廖雪峰Java9正则表达式-1正则表达式入门-1正则表达式简介
1.使用代码来判断字符串
场景:
- 判断字符串是否是有效的电话号码:"010-12345678", "123ABC456"
- 判断字符串是否是有效的电子邮箱地址:"test@example.com", "test#example"
- 判断字符串是否是有效的时间:"12:34", "99:99"
通过程序判断需要为每种判断创建规则,然后用代码实现:
示例1:判断手机号
Phone.java
package com.testList;
public class Phone{
public boolean isVaildMobile(String s){
if (s.length() != 11){
return false;
}
for(int i=0;i<s.length();i++){
char c = s.charAt(i);
if ( c<'0' || c > '9'){
return false;
}
}
return true;
}
}
PhoneTest.java
package com.testList;
import org.junit.After;
import org.junit.Before;
import org.junit.Test;
import static org.junit.Assert.*;
public class PhoneTest {
Phone phone;
@Before
public void setUp(){
phone = new Phone();
}
@Test
public void isVaildMobile() {
System.out.println(phone.isVaildMobile("13311112222"));
}
@After
public void tearDown(){
phone = null;
}
}
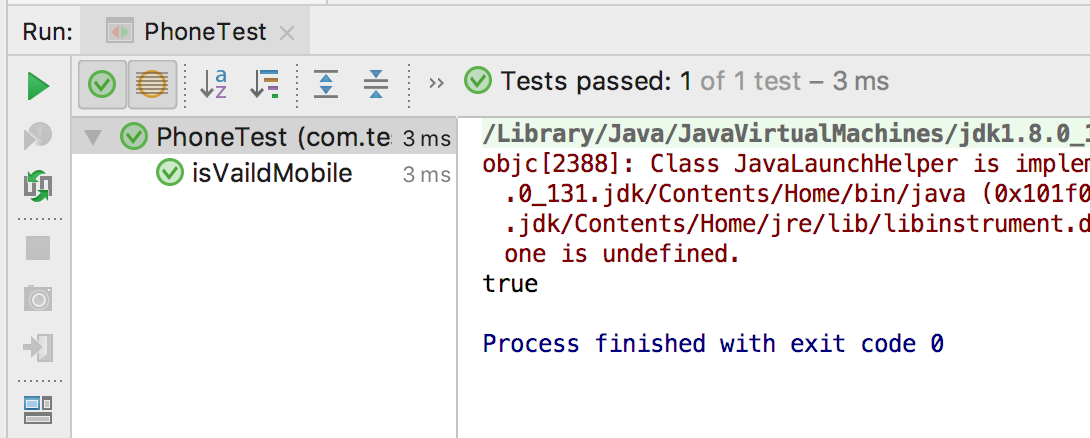
2.正则表达式的优势
如果用代码来实现各种判断,就要为每种判断创建规则,如判断邮箱、日期、时间,会相当麻烦。
正则表达式可以用字符串来描述规则,并用来匹配字符串。如上例中手机号的校验
package com.testList;
public class Phone{
public boolean isVaildMobile(String s){
String rule = "\\d{11}";
return s.matches(rule);
}
}
使用正则表达式的好处:
- 一个正则表达式就是一个描述规则的字符串
- 只需要编写正确的规则,就可以让正则表达式引擎去判断目标字符串是否符合规则
- 正则表达式是一套标准,可以用于任何语言
- JDK内置正则表达式引擎:java.util.regex
Phone.java
package com.testList;
public class Phone{
public static boolean isCentury20th(String s){
if(s == null){
return false;
}
String rule = "19\\d\\d";
return s.matches(rule);
}
}
PhoneTest.java
package com.testList;
import org.junit.After;
import org.junit.Before;
import org.junit.Test;
import static org.junit.Assert.*;
public class PhoneTest {
@Test
public void testIsCentury20th(){
assertTrue(Phone.isCentury20th("1900"));
assertTrue(Phone.isCentury20th("1901"));
assertTrue(Phone.isCentury20th("1922"));
assertTrue(Phone.isCentury20th("1948"));
assertTrue(Phone.isCentury20th("1901"));
assertTrue(Phone.isCentury20th("1999"));
assertFalse(Phone.isCentury20th(null));
assertFalse(Phone.isCentury20th(""));
assertFalse(Phone.isCentury20th(" "));
assertFalse(Phone.isCentury20th("19"));
assertFalse(Phone.isCentury20th("190"));
assertFalse(Phone.isCentury20th("190A"));
assertFalse(Phone.isCentury20th("19001"));
assertFalse(Phone.isCentury20th("1900s"));
assertFalse(Phone.isCentury20th("2900"));
assertFalse(Phone.isCentury20th("A900"));
}
}
3.总结:
正则表达式是一个字符串
正则表达式用字符串描述一个匹配规则
使用正则表达式可以快速判断给定的字符串是否符合匹配规则
Java内建正则表达式java.util.regex