java.sql包
在使用jdbc之前,我们先看看有关操作jdbc会用到的几个类和接口,通过查看官方文档可知
- DriverManager:用于管理一组JDBC驱动程序的基本服务,即管理数据库中的所有驱动程序
- Connection:与特定数据库的连接(会话),执行SQL语句并在连接的上下文中返回结果,即用来建立数据库连接
- ResultSet:表示数据库结果集的数据表,通常通过执行查询数据库的语句生成,该接口类似于临时表,暂时存放数据库查询的结果集
- Statement:用于执行静态SQL语句并返回其生成的结果的对象,即管理sql语句,在已连接的基础上向数据发送sql语句
- PreparedStatement:继承了Statement,区别在于父类执行的是不带参的sql语句,子类则执行动态的sql语句
- CallableStatement:继承了PreparedStatement,用于执行SQL存储过程的界面
jdbc操作步骤
- 导入MySQL驱动
- 利用反射找到jar中的驱动
- 通过DriverManager获取connection连接对象来连接数据库
- 通过连接对象获取Statement对象,传入sql语句操作数据库
- 处理返回的结果集ResultSet
操作案例
新建一个数据库
- 这里我使用的navicat,数据库名jdbctest,表名t_user,id设置为自动增长
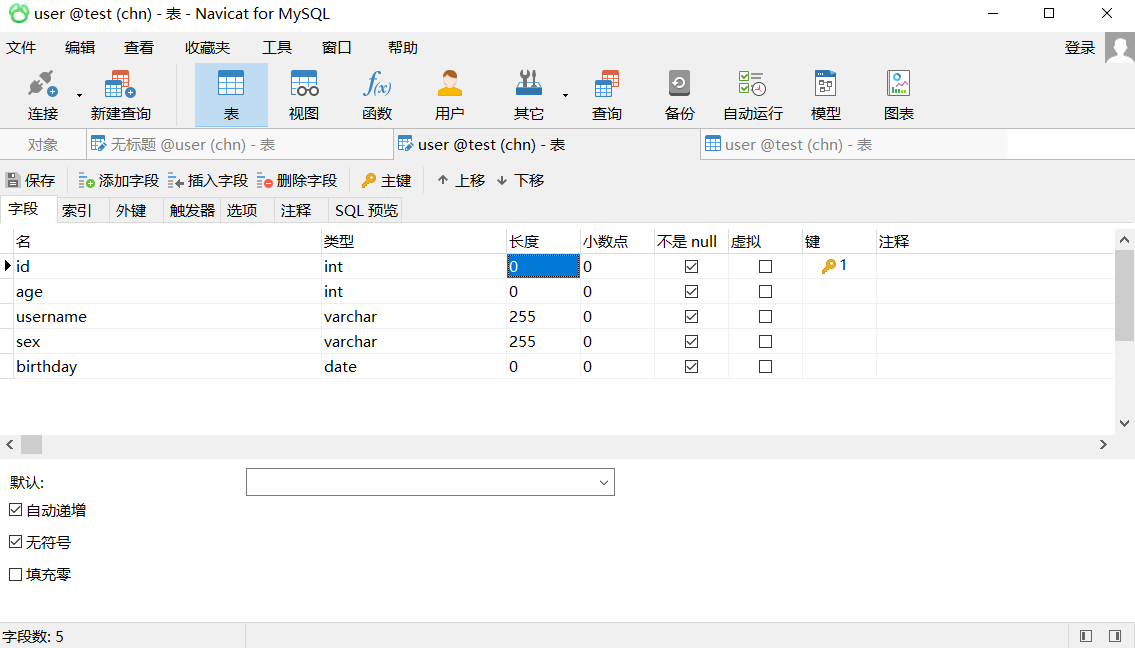
导入驱动
- 在IDEA中新建一个项目后,右键该项目 > Open Module Settings > Libraries > + > java
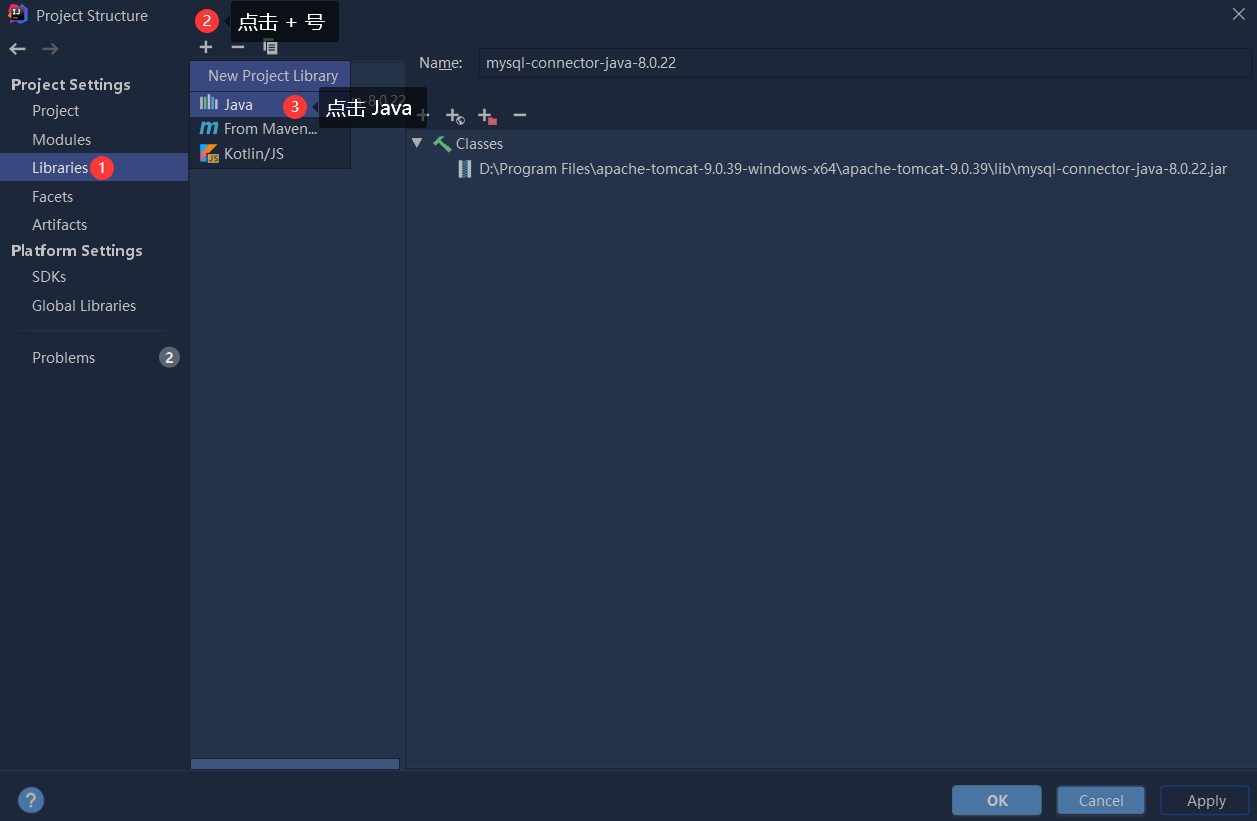
建一个实体类User
点击查看详细代码
import java.util.Date;
public class User {
private int id;
private int age;
private String username;
private String sex;
private Date birthday;
public int getId() {
return id;
}
public void setId(int id) {
this.id = id;
}
public int getAge() {
return age;
}
public void setAge(int age) {
this.age = age;
}
public String getUsername() {
return username;
}
public void setUsername(String username) {
this.username = username;
}
public String getSex() {
return sex;
}
public void setSex(String sex) {
this.sex = sex;
}
public Date getBirthday() {
return birthday;
}
public void setBirthday(Date birthday) {
this.birthday = birthday;
}
}
案例一:原始操作
点击查看详细代码
/**
* 查询t_user表中的所有数据
*/
public void getList() {
try {
//导入MySQL驱动,利用反射机制加载驱动程序
Class.forName("com.mysql.cj.jdbc.Driver");
//通过DriverManager获取connection对象
String url = "jdbc:mysql://localhost/jdbctest?user=root&password=root";
Connection conn = DriverManager.getConnection(url);
//通过Connection对象获取Statement对象
