SpringBoot 整合篇 笔记--Spring Boot与检索
Spring Boot与检索
版本问题:我的版本如下:spring-boot 1.5.9 spring-boot-starter-data-elasticsearch 1.5.9 elasticsearch 2.3.5
<parent> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-parent</artifactId> <version>1.5.9.RELEASE</version> <relativePath/> <!-- lookup parent from repository --> </parent> <dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-data-elasticsearch</artifactId> <version>1.5.9.RELEASE</version> </dependency>
检索
我们的应用经常需要添加检索功能,开源的 ElasticSearch 是目前全文搜索引擎的首选。他可以快速的存储、搜索和分析海量数据。
Spring Boot通过整合SpringData ElasticSearch为我们提供了非常便捷的检索功能支持;
Elasticsearch是一个分布式搜索服务,提供Restful API,底层基于Lucene,采用多shard(分片)的方式保证数据安全,并且提供自动resharding的功能,github
等大型的站点也是采用了ElasticSearch作为其搜索服务,
安装ElasticSearch
问题1:限制堆空间内存,elasticSearch默认占用2G
docker run -d -p 9200:9200 -p 9300:9300 -e "ES_JAVA_OPTS=-Xms512m -Xmx512m" --name=myes elasticsearch:version
1.启动时修改 内存 -e "ES_JAVA_OPTS=-Xms512m -Xmx512m"
2.修改内存
启动成功:
概念
以 员工文档 的形式存储为例:一个文档代表一个员工数据。存储数据到ElasticSearch 的行为叫做 索引 ,但在索引一个文档之前,需要确定将文档存
储在哪里。
• 一个 ElasticSearch 集群可以 包含多个 索引 ,相应的每个索引可以包含多个 类型 。
这些不同的类型存储着多个 文档 ,每个文档又有 多个 属性 。
• 类似关系:
– 索引-数据库
– 类型-表
– 文档-表中的记录
– 属性-列
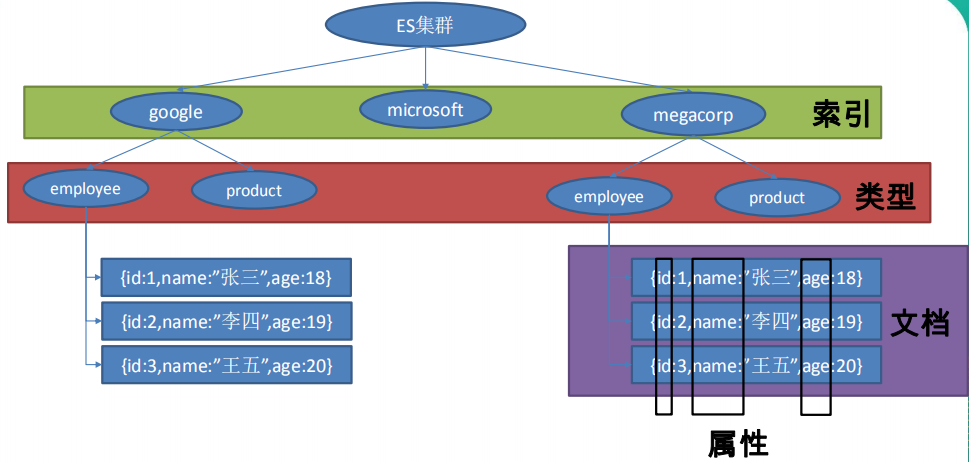
整合ElasticSearch测试
/** * SpringBoot默认支持两种技术来和ES交互; * 1、Jest(默认不生效) * 需要导入jest的工具包(io.searchbox.client.JestClient) * 2、SpringData ElasticSearch【ES版本有可能不合适】 * 版本适配说明:https://github.com/spring-projects/spring-data-elasticsearch * 如果版本不适配:2.4.6 * 1)、升级SpringBoot版本 * 2)、安装对应版本的ES * * 1)、Client 节点信息clusterNodes;clusterName * 2)、ElasticsearchTemplate 操作es * 3)、编写一个 ElasticsearchRepository 的子接口来操作ES; * 两种用法:https://github.com/spring-projects/spring-data-elasticsearch * 1)、编写一个 ElasticsearchRepository */ public static void main(String[] args) { SpringApplication.run(TestelasticsearchApplication.class, args); }
使用jest操作elasticSearch
1.配置文件
spring.elasticsearch.jest.uris=http://10.87.3.137:9200
2.引入依赖
<dependency> <groupId>io.searchbox</groupId> <artifactId>jest</artifactId> <version>2.0.0</version> </dependency>
3.测试类
@Autowired
JestClient jestClient;
@Test
public void contextLoads() throws IOException {
Article article = new Article();
article.setId(2);
article.setTitle("aaa");
article.setAuthor("bbb");
article.setContent("hello ccc");
Index build = new Index.Builder(article).index("atguigu").type("news").build();//构建一个索引功能
jestClient.execute(build);
}
/**
* 测试搜索
*/
@Test
public void search() throws IOException {
String json="{\n"+
" \"query\" :{\n"+
" \"match\" :{\n"+
" \"content\" : \"hello\"\n"+
" }\n"+
" }\n"+
"}";
Search build = new Search.Builder(json).addIndex("atguigu").addType("news").build();
SearchResult execute = jestClient.execute(build);
System.out.println("Message="+execute.getJsonString());
}
4.结果
编写一个 ElasticsearchRepository 的子接口来操作ES
1.引入
在pom文件中spring-boot-starter-data-elasticsearch
2.代码

package com.example.bean; import org.springframework.data.elasticsearch.annotations.Document; @Document(indexName = "atguigu",type = "book") public class Book { private Integer id; private String bookName; private String author; public Integer getId() { return id; } public void setId(Integer id) { this.id = id; } public String getBookName() { return bookName; } public void setBookName(String bookName) { this.bookName = bookName; } public String getAuthor() { return author; } public void setAuthor(String author) { this.author = author; } @Override public String toString() { return "Book{" + "id=" + id + ", bookName='" + bookName + '\'' + ", author='" + author + '\'' + '}'; } }

package com.example.repository; import com.example.bean.Book; import org.springframework.data.elasticsearch.repository.ElasticsearchRepository; import java.util.List; public interface BookRepository extends ElasticsearchRepository<Book,Integer> { public List<Book> findByBookNameLike(String bookName); }
3.测试
@Autowired BookRepository bookRepository; @Test public void test02(){ Book book = new Book(); book.setId(1); book.setBookName("西游记"); book.setAuthor("吴承恩"); bookRepository.index(book); // for (Book book : bookRepository.findByBookNameLike("游")) { // System.out.println(book); // } }
版本对应关系