贪心放灯
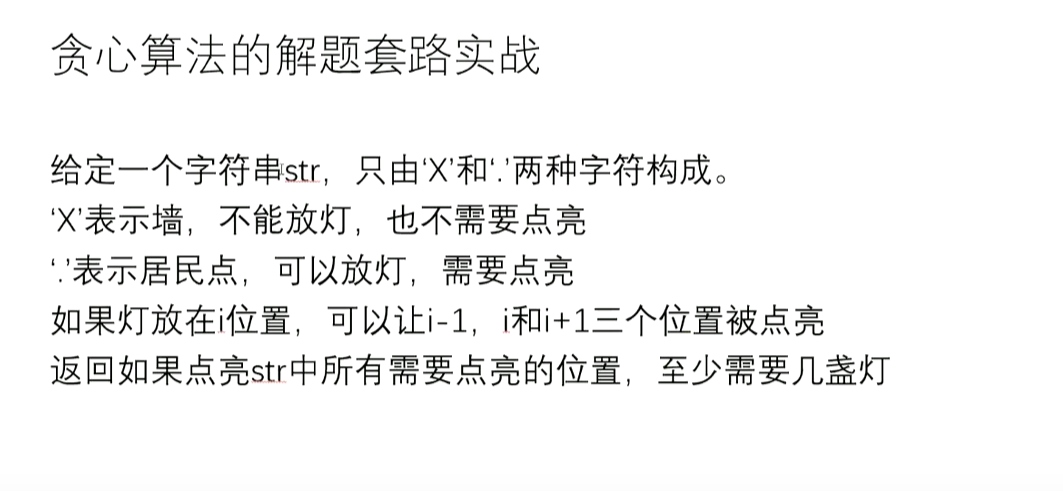
暴力递归:
1 #include <bits/stdc++.h> 2 3 using namespace std; 4 5 class Solution { 6 public: 7 //定义:index之后的位置选择还没做,前面的已经做了,light存放已经放过灯的位置; 8 //返回能够照亮所有位置的最小的灯的使用情况 9 int process(int index,string & s,unordered_set<int> light){ 10 //所有的位置都已经选择完了 11 if(index == s.size()){ 12 //查找一遍,看付不付合是所有的位置都照亮了 13 for (int i = 0; i < s.size(); ++i) { 14 if(s[i] == '.'){ 15 if(((light.find(i) == light.end()) && 16 (light.find(i+1) == light.end())&& 17 (light.find(i-1) == light.end()))) 18 return INT_MAX; 19 } 20 } 21 return light.size(); 22 } 23 //还有选择 24 else{ 25 //不管是墙还是屋子,都不给放灯 26 int no = process(index+1,s,light); 27 28 //如果是屋子,选择放灯 29 int yes = INT_MAX; 30 if(s[index] == '.'){ 31 light.insert(index); 32 yes = process(index+1,s,light); 33 light.erase(index); 34 } 35 return min(no,yes); 36 } 37 38 } 39 40 }; 41 42 int main() { 43 // 44 Solution a; 45 string ss = {"X...XX."}; 46 unordered_set<int> set1; 47 set1.clear(); 48 int b = a.process(0,ss,set1); 49 return 0; 50 }
贪心:
1 #include <bits/stdc++.h> 2 3 using namespace std; 4 5 class Solution { 6 public: 7 int minlight(string s) { 8 int index = 0; 9 int light = 0; 10 while (index < s.size()) { 11 if (s[index] == 'X') index++; 12 else { 13 //放一盏灯 14 light++; 15 //最后一个位置是房子,必然要放一盏灯; 16 if (index + 1 == s.size()) break; 17 else { 18 //灯放index 19 if (s[index + 1] == 'X') index = index + 2; 20 //灯放index+1位置; 21 else index = index + 3; 22 } 23 } 24 } 25 return light; 26 } 27 };