springMVC 实现图片上传
1、新建项目工程
2、配置Tomcat
3、编写pom.xml文件
<?xml version="1.0" encoding="UTF-8"?>
<project xmlns="http://maven.apache.org/POM/4.0.0"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 https://maven.apache.org/xsd/maven-4.0.0.xsd">
<modelVersion>4.0.0</modelVersion>
<groupId>com.xiang</groupId>
<artifactId>viewDemo</artifactId>
<version>1.0-SNAPSHOT</version>
<name>viewDemo</name>
<packaging>war</packaging>
<!-- 依赖软件包版本 -->
<properties>
<commons-lang.version>2.6</commons-lang.version>
<spring.version>4.1.3.RELEASE</spring.version>
<slf4j.version>1.7.6</slf4j.version>
<maven.compiler.target>1.8</maven.compiler.target>
<maven.compiler.source>1.8</maven.compiler.source>
<junit.version>5.7.1</junit.version>
</properties>
<!-- 配置依赖管理包 -->
<dependencyManagement>
<dependencies>
<dependency>
<groupId>org.springframework</groupId>
<artifactId>spring-framework-bom</artifactId>
<version>${spring.version}</version>
<type>pom</type>
<scope>import</scope>
</dependency>
</dependencies>
</dependencyManagement>
<!-- sprint MVC 依赖包 -->
<dependencies>
<dependency>
<groupId>org.springframework</groupId>
<artifactId>spring-webmvc</artifactId>
<version>3.2.3.RELEASE</version>
</dependency>
<!-- 通用依赖包 -->
<dependency>
<groupId>commons-lang</groupId>
<artifactId>commons-lang</artifactId>
<version>${commons-lang.version}</version>
</dependency>
<!-- log4j依赖包 -->
<dependency>
<groupId>org.slf4j</groupId>
<artifactId>slf4j-log4j12</artifactId>
<version>1.7.6</version>
<exclusions>
<exclusion>
<groupId>org.slf4j</groupId>
<artifactId>slf4j-api</artifactId>
</exclusion>
</exclusions>
</dependency>
<dependency>
<groupId>org.slf4j</groupId>
<artifactId>slf4j-api</artifactId>
<version>${slf4j.version}</version>
</dependency>
<!-- 文件上传 -->
<dependency>
<groupId>commons-fileupload</groupId>
<artifactId>commons-fileupload</artifactId>
<version>1.3.1</version>
</dependency>
<!-- jstl标签包 -->
<dependency>
<groupId>javax.servlet</groupId>
<artifactId>jstl</artifactId>
<version>1.2</version>
</dependency>
<!-- xml与实体转换依赖-->
<dependency>
<groupId>javax.xml.bind</groupId>
<artifactId>jaxb-api</artifactId>
<version>2.2.7</version>
</dependency>
<dependency>
<groupId>com.sun.xml.bind</groupId>
<artifactId>jaxb-impl</artifactId>
<version>2.2.7</version>
</dependency>
<dependency>
<groupId>org.springframework</groupId>
<artifactId>spring-oxm</artifactId>
<version>${spring.version}</version>
</dependency>
<!-- json依赖包 -->
<dependency>
<groupId>com.fasterxml.jackson.core</groupId>
<artifactId>jackson-core</artifactId>
<version>2.7.4</version>
</dependency>
<dependency>
<groupId>com.fasterxml.jackson.core</groupId>
<artifactId>jackson-annotations</artifactId>
<version>2.7.4</version>
</dependency>
<dependency>
<groupId>org.codehaus.jackson</groupId>
<artifactId>jackson-mapper-asl</artifactId>
<version>1.9.13</version>
</dependency>
<dependency>
<groupId>com.fasterxml.jackson.core</groupId>
<artifactId>jackson-databind</artifactId>
<version>2.7.4</version>
</dependency>
<!-- mysql连接 -->
<dependency>
<groupId>mysql</groupId>
<artifactId>mysql-connector-java</artifactId>
<version>8.0.25</version>
</dependency>
<!-- 数据源 c3p0 -->
<dependency>
<groupId>c3p0</groupId>
<artifactId>c3p0</artifactId>
<version>0.9.1.2</version>
</dependency>
<!--Spring dao管理 -->
<dependency>
<groupId>org.springframework</groupId>
<artifactId>spring-dao</artifactId>
<version>2.0.3</version>
</dependency>
<dependency>
<groupId>junit</groupId>
<artifactId>junit</artifactId>
<version>4.13</version>
</dependency>
<dependency>
<groupId>org.springframework</groupId>
<artifactId>spring-test</artifactId>
<version>3.2.3.RELEASE</version>
</dependency>
<!--mybatis核心包-->
<dependency>
<groupId>org.mybatis</groupId>
<artifactId>mybatis</artifactId>
<version>3.2.8</version>
</dependency>
