IGPGraphicsPath.Flatten(); //把路径中的曲线转换为近似直线段(路径中只有 Bezier 线和直线). IGPGraphicsPath.Outline(); //同 Flatten(); IGPGraphicsPath.Warp(); //四边形或平行四边形扭曲. IGPGraphicsPath.Widen(); //把轮廓转换为范围. IGPGraphicsPath.Transform(); //矩阵变换; 其实上面几个方法都包含矩阵变换的参数.
Flatten 测试图:
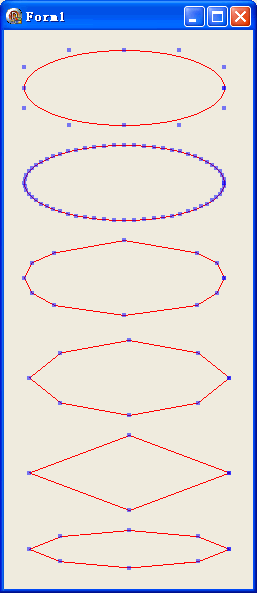
Flatten 测试代码:
uses GdiPlus; procedure TForm1.FormPaint(Sender: TObject); var Graphics: IGPGraphics; Pen: IGPPen; Brush: IGPBrush; Path, PathClone: IGPGraphicsPath; Rect: TGPRect; Pt: TGPPoint; Matrix: IGPMatrix; begin Graphics := TGPGraphics.Create(Canvas.Handle); Pen := TGPPen.Create($FFFF0000); Brush := TGPSolidBrush.Create($800000FF); Path := TGPGraphicsPath.Create; Rect.Initialize(20, 20, 200, 75); Path.AddEllipse(Rect); PathClone := Path.Clone; Graphics.DrawPath(Pen, PathClone); for Pt in PathClone.PathPointsI do Graphics.FillRectangle(Brush, Pt.X-2, Pt.Y-2, 4, 4); Graphics.TranslateTransform(0, Rect.Y + Rect.Height); PathClone := Path.Clone; PathClone.Flatten(); //其参数 2 默认是 0.25 Graphics.DrawPath(Pen, PathClone); for Pt in PathClone.PathPointsI do Graphics.FillRectangle(Brush, Pt.X-2, Pt.Y-2, 4, 4); Graphics.TranslateTransform(0, Rect.Y + Rect.Height); PathClone := Path.Clone; PathClone.Flatten(nil, 10); Graphics.DrawPath(Pen, PathClone); for Pt in PathClone.PathPointsI do Graphics.FillRectangle(Brush, Pt.X-2, Pt.Y-2, 4, 4); Graphics.TranslateTransform(0, Rect.Y + Rect.Height); PathClone := Path.Clone; PathClone.Flatten(nil, 25); Graphics.DrawPath(Pen, PathClone); for Pt in PathClone.PathPointsI do Graphics.FillRectangle(Brush, Pt.X-2, Pt.Y-2, 4, 4); Graphics.TranslateTransform(0, Rect.Y + Rect.Height); PathClone := Path.Clone; PathClone.Flatten(nil, 50); Graphics.DrawPath(Pen, PathClone); for Pt in PathClone.PathPointsI do Graphics.FillRectangle(Brush, Pt.X-2, Pt.Y-2, 4, 4); Graphics.TranslateTransform(0, Rect.Y + Rect.Height); PathClone := Path.Clone; Matrix := TGPMatrix.Create; Matrix.Scale(1, 0.5); Matrix.Translate(0, 20); PathClone.Flatten(Matrix, 25); Graphics.DrawPath(Pen, PathClone); for Pt in PathClone.PathPointsI do Graphics.FillRectangle(Brush, Pt.X-2, Pt.Y-2, 4, 4); Graphics.TranslateTransform(0, Rect.Y + Rect.Height); end;
Warp 测试图:
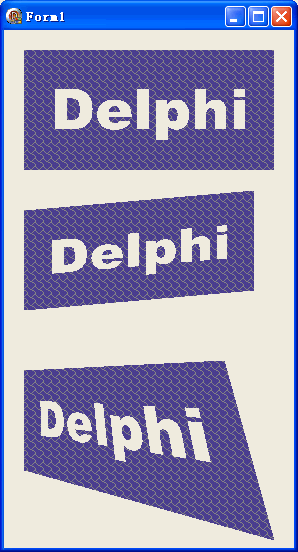
Warp 测试代码:
uses GdiPlus; const { 数组有三个元素时, 0: 左上; 1: 右上; 2: 左下; 3: 自动调整为平行四边形 } pts3: array[0..2] of TGPPointF = ((X:20; Y:20),(X:250; Y:0),(X:20; Y:120)); { 数组有四个元素时, 0: 左上; 1: 右上; 2: 左下; 3: 右下 } pts4: array[0..3] of TGPPointF = ((X:20; Y:20),(X:220; Y:10),(X:20; Y:120),(X:270; Y:190)); procedure TForm1.FormPaint(Sender: TObject); var Graphics: IGPGraphics; Brush: IGPHatchBrush; Path,PathClone: IGPGraphicsPath; Rect: TGPRectF; FontFamily: IGPFontFamily; StringFormat: IGPStringFormat; Pt: TGPPointF; begin Graphics := TGPGraphics.Create(Canvas.Handle); Brush := TGPHatchBrush.Create(HatchStyleShingle, $FF808080, $FF483D8B); Rect.Initialize(20, 20, 250, 120); FontFamily := TGPFontFamily.Create('Arial Black'); StringFormat := TGPStringFormat.Create; StringFormat.Alignment := StringAlignmentCenter; StringFormat.LineAlignment := StringAlignmentCenter; Path := TGPGraphicsPath.Create; Path.AddRectangle(Rect); Path.AddString('Delphi', FontFamily, [], 56, Rect, StringFormat); PathClone := Path.Clone; Graphics.FillPath(Brush, PathClone); Graphics.TranslateTransform(0, Rect.Y*2 + Rect.Height); //PathClone := Path.Clone; PathClone.Warp(pts3, Rect); Graphics.FillPath(Brush, PathClone); Graphics.TranslateTransform(0, Rect.Y*2 + Rect.Height); PathClone := Path.Clone; PathClone.Warp(pts4, Rect); Graphics.FillPath(Brush, PathClone); Graphics.TranslateTransform(0, Rect.Y*2 + Rect.Height); end;
Widen 测试图:
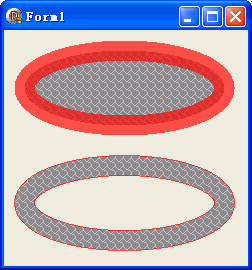
Widen 测试代码:
uses GdiPlus; procedure TForm1.FormPaint(Sender: TObject); var Graphics: IGPGraphics; Pen: IGPPen; Brush: IGPHatchBrush; Path, PathClone: IGPGraphicsPath; Rect: TGPRect; begin Graphics := TGPGraphics.Create(Canvas.Handle); Pen := TGPPen.Create($AAFF0000, 20); Brush := TGPHatchBrush.Create(HatchStyleShingle, $FFC0C0C0, $FF888888); Path := TGPGraphicsPath.Create; Rect.Initialize(20, 20, 200, 75); Path.AddEllipse(Rect); PathClone := Path.Clone; Graphics.FillPath(Brush, PathClone); Graphics.DrawPath(Pen, PathClone); Graphics.TranslateTransform(0, Rect.Y*2 + Rect.Height); PathClone := Path.Clone; PathClone.Widen(Pen); Graphics.FillPath(Brush, PathClone); Pen.Width := 1; Graphics.DrawPath(Pen, PathClone); Graphics.TranslateTransform(0, Rect.Y + Rect.Height); end;
Translate 测试图:
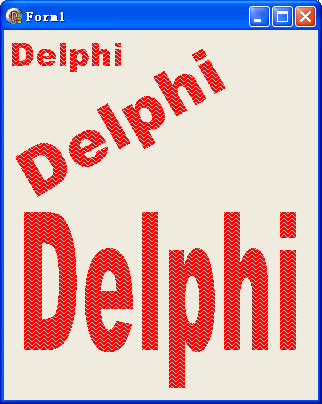
Translate 测试代码:
uses GdiPlus; procedure TForm1.FormPaint(Sender: TObject); var Graphics: IGPGraphics; Brush: IGPHatchBrush; Path,PathClone: IGPGraphicsPath; FontFamily: IGPFontFamily; Pt: TGPPointF; Matrix: IGPMatrix; begin Graphics := TGPGraphics.Create(Canvas.Handle); Brush := TGPHatchBrush.Create(HatchStyleZigZag, $FFC0C0C0, $FFFF0000); FontFamily := TGPFontFamily.Create('Arial Black'); Pt.Initialize(0, 0); Path := TGPGraphicsPath.Create; Path.AddString('Delphi', FontFamily, [], 32, Pt, nil); Graphics.FillPath(Brush, Path); PathClone := Path.Clone; Matrix := TGPMatrix.Create; Matrix.Scale(2, 2); Matrix.Rotate(-30); Matrix.Translate(-35, 45); PathClone.Transform(Matrix); Graphics.FillPath(Brush, PathClone); PathClone := Path.Clone; Matrix.Reset; Matrix.Scale(2.5, 6); Matrix.Translate(0, 18); PathClone.Transform(Matrix); Graphics.FillPath(Brush, PathClone); end;