项目结构
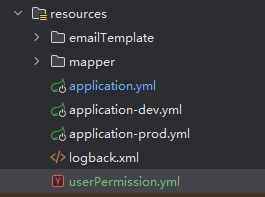
userPermission.yml
# 用户权限
user-permission:
api:
# 系统管理员
system_manager:
- "*:*:*"
# 应用作者
app_author:
- "test:data:query"
- "test:data:add"
# 应用用户
app_customer:
- "test:data:query"
- "test:data:add"
新建一个yml解析工厂 YmlConfigurationFactory.java
package cn.daenx.framework.YmlConfigurationFactory.config;
import org.springframework.boot.env.YamlPropertySourceLoader;
import org.springframework.core.env.PropertySource;
import org.springframework.core.io.support.DefaultPropertySourceFactory;
import org.springframework.core.io.support.EncodedResource;
import java.io.IOException;
public class YmlConfigurationFactory extends DefaultPropertySourceFactory {
@Override
public PropertySource<?> createPropertySource(String name, EncodedResource resource) throws IOException {
// 指定配置路径中的文件名
String filename = resource.getResource().getFilename() == null ? "" : resource.getResource().getFilename();
// 资源为空,则会抛出异常
resource.getInputStream();
if (resource == null || filename.endsWith(".properties")) {
// 配置文件为 properties 文件,调用原逻辑
return super.createPropertySource(name, resource);
} else if (filename.endsWith(".yml") || filename.endsWith(".yaml")) {
// yml文件 或 yaml文件,则使用yaml属性源加载器加载并返回标准属性源
return new YamlPropertySourceLoader().load(resource.getResource().getFilename(), resource.getResource()).get(0);
} else {
// 非以上情况,则直接抛出异常,提示格式错误
throw new IOException("file format error! only support: properties, yml, yaml!");
}
}
}
新建你的映射bean UserPermissionProperties.java
package cn.daenx.framework.userPermission;
import cn.daenx.framework.YmlConfigurationFactory.config.YmlConfigurationFactory;
import lombok.Data;
import org.springframework.boot.context.properties.ConfigurationProperties;
import org.springframework.context.annotation.Configuration;
import org.springframework.context.annotation.PropertySource;
import java.util.List;
import java.util.Map;
@Data
@Configuration
@PropertySource(
value = "classpath:userPermission.yml",
ignoreResourceNotFound = true,
encoding = "UTF-8",
factory = YmlConfigurationFactory.class
)
@ConfigurationProperties(prefix = "user-permission")
public class UserPermissionProperties {
private Map<String, List<String>> api;
}
使用
@Resource
private UserPermissionProperties userPermissionConfig;
/**
* 测试接口
*
* @return
*/
@GetMapping("/test0")
public Result test0() {
Map<String, List<String>> api = userPermissionConfig.getApi();
System.out.println(api);
return Result.ok("查询成功");
}