<!-- mybatis和spring集成包-->
<dependency>
<groupId>org.mybatis</groupId>
<artifactId>mybatis-spring</artifactId>
<version>1.3.0</version>
</dependency>
<!-- spring创建数据源的依赖包-->
<dependency>
<groupId>org.springframework</groupId>
<artifactId>spring-jdbc</artifactId>
<version>4.2.5.RELEASE</version>
</dependency>
<!-- AspectJ -->
<dependency>
<groupId>org.aspectj</groupId>
<artifactId>aspectjweaver</artifactId>
<version>1.8.6</version>
</dependency>
</dependencies>
<build>
<plugins>
<plugin>
<groupId>org.apache.maven.plugins</groupId>
<artifactId>maven-war-plugin</artifactId>
<version>3.3.1</version>
</plugin>
</plugins>
</build>
</project>
4、编写mvc-dispatcher-servlet.xml文件
<?xml version="1.0" encoding="UTF-8"?>
<beans xmlns="http://www.springframework.org/schema/beans"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xmlns:context="http://www.springframework.org/schema/context"
xmlns:mvc="http://www.springframework.org/schema/mvc"
xsi:schemaLocation="
http://www.springframework.org/schema/beans
http://www.springframework.org/schema/beans/spring-beans.xsd
http://www.springframework.org/schema/context
http://www.springframework.org/schema/context/spring-context.xsd
http://www.springframework.org/schema/mvc
http://www.springframework.org/schema/mvc/spring-mvc.xsd">
<!-- 扫描控制器 -->
<context:component-scan base-package="com.xiang.controller">
<context:include-filter type="annotation"
expression="org.springframework.stereotype.Controller" />
</context:component-scan>
<!-- 扩充了注解驱动,可以将请求参数绑定到控制器参数 -->
<mvc:annotation-driven />
<!-- 静态资源处理, css, js, imgs -->
<!-- <mvc:resources mapping="/resources/css/**" location="/resources/css/" />-->
<!-- <mvc:resources mapping="/resources/video/**" location="/resources/video/" />-->
<!-- <mvc:resources mapping="/resources/pic/**" location="/resources/pic/" />-->
<mvc:resources mapping="/upload/**" location="/upload/" />
<!--协商视图器,可以自定义解析器-->
<bean class="org.springframework.web.servlet.view.ContentNegotiatingViewResolver">
<property name="order" value="1"></property>
<property name="mediaTypes">
<map>
<entry key="json" value="application/json"/>
<entry key="xml" value="application/xml" />
</map>
</property>
<property name="defaultViews">
<list>
<bean class="org.springframework.web.servlet.view.json.MappingJackson2JsonView"></bean>
<bean class="org.springframework.web.servlet.view.xml.MarshallingView">
<constructor-arg>
<bean class="org.springframework.oxm.jaxb.Jaxb2Marshaller">
<property name="classesToBeBound">
<list>
<value>com.xiang.pojo.User</value>
</list>
</property>
</bean>
</constructor-arg>
</bean>
</list>
</property>
<!--打开请求头-->
<property name="ignoreAcceptHeader" value="false"></property>
</bean>
<!--文件上传解析器, resolveLazily属性启用是为了推迟文件解析,以便捕获文件大小异常 -->
<bean id="multipartResolver"
class="org.springframework.web.multipart.commons.CommonsMultipartResolver">
<property name="maxUploadSize" value="209715200" />
<property name="defaultEncoding" value="UTF-8" />
<property name="resolveLazily" value="true" />
</bean>
<!-- 配置试图解析器 -->
<bean
class="org.springframework.web.servlet.view.InternalResourceViewResolver">
<property name="viewClass"
value="org.springframework.web.servlet.view.JstlView"></property>
<!-- 配置jsp文件前缀及后缀 -->
<property name="prefix" value="/"></property>
<property name="suffix" value=".jsp"></property>
</bean>
</beans>
5、编写springApplication.xml文件
<?xml version="1.0" encoding="UTF-8"?>
<beans xmlns="http://www.springframework.org/schema/beans"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://www.springframework.org/schema/beans
http://www.springframework.org/schema/beans/spring-beans-4.0.xsd
">
</beans>
6、配置web.xml文件
<!DOCTYPE web-app>
<web-app>
<display-name>Archetype Created Web Application</display-name>
<!-- 欢迎页面 -->
<welcome-file-list>
