任务三:
测试代码>
pets.hpp
点击查看代码
#pragma once
#include<iostream>
#include<string>
using namespace std;
class MachinePets {
public:
MachinePets(const string& s):nickname{s}{}
virtual string talk()const = 0;
string get_nickname() {
return nickname;
}
private:
string nickname;
};
class PetCats:public MachinePets {
public:
PetCats(const string& s):MachinePets(s){}
string talk()const override {
return "miao wu~";
}
};
class PetDogs:public MachinePets {
public:
PetDogs(const string& s):MachinePets(s){}
string talk() const override{
return "wang wang~";
}
};
任务四:
测试代码:
点击查看代码
#pragma once
#include<string>
#include<iostream>
#include<iomanip>
using namespace std;
class Film {
public:
string get_year() const{
return year;
}
friend ostream& operator<<(ostream& cout, Film& f);
friend istream& operator>>(istream& cin, Film& f);
private:
string name;
string director;
string area;
string year;
};
istream& operator>>(istream& cin, Film& f) {
string s1, s2, s3, s4;
cout << "录入片名:";
cin >> s1;
f.name = s1;
cout << "录入导演:";
cin >> s2;
f.director = s2;
cout << "录入制片国家/地区:";
cin >> s3;
f.area = s3;
cout << "录入上映年份:";
cin >> s4;
f.year = s4;
return cin;
}
ostream& operator<<(ostream& cout, Film& f) {
cout << setw(16) << left << f.name;
cout << setw(16) << left << f.director;
cout << setw(16) << left << f.area;
cout << setw(16) << left << f.year;
return cout;
}
bool compare_by_year(const Film& f1, const Film& f2) {
return f1.get_year() < f2.get_year();//升序---从小到大
}
任务五:
代码:
点击查看代码
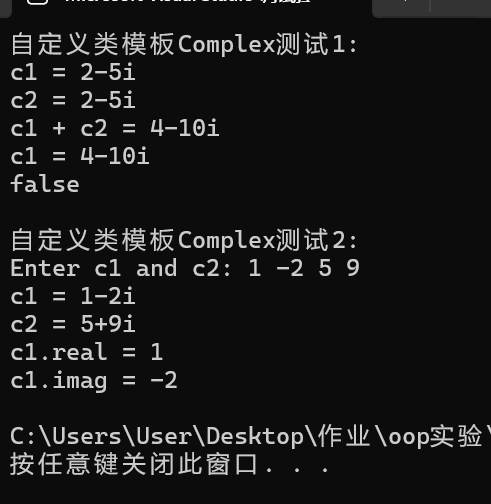
任务六
代码
点击查看代码
#pragma once
#include"date.h"
#include"accumulator.h"
#include<string>
using namespace std;
class Account {
private:
string id;
double balance;
static double total;
protected:
Account(const Date & date, const string & id);
void record(const Date & date, double amount, const string & desc);
void error(const string & msg) const;
public:const string & getId() { return id; }
double getBalance()const { return balance; }
static double getTotal() { return total; }
virtual void deposit(const Date & date, double amount, const string & desc) = 0;
virtual void withdraw(const Date & date, double amount, const string & desc) = 0;
virtual void settle(const Date & date) = 0;
virtual void show()const;
};
class SavingsAccount :public Account {
private:
Accumulator acc;
double rate;
public:
SavingsAccount(const Date & date, const string & id, double rate);
double getRate() const { return rate; }
void deposit(const Date & date, double amount, const string & desc);
void withdraw(const Date & date, double amount, const string & desc);
void settle(const Date & date);
};
class CreditAccount :public Account {
private:
Accumulator acc;
double credit;
double rate;
double fee;
double getDebt()const {
double balance = getBalance();
return(balance < 0 ? balance : 0);
}
public:CreditAccount(const Date & date, const string & id, double credit, double rate, double fee);
double getCredit()const { return credit; }
double getRate()const { return rate; }
double getFee() const { return fee; }
double getAvailableCredit()const {
if (getBalance() < 0)return credit + getBalance();
else return credit;
}
void deposit(const Date & date, double amount, const string & desc);
void withdraw(const Date & date, double amount, const string & desc);
void settle(const Date & date);
void show()const;
};
#pragma once
#include "date.h"
class Accumulator {
private:
Date lastDate;
double value;
double sum;
public:
Accumulator(const Date & date, double value) :lastDate(date), value(value), sum{ 0 } {}
double getSum(const Date & date) const {
return sum + value * (date - lastDate);
}
void change(const Date & date, double value) {
sum = getSum(date);
lastDate = date;
this->value = value;
}
void reset(const Date & date, double value) {
lastDate = date;
this->value;
sum = 0;
}
};
#pragma once
class Date {
private:
int year;
int month;
int day;
int totalDays;
public:
Date(int year, int month, int day);
int getYear()const { return year; }
int getMonth()const { return month; }
int getDay()const { return day; }
int getMaxDay()const;
bool isLeapYear()const {
return year % 4 == 0 && year % 100 != 0 || year % 400 == 0;
}
void show()const;
int operator - (const Date & date)const {
return totalDays - date.totalDays;
}
};
#include "account.h"
#include <cmath>
#include<iostream>
using namespace std;
double Account::total = 0;
Account::Account(const Date & date, const string & id) :id(id), balance(0) {
