<script lang = "ts" scoped>
export default {
data() {
return {
}
},
methods: {
getUserList(){
this.$http.get("user/userList").then((response)=>{
console.log(response)
if(response.data.code == 200) {
console.log("获取成功!")
}
}).catch(error=>{console.error(error)});
}
},
created(){
this.getUserList();
}
}
</script>
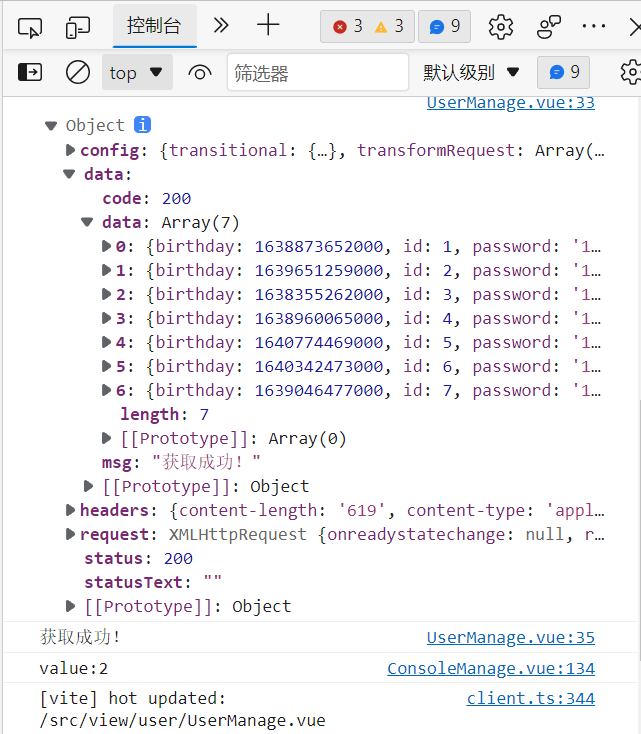
<script lang = "ts" scoped>
export default {
data() {
return {
}
},
methods: {
getUserList(){
const reqData = {
username: "chen1",
sex: "0"
}
this.$http.post("user/listAll", reqData).then((response)=>{
console.log(response)
if(response.data.code == 200) {
console.log("获取成功!")
}
}).catch(error=>{console.error(error)});
}
},
created(){
this.getUserList();
}
}
</script>
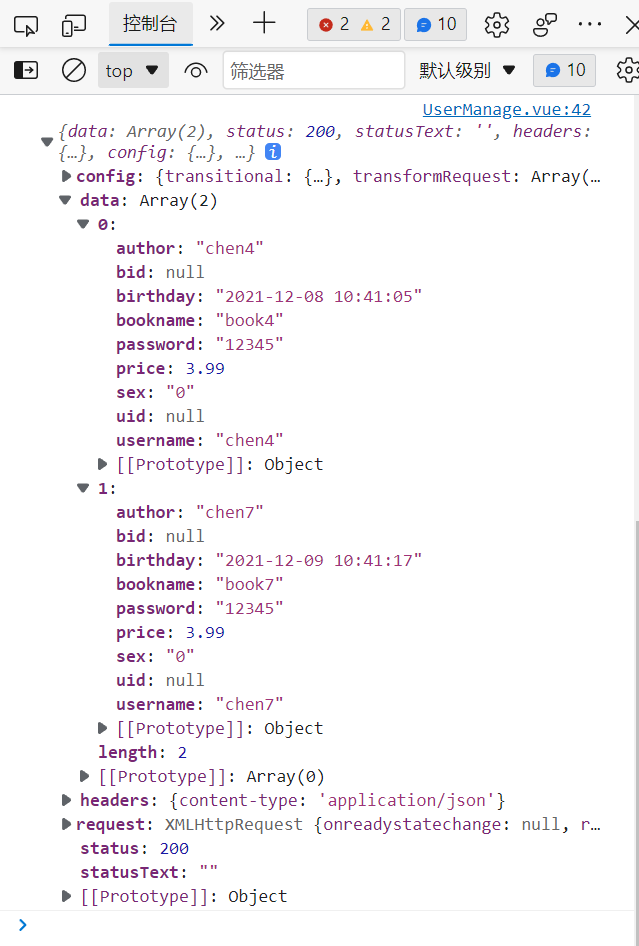
<script lang = "ts" scoped>
export default {
data() {
return {
UserList: []
}
},
methods: {
async getUserList(){
const reqData = {
username: "chen1",
sex: "0"
}
const {data: res} = await this.$http.post("user/listAll", reqData)
console.log(res)
this.UserList = res;
}
},
created(){
this.getUserList();
}
}
</script>
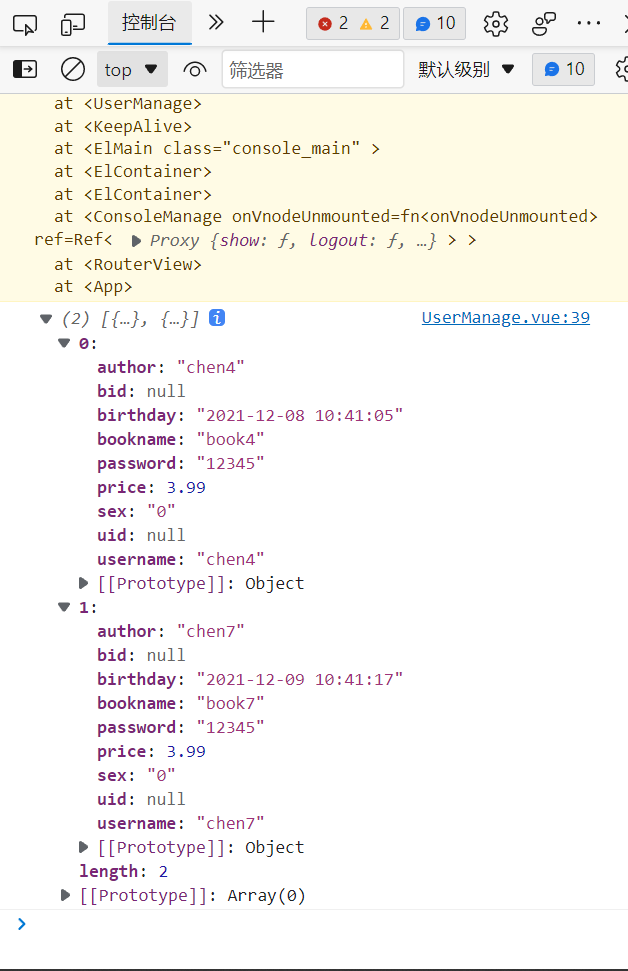
<el-table
class = "table"
:data="UserList"
style="width: 100%"
:row-class-name="tableRowClassName"
border
stript
>
<el-table-column type="index" label="#" />
<el-table-column prop="username" label="姓名" width="80" />
<el-table-column prop="password" label="密码" width="80" />
<el-table-column prop="price" label="价格" width="80" />
<el-table-column prop="bookname" label="书名" width="80" />
<el-table-column prop="birthday" label="生日" width="170" />
<el-table-column label="状态" width="100" />
<el-table-column label="操作" width="100" />
</el-table>
# js部分获取的数据
:data="UserList"
# 遍历字段
prop="username"
# 添加索引
<el-table-column type="index" label="#" />