<welcome-file>index.jsp</welcome-file>
</welcome-file-list>
<!-- 配置spring 上下文 -->
<context-param>
<param-name>contextConfigLocation</param-name>
<param-value>classpath:springApplication.xml</param-value>
</context-param>
<!-- 配置监听器 -->
<listener>
<listener-class>org.springframework.web.context.ContextLoaderListener</listener-class>
</listener>
<!-- spring mvc的配置上下文 -->
<servlet>
<servlet-name>mvc-dispatcher</servlet-name>
<servlet-class>org.springframework.web.servlet.DispatcherServlet</servlet-class>
<init-param>
<param-name>contextConfigLocation</param-name>
<param-value>classpath:mvc-dispatcher-servlet.xml</param-value>
</init-param>
<load-on-startup>1</load-on-startup>
</servlet>
<servlet-mapping>
<servlet-name>mvc-dispatcher</servlet-name>
<!-- 拦截所有请求 -->
<url-pattern>/</url-pattern>
</servlet-mapping>
<!-- 编码过滤器 -->
<filter>
<filter-name>characterEncodingFilter</filter-name>
<filter-class>org.springframework.web.filter.CharacterEncodingFilter</filter-class>
<init-param>
<param-name>encoding</param-name>
<param-value>UTF-8</param-value>
</init-param>
<init-param>
<param-name>forceEncoding</param-name>
<param-value>true</param-value>
</init-param>
</filter>
<filter-mapping>
<filter-name>characterEncodingFilter</filter-name>
<url-pattern>/*</url-pattern>
</filter-mapping>
</web-app>
7、 编写controller.FileUploadController类
package com.xiang.controller;
import org.apache.commons.io.FileUtils;
import org.springframework.stereotype.Controller;
import org.springframework.ui.Model;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RequestMethod;
import org.springframework.web.bind.annotation.RequestParam;
import org.springframework.web.multipart.MultipartFile;
import org.springframework.web.multipart.MultipartHttpServletRequest;
import javax.servlet.http.HttpServletRequest;
import java.io.File;
import java.io.IOException;
/**
* Created by IntelliJ IDEA.
* User: xiang
* Date: 2021/9/13 11:02
*/
@Controller
@RequestMapping(value = "/file")
public class FileUploadController {
// @RequestMapping(value = "/up", method = RequestMethod.POST)
// public String uploadFile(@RequestParam("imgPath") MultipartFile file, Model model, MultipartHttpServletRequest request) throws Exception {
// //判断目录是否在服务器上,如果没有我们创建一个目录,并把上传文件放在目录下面
// if (!file.isEmpty()) {
// String imgPath =
// request.getSession().getServletContext().getRealPath("")
// + file.getOriginalFilename();
// System.out.println(imgPath);
// //上传文件到指定目录
// FileUtils.copyInputStreamToFile(file.getInputStream(), new File(imgPath));
// model.addAttribute("url",imgPath);
// }
// return "view/res";
// }
@RequestMapping(value = "/up2", method = RequestMethod.POST)
public String uploadFile2(@RequestParam("imgPath") MultipartFile imgPath, Model model, HttpServletRequest request) throws IOException {
String realPath = request.getSession().getServletContext().getRealPath("/upload/");
File dir = new File(realPath);
if(!dir.exists()){
dir.mkdirs();
}
// 获取文件名
String originalFilename = imgPath.getOriginalFilename();
// 文件存储位置
File file =new File(dir,originalFilename);
// 文件保存
imgPath.transferTo(file);
/*//上传图片
FileUtils.copyInputStreamToFile(file.getInputStream(),new File(imgPath));*/
System.out.println(file);
// model.addAttribute("")
model.addAttribute("url",file);
model.addAttribute("name",originalFilename);
return "view/res";
}
}
8、编写controller.FileController类
package com.xiang.controller;
import org.springframework.stereotype.Controller;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RequestMethod;
/**
* Created by IntelliJ IDEA.
* User: xiang
* Date: 2021/9/13 11:12
*/
@Controller
public class FileController {
@RequestMapping(value = "/vi",method = RequestMethod.GET)
public String getToFile(){
return "view/toFile";
}
}
9、编写view.toFile.jsp文件
<%--
Created by IntelliJ IDEA.