Statement statement = conn.createStatement();
//通过Statement对象操作数据库
String sql = "select * from t_user";
ResultSet rs = statement.executeQuery(sql);
//处理结果集
while (rs.next()) {
int id = rs.getInt(1); // 获取第一列的int类型的值
String username = rs.getString(2);
int age = rs.getInt(3);
String sex = rs.getString(4);
Date birthday = rs.getDate(5);
System.out.println("id:" + id + ", username:" + username + ", age:" + age + ", sex:" + sex + ",birthday:" + birthday);
}
} catch (ClassNotFoundException e) {
e.printStackTrace();
} catch (SQLException e) {
e.printStackTrace();
}
}
案例二:提取共有对象
点击查看详细代码
/**
* 传入参数userid,获取id对应的list对象
* 将Connection Statement ResultSet作为局部变量,最后需关闭
*/
public static List<Map<String, Object>> getList(int userid) {
Connection conn = null;
Statement statement = null;
ResultSet rs = null;
try {
Class.forName("com.mysql.cj.jdbc.Driver");
String url = "jdbc:mysql://localhost/jdbctest?user=root&password=root";
conn = DriverManager.getConnection(url);
statement = conn.createStatement();
String sql = "select * from t_user where id=" + userid;
rs = statement.executeQuery(sql);
//处理结果集
List<Map<String, Object>> lists = new ArrayList<Map<String,Object>>();
while (rs.next()) {
int id = rs.getInt("id");
String username = rs.getString("username");
int age = rs.getInt("age");
String sex = rs.getString("sex");
Date birthday = rs.getDate("birthday");
Map<String, Object> map = new HashMap<String, Object>();
map.put("id", id);
map.put("username", username);
map.put("age", age);
map.put("sex", sex);
map.put("birthday", birthday);
lists.add(map);
}
return lists;
} catch (ClassNotFoundException e) {
e.printStackTrace();
} catch (SQLException e) {
e.printStackTrace();
}finally {
if(rs != null) {
try {
rs.close();
} catch (SQLException e) {