<!- element ui中scope.row可获取到当前行的数据>
<template>
<div>
<el-table-column label="状态" width="100">
<template slot-scope="scope">
{{scope.row}}
</template>
<el-switch v-model="scope.row.state" />
</el-table-column>
</div>
</template>
<!- element plus中scope.row无法获取>
<template>
<div>
<el-switch v-model="scope.row.state" />
</div>
</template>
<!-- el-tooltip表示提示信息,:enterable="false"表示鼠标不能进入提示信息 -->
<el-table-column label="操作" width="300">
<el-tooltip
class="item"
effect="dark"
content="提示信息"
placement="top-start"
:enterable="false"
>
<el-button type="primary" :icon="Edit" size="mini">修改</el-button>
</el-tooltip>
<el-button type="primary" :icon="Share" size="mini">删除</el-button>
<el-button type="primary" :icon="Delete" size="mini">设置</el-button>
</el-table-column>
<template>
<div class="demo-pagination-block">
<span class="demonstration">All combined</span>
<el-pagination
v-model:currentPage="currentPage4"
:page-sizes="[100, 200, 300, 400]"
:page-size="100"
// total表示显示总数
layout="total, sizes, prev, pager, next, jumper"
:total="400"
// 每页多少条发生改变时
@size-change="handleSizeChange"
// 页数发生改变时
@current-change="handleCurrentChange"
>
</el-pagination>
</div>
</template>
<script lang="ts">
import { defineComponent, ref } from 'vue'
export default defineComponent({
setup() {
const handleSizeChange = (val) => {
console.log(`${val} items per page`)
// 当数据发送改变时,将数据保存到data函数中,之后发送请求获取所需数据
}
const handleCurrentChange = (val) => {
console.log(`current page: ${val}`)
}
return {
currentPage1: ref(5),
currentPage2: ref(5),
currentPage3: ref(5),
currentPage4: ref(4),
handleSizeChange,
handleCurrentChange,
}
},
})
</script>
使用v-for遍历时获取索引,v-on:click="show(item.id)"
- 超出隐藏
.span {
white-space: nowrap;
text-overflow:ellipsis;
overflow:hidden;
}