User: Xiang
Date: 2021/9/13
Time: 11:02
To change this template use File | Settings | File Templates.
--%>
<%@ page contentType="text/html;charset=UTF-8" language="java" %>
<html>
<head>
<title>toFile</title>
</head>
<body>
<div>
<%-- <form action="<%=request.getContextPath()%>/file/up" enctype="multipart/form-data" method="post">--%>
<form action="<%=request.getContextPath()%>/file/up2" enctype="multipart/form-data" method="post">
<input type="file" name="imgPath">
<input type="submit" value="上传">
</form>
</div>
</body>
</html>
10、编写view.res.jsp文件
<%--
Created by IntelliJ IDEA.
User: Xiang
Date: 2021/9/13
Time: 11:16
To change this template use File | Settings | File Templates.
--%>
<%@ page contentType="text/html;charset=UTF-8" language="java" isELIgnored="false" %>
<html>
<head>
<title>res</title>
</head>
<body>
<h3 style="color: red">
success
</h3>
<%--<img src="${url}" width="500px" height="500px">--%>
<img src="http://127.0.0.1:8001/upload/${name}"/>
</body>
</html>
11、项目工程运行截图
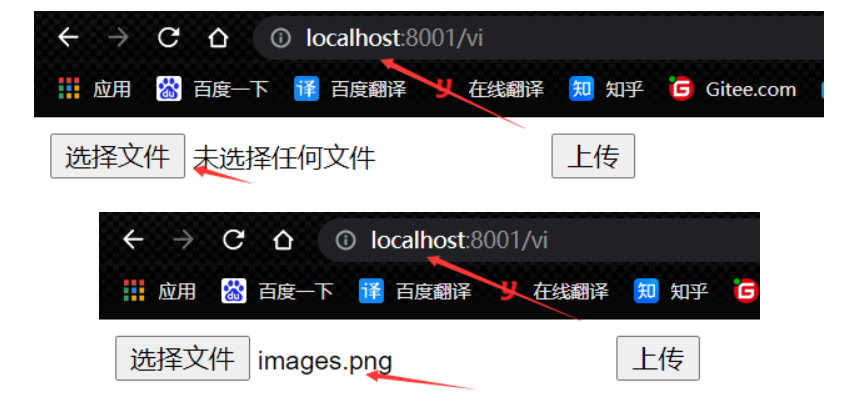
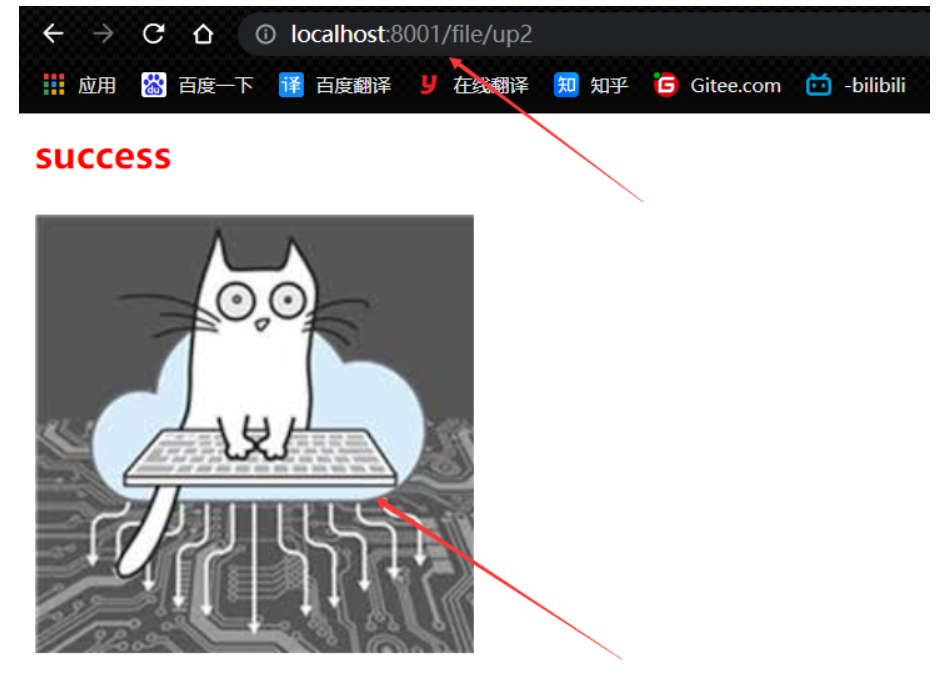