Thymeleaf遍历
编写User类
package com.xiang.pojo;
import com.fasterxml.jackson.annotation.JsonFormat;
import org.springframework.context.annotation.Configuration;
import org.springframework.format.annotation.DateTimeFormat;
import org.springframework.stereotype.Component;
import java.util.Date;
/**
* Created by IntelliJ IDEA.
* User: xiang
* Date: 2021/10/17 15:50
*/
@Component
@Configuration
public class User {
private String name;
private String gender;
private Integer age;
@DateTimeFormat(pattern = "yyyy-MM-dd")
@JsonFormat(pattern = "yyyy-MM-dd")
private Date birthday;
// private DateTimeFormatter birthday;
public User(String name, String gender, Integer age, Date birthday) {
this.name = name;
this.gender = gender;
this.age = age;
this.birthday = birthday;
}
public User() {
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public String getGender() {
return gender;
}
public void setGender(String gender) {
this.gender = gender;
}
public Integer getAge() {
return age;
}
public void setAge(Integer age) {
this.age = age;
}
public Date getBirthday() {
return birthday;
}
public void setBirthday(Date birthday) {
this.birthday = birthday;
}
@Override
public String toString() {
return "User{" +
"name='" + name + '\'' +
", gender='" + gender + '\'' +
", age=" + age +
", birthday=" + birthday +
'}';
}
}
编写ThymeleafEachController类
package com.xiang.controller;
import com.xiang.pojo.User;
import org.springframework.stereotype.Controller;
import org.springframework.ui.Model;
import org.springframework.web.bind.annotation.RequestMapping;
import java.text.ParseException;
import java.text.SimpleDateFormat;
import java.time.LocalDateTime;
import java.time.format.DateTimeFormatter;
import java.util.ArrayList;
import java.util.Calendar;
import java.util.Date;
/**
* Created by IntelliJ IDEA.
* User: xiang
* Date: 2021/10/17 15:47
*/
@Controller
public class ThymeleafEachController {
@RequestMapping("/each")
public String each(Model model) {
try {
ArrayList<User> users = new ArrayList<>();
SimpleDateFormat format = new SimpleDateFormat("yyyy-MM-dd");
Date date = format.parse("2000-11-1");
User user = new User("xiang", "男", 18, date);
User user2 = new User("xiao_xiang", "男", 18, date);
User user3 = new User("xiang_xiang", "男", 18, date);
users.add(user);
users.add(user2);
users.add(user3);
model.addAttribute("users", users);
} catch (ParseException e) {
e.printStackTrace();
}
return "each";
}
}
编写each.html
<!DOCTYPE html>
<html lang="en" xmlns:th="http://www.thymeleaf.org" xmlns:fmt="">
<head>
<meta charset="UTF-8">
<title>Thymeleaf-Each</title>
<style>
div {
margin-top: 10px;
background-color: pink;
tab-size: 20px;
}
table{
margin-top: 30px;
}
</style>
</head>
<body>
<h2>
Thymeleaf - Each
</h2>
<div th:text="${users.get(0)}"></div>
<div th:text="${users.get(1)}"></div>
<div th:text="${users.get(2)}"></div>
<table border="2">
<tr>
<th>序号</th>
<th>姓名</th>
<th>性别</th>
<th>年龄</th>
<th>生日(美国日期)</th>
<!-- <th>生日(中国日期)</th>-->
</tr>
<tr th:each="users,user:${users}">
<!--可以得到循环次数-->
<td th:text="${user.count}"></td>
<td th:text="${users.getName()}"></td>
<td th:text="${users.gender}"></td>
<td th:text="${users.getAge()}"></td>
<td th:text="${users.birthday}"></td>
<!-- <td th:text="${users.getBirthday()}"></td>-->
<!-- <td fmt:formatDate pattern="yyyy-MM-dd HH:mm" value="${model.birthday}"></td>-->
</tr>
</table>
</body>
</html>
运行结果
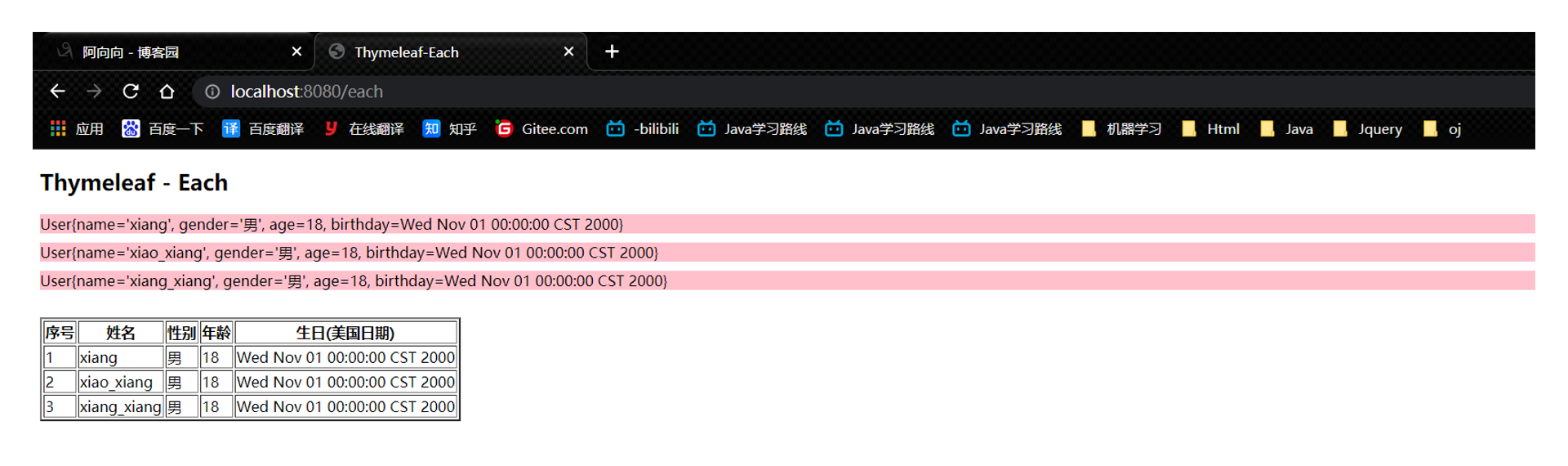