使用图标
# 安装
npm install --save @element-plus/icons-vue
- 在某子组件中引入并注册为自己的组件,就可以使用图标了
<template>
<div>
<el-icon><Avatar /></el-icon>
<el-icon><Unlock /></el-icon>
</div>
</template>
<script lang="ts">
import { Avatar, Unlock } from '@element-plus/icons'
export default {
components: {
Unlock,
Avatar
},
data() {
return {
}
}
}
</script>
<style lang="less">
</style>
# 安装依赖element plus
npm install element-plus@1.0.2-beta.69
# 安装图标依赖
npm install @element-plus/icons
<template>
<el-icon :size='20'><check /></el-icon>
</template>
<script lang = "ts" scoped>
// icon
import { Edit, Check, Coffee } from '@element-plus/icons'
export default {
components: {
Edit,
Check,
Coffee,
},
}
</script>
表单验证
- 官网
- 案例地址
vite2 + vue3 + element plus + sass + ts
案例一
// form使用表单验证
:rules="rules"
// 定义验证规则
rules: {
name: [
{
required: true,
message: 'Please input Activity name',
trigger: 'blur',
},
{
min: 5,
max: 10,
message: 'Length should be 3 to 5',
trigger: 'blur',
},
],
},
点击查看详情
<template>
<el-form
ref="ruleForm"
:model="ruleForm"
:rules="rules"
label-width="120px"
class="demo-ruleForm"
>
<!-- 文本框 -->
<el-form-item label="Activity name" prop="name">
<el-input v-model="ruleForm.name"></el-input>
</el-form-item>
<!-- 文本域 -->
<el-form-item label="Activity form" prop="desc">
<el-input v-model="ruleForm.desc" type="textarea"></el-input>
</el-form-item>
<!-- 提交按钮 -->
<el-form-item>
<el-button type="primary" @click="submitForm('ruleForm')"
>Create</el-button
>
<!-- 重置按钮 -->
<el-button @click="resetForm('ruleForm')" class="reset">Reset</el-button>
</el-form-item>
</el-form>
</template>
<script lang="ts" scoped>
export default {
data() {
return {
ruleForm: {
name: '',
desc: '',
},
// 验证规则
rules: {
name: [
{
required: true,
message: 'Please input Activity name',
trigger: 'blur',
},
{
min: 5,
max: 10,
message: 'Length should be 3 to 5',
trigger: 'blur',
},
],
},
}
},
// 提交按钮、重置按钮 的方法
methods: {
submitForm(formName) {
this.$refs[formName].validate((valid) => {
if (valid) {
alert('submit!')
} else {
console.log('error submit!!')
return false
}
})
},
resetForm(formName) {
this.$refs[formName].resetFields()
},
},
}
</script>
<style scoped>
.el-form {
border: 1px gray solid;
width: 500px;
height: 220px;
margin-top: 30px;
margin-left: 30px;
padding-top: 15px;
padding-right: 25px;
}
.reset{
margin-left: 20px;
}
</style>
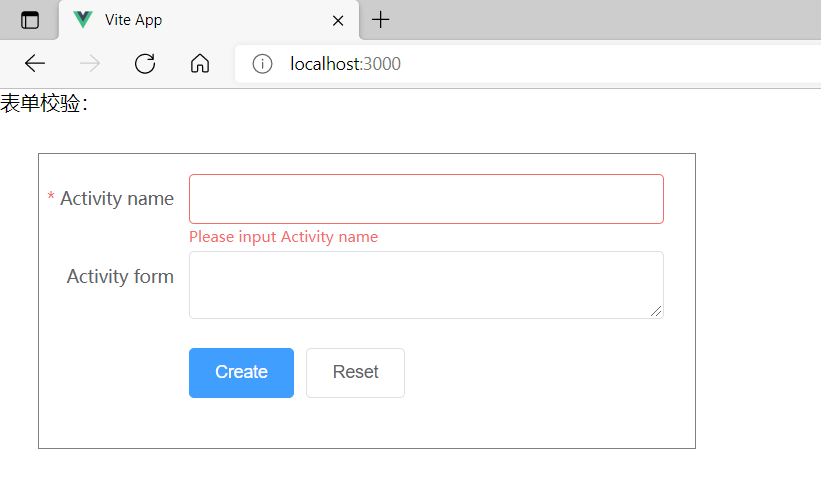
案例二
# form开启验证
:rules="rules"
# 自定义验证规则
data() {
// 回调方法
const checkAge = (rule, value, callback) => {
if (!value) {
return callback(new Error('Please input the age'))
}
setTimeout(() => {
if (!Number.isInteger(value)) {
callback(new Error('Please input digits'))
} else {
if (value < 18) {
callback(new Error('Age must be greater than 18'))
} else {
callback()
}
}
}, 1000)
}
const validatePass = (rule, value, callback) => {
if (value === '') {
callback(new Error('Please input the password'))
} else {
if (this.ruleForm.checkPass !== '') {
this.$refs.ruleForm.validateField('checkPass')
}
callback()
}
}
const validatePass2 = (rule, value, callback) => {
if (value === '') {
callback(new Error('Please input the password again'))
} else if (value !== this.ruleForm.pass) {
callback(new Error("Two inputs don't match!"))