e.printStackTrace();
}
}
if(statement != null) {
try {
statement.close();
} catch (SQLException e) {
e.printStackTrace();
}
}
if(conn != null) {
try {
conn.close();
} catch (SQLException e) {
e.printStackTrace();
}
}
}
return null;
}
案例三:防止SQL注入
点击查看详细代码
/**
* 按条件查询,
* 防止SQL注入,使用prepareStatement
* 传入两个参数,用prepareStatement的方法接受参数,操作MySQL
*/
public static List<Map<String, Object>> getList(int userid, String uname) {
Connection conn = null;
PreparedStatement ps = null;
ResultSet rs = null;
try {
Class.forName("com.mysql.cj.jdbc.Driver");
String url = "jdbc:mysql://localhost/jdbctest?user=root&password=root";
conn = DriverManager.getConnection(url);
//通过prepareStatement对象操作数据库
String sql = "select * from t_user where id = ? and username = ?";
ps = conn.prepareStatement(sql);
ps.setInt(1, userid);
ps.setString(2, uname);
//返回结果集
rs = ps.executeQuery();
//处理结果集
List<Map<String, Object>> lists = new ArrayList<Map<String,Object>>();
while (rs.next()) {
int id = rs.getInt("id");
String username = rs.getString("username");
int age = rs.getInt("age");
String sex = rs.getString("sex");
Date birthday = rs.getDate("birthday");
Map<String, Object> map = new HashMap<String, Object>();
map.put("id", id);
map.put("username", username);
map.put("age", age);
map.put("sex", sex);
map.put("birthday", birthday);
lists.add(map);
}
return lists;
} catch (ClassNotFoundException e) {
e.printStackTrace();
} catch (SQLException e) {
e.printStackTrace();
}finally {
if(rs != null) {
try {
rs.close();
} catch (SQLException e) {
e.printStackTrace();
}
}
if(ps != null) {
try {
ps.close();
} catch (SQLException e) {
e.printStackTrace();
}
}
if(conn != null) {
try {
conn.close();
} catch (SQLException e) {
e.printStackTrace();
}
}
}
return null;
}