- 数组定义中的类型名可以是内置数据类型或类类型;除引用之外,数组元素的类型还可以是任意的复合类型。没有所有元素都是引用的数组。
- 与vector不同,一个数组不能用另外一个数组初始化,也不能将一个数组赋值给另一个数组,这些操作都是非法的:
int ia[] = {0, 1, 2}; // ok: array of ints int ia2[](ia); // error: cannot initialize one array with another int main() { const unsigned array_size = 3; int ia3[array_size]; // ok: but elements are uninitialized! ia3 = ia; // error: cannot assign one array to another return 0; }
- 一个有效的指针必然是以下三种状态之一:保存一个特定对象的地址;指向某个对象后面的另一对象;或者是0值。若指针保存0值,表明它不指向任何对象。未初始化的指针是无效的,直到给该指针赋值后,才可使用它。下列定义和赋值都是合法的:
int ival = 1024; int *pi = 0; // pi initialized to address no object int *pi2 = & ival; // pi2 initialized to address of ival int *pi3; // ok, but dangerous, pi3 is uninitialized pi = pi2; // pi and pi2 address the same object, e.g. ival pi2 = 0; // pi2 now addresses no object
- 对指针进行初始化或赋值只能使用以下四种类型的值:
- 0 值常量表达式(第 2.7 节),例如,在编译时可获得 0 值的整型 const 对象或字面值常量 0。
- 类型匹配的对象的地址。
- 另一对象末的下一地址。
- 同类型的另一个有效指针。
- 如果没有显式提供元素初值,则数组元素会像普通变量一样初始化(第 2.3.4 节):
在函数体外定义的内置数组,其元素均初始化为 0。
在函数体内定义的内置数组,其元素无初始化。
不管数组在哪里定义,如果其元素为类类型,则自动调用该类的默认构造函数进行初始化;如果该类没有默认构造函数,则必须为该数组的元素提供显式初始化。 - 阅读 const 声明语句产生的部分问题,源于 const 限定符既可以放在类型前也可以放在类型后:
string const s1; // s1 and s2 have same type, const string s2; // they're both strings that are const
用 typedef 写 const 类型定义时,const 限定符加在类型名前面容易引起对所定义的真正类型的误解:
string s; typedef string *pstring; const pstring cstr1 = &s; // written this way the type is obscured pstring const cstr2 = &s; // all three decreations are the same type string *const cstr3 = &s; // they're all const pointers to string
把 const 放在类型 pstring 之后,然后从右向左阅读该声明语句就会非常清楚地知道 cstr2 是 const pstring 类型,即指向 string 对象的 const 指针。
不幸的是,大多数人在阅读 C++ 程序时都习惯看到 const 放在类型前面。于是为了遵照惯例,只好建议编程时把 const 放在类型前面。但是,把声明语句重写为置 const 于类型之后更便于理解。
- 动态分配数组时,如果数组元素具有类类型,将使用该类的默认构造函数(第 2.3.4 节)实现初始化;如果数组元素是内置类型,则无初始化:
- 也可使用跟在数组长度后面的一对空圆括号,对数组元素做值初始化(第 3.3.1 节):
int *pia2 = new int[10] (); // array of 10 uninitialized ints
圆括号要求编译器对数组做值初始化,在本例中即把数组元素都设置为0。
- 对于动态分配的数组,其元素只能初始化为元素类型的默认值,而不能像数组变量一样,用初始化列表为数组元素提供各不相同的初值。
- 如果我们在自由存储区中创建的数组存储了内置类型的 const 对象,则必须为这个数组提供初始化:因为数组元素都是 const 对象,无法赋值。实现这个要求的唯一方法是对数组做值初始化:
// error: uninitialized const array const int *pci_bad = new const int[100]; // ok: value-initialized const array const int *pci_ok = new const int[100]();
- c_str 返回的数组并不保证一定是有效的,接下来对 st2 的操作有可能会改变 st2 的值,使刚才返回的数组失效。如果程序需要持续访问该数据,则应该复制 c_str 函数返回的数组。
-
int *ip[4]; // array of pointers to int int (*ip)[4]; // pointer to an array of 4 ints
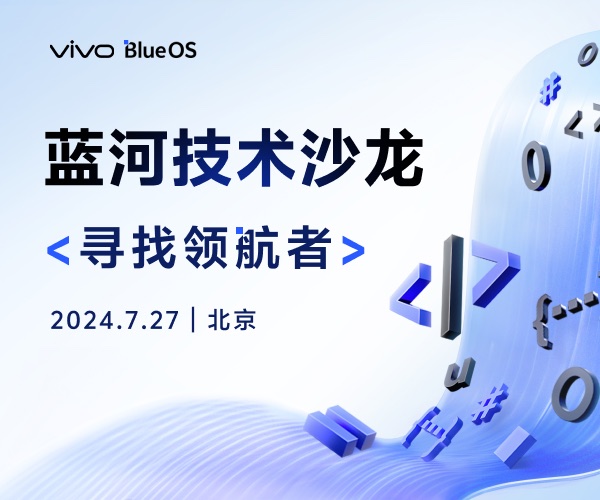