date.show();
cout << "\t#" << id << "created" << endl;
}
void Account::record(const Date & date, double amount, const string & desc) {
amount = floor(amount * 100 + 0.5) / 100;
balance += amount;
total += amount;
date.show();
cout << "\t#" << id << "\t" << amount << "\t" << balance << "\t" << desc << endl;
}
void Account::show()const { cout << id << "\tBalance:" << balance; }
void Account::error(const string & msg)const {
cout << "Error(#" << id << "):" << msg << endl;
}
SavingsAccount::SavingsAccount(const Date & date, const string & id, double rate) :Account(date, id), rate(rate), acc(date, 0) {}
void SavingsAccount::deposit(const Date & date, double amount, const string & desc) {
record(date, amount, desc);
acc.change(date, getBalance());
}
void SavingsAccount::withdraw(const Date & date, double amount, const string & desc) {
if (amount > getBalance()) {
error("not enough money");
}
else {
record(date, -amount, desc);
acc.change(date, getBalance());
}
}
void SavingsAccount::settle(const Date & date) {
if (date.getMonth() == 1) {
double interest = acc.getSum(date) * rate / (date - Date(date.getYear() - 1, 1, 1));
if (interest != 0) record(date, interest, "interest");
acc.reset(date, getBalance());
}
}
CreditAccount::CreditAccount(const Date & date, const string & id, double credit, double rate, double fee) :Account(date, id), credit(credit), rate(rate), fee(fee), acc(date, 0) {}
void CreditAccount::deposit(const Date & date, double amount, const string & desc) {
record(date, amount, desc);
acc.change(date, getDebt());
}
void CreditAccount::withdraw(const Date & date, double amount, const string & desc) {
if (amount - getBalance() > credit) {
error("not enouogh credit");
}
else {
record(date, -amount, desc);
acc.change(date, getDebt());
}
}
void CreditAccount::settle(const Date & date) {
double interest = acc.getSum(date) * rate;
if (interest != 0) record(date, interest, "interest");
if (date.getMonth() == 1)record(date, -fee, "annual fee");
acc.reset(date, getDebt());
}
void CreditAccount::show()const {
Account::show();
cout << "\tAvailable credit:" << getAvailableCredit();
}
#include"account.h"
#include<iostream>
using namespace std;
int main() {
Date date(2008, 11, 1);
SavingsAccount sa1(date, "S3755217", 0.015);
SavingsAccount sa2(date, "02342342", 0.015);
CreditAccount ca(date, "C5392394", 10000, 0.0005, 50);
Account * accounts[] = { &sa1,&sa2,&ca };
const int n = sizeof(accounts) / sizeof(Account*);
cout << "(d)deposit (w)withdraw (s)show (c)change day (n)next month (e)exit" << endl;
char cmd;
do {
date.show();
cout << "\tTotal:" << Account::getTotal() << "\tcommand>";
int index, day;
double amount;
string desc;
cin >> cmd;
switch (cmd) {
case 'd':
cin >> index >> amount;
getline(cin, desc);
accounts[index]->deposit(date, amount, desc);
break;
case 'w':
cin >> index >> amount;
getline(cin, desc);
accounts[index]->withdraw(date, amount, desc);
break;
case 's':
for (int i = 0; i < n; i++) {
cout << "[" << i << "]";
accounts[i]->show();
cout << endl;
}
break;
case 'c':
cin >> day;
if (day < date.getDay()) {
cout << "You cannot specify a previous day";
}
else if (day > date.getMaxDay())
cout << "Invalid day";
else date = Date(date.getYear(), date.getMonth(), day);
break;
case 'n':
if (date.getMonth() == 12)
date = Date(date.getYear() + 1, 1, 1);
else date = Date(date.getYear(), date.getMonth() + 1, 1);
for (int i = 0; i < n; i++) {
accounts[i]->settle(date);
}
break;
}
} while (cmd != 'e');
return 0;
}
#include"date.h"
#include<iostream>
#include<cstdlib>
using namespace std;
namespace {
const int DAYS_BEFIRE_MONTH[] = { 0,31,59,90,120,151,181,212,243,273,304,334,365 };
}
Date::Date(int year, int month, int day) :year(year), month(month), day(day) {
if (day <= 0 || day > getMaxDay()) {
cout << "Invalid date:";
show();
cout << endl;
exit(1);
}
int years = year - 1;
totalDays = years * 365 + year / 4 - years / 100 + years / 400 + DAYS_BEFIRE_MONTH[month - 1] + day;
if (isLeapYear() && month > 2)totalDays++;
}
int Date::getMaxDay()const {
if (isLeapYear() && month == 2)
return 29;
else return DAYS_BEFIRE_MONTH[month] - DAYS_BEFIRE_MONTH[month - 1];
}
void Date::show()const {
cout << getYear() << "-" << getMonth() << "-" << getDay();
}
思考:我们首先回顾一下7-10的调整,通过类的继承的方式无需重复写相同的类的成员,但是由于派生类和基类的本质上依旧是独立的,成员函数可以通过作用域运算符“继承”基类的成员函数,但是无法通过指向基类的指针或引用类型的数组操控所有类。在8-7的实例中通过虚函数,使得相同的函数在不同类的对象中进行动态绑定,实现基类函数的覆盖,同时可以通过一组相同类型的指针或引用类型对不同基类和派生类进行一系列的相同操作。