} else {
callback()
}
}
return {
ruleForm: {
pass: '',
checkPass: '',
age: '',
},
// 自定义验证规则,使用回调方法
rules: {
pass: [{ validator: validatePass, trigger: 'blur' }],
checkPass: [{ validator: validatePass2, trigger: 'blur' }],
age: [{ validator: checkAge, trigger: 'blur' }],
},
}
},
点击查看详情
<template>
<el-form
ref="ruleForm"
:model="ruleForm"
status-icon
:rules="rules"
label-width="120px"
class="demo-ruleForm"
>
<!-- 密码框 -->
<el-form-item label="Password" prop="pass">
<el-input
v-model="ruleForm.pass"
type="password"
autocomplete="off"
></el-input>
</el-form-item>
<!-- 确认密码框 -->
<el-form-item label="Confirm" prop="checkPass">
<el-input
v-model="ruleForm.checkPass"
type="password"
autocomplete="off"
></el-input>
</el-form-item>
<!-- 年龄框 -->
<el-form-item label="Age" prop="age">
<el-input v-model.number="ruleForm.age"></el-input>
</el-form-item>
<el-form-item>
<!-- 提交按钮 -->
<el-button type="primary" @click="submitForm('ruleForm')"
>Submit</el-button
>
<!-- 重置按钮 -->
<el-button @click="resetForm('ruleForm')">Reset</el-button>
</el-form-item>
</el-form>
</template>
<script lang="ts" scoped>
export default {
data() {
// 回调方法
const checkAge = (rule, value, callback) => {
if (!value) {
return callback(new Error('Please input the age'))
}
setTimeout(() => {
if (!Number.isInteger(value)) {
callback(new Error('Please input digits'))
} else {
if (value < 18) {
callback(new Error('Age must be greater than 18'))
} else {
callback()
}
}
}, 1000)
}
const validatePass = (rule, value, callback) => {
if (value === '') {
callback(new Error('Please input the password'))
} else {
if (this.ruleForm.checkPass !== '') {
this.$refs.ruleForm.validateField('checkPass')
}
callback()
}
}
const validatePass2 = (rule, value, callback) => {
if (value === '') {
callback(new Error('Please input the password again'))
} else if (value !== this.ruleForm.pass) {
callback(new Error("Two inputs don't match!"))
} else {
callback()
}
}
return {
ruleForm: {
pass: '',
checkPass: '',
age: '',
},
// 自定义验证规则,使用回调方法
rules: {
pass: [{ validator: validatePass, trigger: 'blur' }],
checkPass: [{ validator: validatePass2, trigger: 'blur' }],
age: [{ validator: checkAge, trigger: 'blur' }],
},
}
},
// 提交按钮 重置按钮 的方法
methods: {
submitForm(formName) {
this.$refs[formName].validate((valid) => {
if (valid) {
alert('submit!')
} else {
console.log('error submit!!')
return false
}
})
},
resetForm(formName) {
this.$refs[formName].resetFields()
},
},
}
</script>
<style scoped>
.el-form {
border: 1px gray solid;
width: 500px;
height: 300px;
margin-top: 30px;
margin-left: 30px;
padding-top: 15px;
padding-right: 25px;
}
</style>
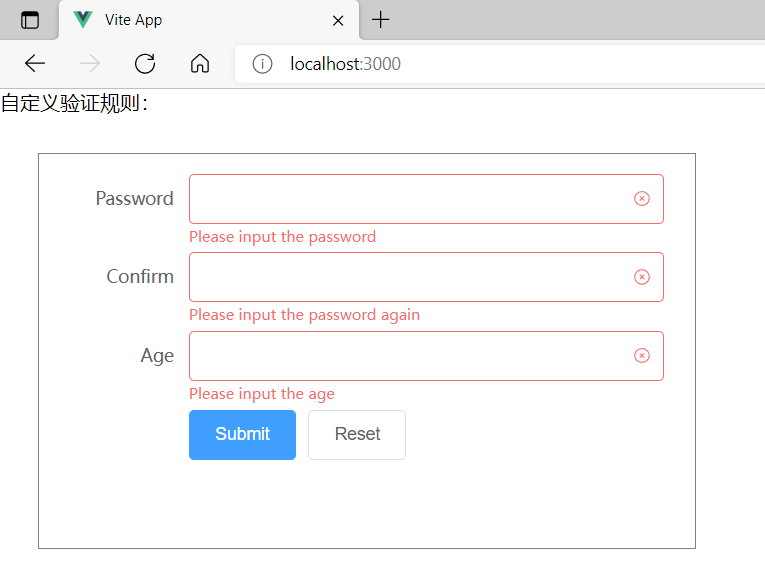