python基础学习-day09==课后作业练习(字符串、列表、元组、字典)
# 1、有列表['alex',49,[1900,3,18]],分别取出列表中的名字,年龄,出生的年,月,日赋值给不同的变量
list=['alex',49,[1900,3,18]] #正向取值: name=list[0] print("姓名为:",name) #反向取值: age=list[-2] print("年龄为:",age) #pop删除最后一个元素,返回值 year, mon, day = list[2][:] print("出生年月为:%s年%s月%s日"%(year,mon,day))
运行结果:
# 2、用列表的insert与pop方法模拟队列
list1 =[] #队列是先进先出的 list1.insert(0,'1') list1.insert(0,'2') list1.insert(0,'3') print(list1) #从最后一个位置顺序出队 print(list1.pop()) print(list1.pop()) print(list1.pop())
运行结果:
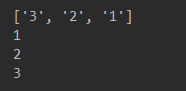
# 3. 用列表的insert与pop方法模拟堆栈
#堆栈是后进先出的 list2 = [] list2.insert(0,'1') list2.insert(0,'2') list2.insert(0,'3') print(list2) print(list2.pop(0))#从第一个位置顺序出栈 print(list2.pop(0)) print(list2.pop(0))
运行结果:
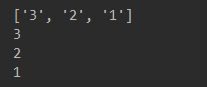
# 4、简单购物车,要求如下:
# 实现打印商品详细信息,用户输入商品名和购买个数,
# 则将商品名,价格,购买个数以三元组形式加入购物列表,
# 如果输入为空或其他非法输入则要求用户重新输入
# my_dict={ 'apple':10, 'tesla':100000, 'mac':3000, 'lenovo':30000, 'chicken':10, } #打印所有商品信息 print("=======商品详细信息有=============") print(my_dict) # 定义一个商品空列表 shop_list=[] shop_name =input("请输入商品名称:").strip() count= int(input("输入购买个数:")) flag = 1 while flag: if shop_name in my_dict and count!=0: price=int(my_dict[shop_name])*int(count) print('商品:%s,单价为:%s,数量:%s,总价为:%s'%(shop_name,my_dict[shop_name],count,price)) #把商品信息放入商品列表 shop_list.append(shop_name) shop_list.append(my_dict[shop_name]) shop_list.append(count) shop_list.append(price) shop_tuple = tuple(shop_list) #将列表转换成元组 print("商品购物列表为:",shop_tuple) flag = 0 else: print("没有该商品请重新输入!") shop_name = input('输入要购买的商品:') count = input('输入商品个数:') print("==========================\n\n\n\n")
运行结果:
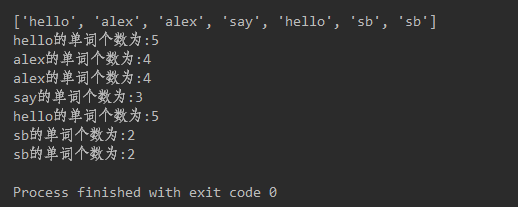
# 5、有如下值集合 [11,22,33,44,55,66,77,88,99,90...],将所有大于 66 的值保存至字典的第一个key中,
# 将小于 66 的值保存至第二个key的值中
#
# 即: {'k1': 大于66的所有值, 'k2': 小于66的所有值}
list=[11,22,33,44,55,66,77,88,99,90] #定义一个空字典 dict = {'k1': [], 'k2': []} #遍历list列表 for x in list: if x >= 66: dict['k1'].append(x) elif x < 66: dict['k2'].append(x) print(dict)
运行结果:

# 6、统计s='hello alex alex say hello sb sb'中每个单词的个数
# 计算单词中的字母个数 s='hello alex alex say hello sb sb' list=s.split(" ") #将字符串里的单词以空格进行切片成子字符串 print(list) for x in list: line=len(x) print("%s的单词中的字母个数为:%s" %(x,line)) #统计单词个数 # 方法一:字典计数 s='hello alex alex say hello sb sb' list=s.split(" ") #将字符串里的单词以空格进行切片成子字符串 print(list) dic = {} for line in list: # 如果当前的line不存在dic字典中,代表该单词是第一个出现,也就是个数为1 if line not in dic: # 若单词出现第一次,则将该单词当做字典的key,1作为字典的value # 初始化单词的个数 dic[line] = 1 else: # 若是重复出现的单词,则个数 += 1 dic[line] += 1 print(dic) # 方法二:使用count s='hello alex alex say hello sb sb' list=s.split(" ") #将字符串里的单词以空格进行切片成子字符串 print(list) dic = {} for line in list: # 如果当前的line不存在dic字典中,代表该单词是第一个出现,也就是个数为1 if line not in dic: dic[line] = list.count(line) print(dic)
运行结果:
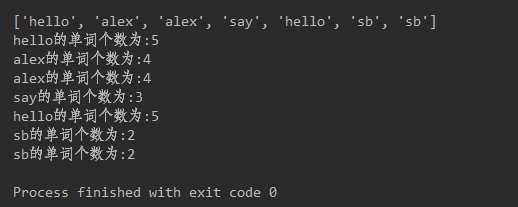
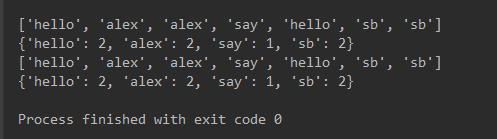