (Exception e){
e.printStackTrace();
}
}
/**
* 获取连接
* @return
* @throws Exception
*/
public static Connection getConnection() throws Exception{
Connection connection = DriverManager.getConnection(url,username,password);
return connection;
}
/**
* 关闭数据库资源
* @param resultSet
* @param ps
* @param connection
*/
public static void close(ResultSet resultSet, PreparedStatement ps, Connection connection){
try{
if(resultSet!=null){
resultSet.close();
}
if(ps!=null){
ps.close();
}
if(connection!=null){
connection.close();
}
}catch (SQLException e){
throw new RuntimeException();
}
}
}
@WebServlet("/jdbc")
public class TestServlet extends HttpServlet {
@Override
protected void doGet(HttpServletRequest req, HttpServletResponse resp) throws ServletException, IOException {
String idStr = req.getParameter("id");
int id = Integer.parseInt(idStr);
try {
Connection connection = CustomDBUtil.getConnection();
PreparedStatement ps = connection.prepareStatement("select * from user where id=?");
ps.setInt(1,id);
ResultSet resultSet = ps.executeQuery();
while (resultSet.next()){
System.out.println("用户名称 name="+ resultSet.getString("username") + " 联系方式 wechat="+ resultSet.getString("wechat"));
}
CustomDBUtil.close(resultSet,ps,connection);
} catch (Exception e) {
e.printStackTrace();
}
}
}
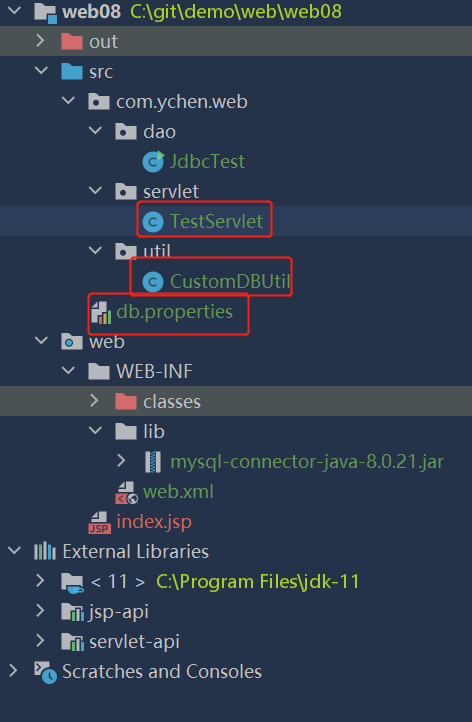
# 浏览器
http://localhost:8081/jdbc?id=1
# 控制台
�û����� name=jack ��ϵ��ʽ wechat=xdclass6
数据库建⽴Connection⽐较耗时,频繁的创建和释放连接引起的⼤量性能开销
如果数据库连接得到重⽤,避免这些开销,也提⾼了系统稳定
数据库连接池在初始化过程中,往往已经创建了若⼲数据库连接置于池中备⽤,对于业务请求处理⽽⾔,直接利⽤现有可⽤连接,缩减了系统整体响应时间
统⼀的连接管理,避免数据库连接泄漏、超时占⽤等问题