一.代码
1.1.前言:
1.双链表与单链表有许多相似之处,区别在于插入与删除。所以只选取不同点的代码。
2.为了简化操作,将头节点的数据部分存储链表的长度,此时,就不必遍历链表了。
#include<stdio.h>
#include<stdlib.h>
#define false 0
#define true 1
typedef struct node {
int data;
struct node* next;
struct node* prior;
}DLinkList;
DLinkList* init(DLinkList* List) {
List = (DLinkList*)malloc(sizeof(DLinkList));
if (List) {
List->data = 0;//元素数量
List->next = NULL;
List->prior = NULL;
return List;
}
printf("fail to allocate menory\n");
return List;
}
int listInsert(DLinkList* List, int pos, int value) {
if (pos < 1) {
printf("abnormal position\n");
return false;
}
if (List) {
DLinkList* temp = List;
int position = 0;
while (temp->next != NULL && position < pos - 1) {
position++;
temp = temp->next;
}
if (position == pos - 1) {
DLinkList* new_node = (DLinkList*)malloc(sizeof(DLinkList));
if (new_node) {
new_node->data = value;
if (temp->next != NULL)
temp->next->prior = new_node;
new_node->next = temp->next;
temp->next = new_node;
new_node->prior = temp;
List->data++;
return true;
}
printf("fail to allocate menory\n");
return false;
}
printf("abnormal position\n");
return false;
}
printf("the list doesn't exist\n");
return false;
}
void print(DLinkList* List) {
if (List) {
DLinkList* temp = List;
printf("ascending order:");
while (temp->next!=NULL) {
printf("%d ", temp->next->data);
temp = temp->next;
}
printf("\n");
printf("descending order:");
while (temp->prior != NULL) {
printf("%d ", temp->data);
temp = temp->prior;
}
printf("\n");
return;
}
printf("the list doesn't exist\n");
}
int listDelete(DLinkList* List,int pos,int* value) {
if (pos < 1) {
printf("abnormal position\n");
return false;
}
if (List) {
DLinkList* temp = List;
int position = 0;
while (temp->next != NULL && position < pos - 1) {
position++;
temp = temp->next;
}
if (temp->next != NULL && pos - 1 == position) {
*value = temp->next->data;
DLinkList* deletde_node=temp->next;
if (temp->next->next != NULL)
temp->next->next->prior = temp;
temp->next = temp->next->next;
List->data--;
free(deletde_node);
return true;
}
printf("abnormal position\n");
return false;
}
printf("the list dosen't exist\n");
return false;
}
int length(DLinkList* List) {
return List->data;
}
int main() {
DLinkList* List = NULL;
List = init(List);
if (List) {
int value;
listInsert(List, 1, 1);
listInsert(List, 1, 2);
listInsert(List, 1, 3);
listInsert(List, 1, 4);
listInsert(List, 1, 5);
printf("the length of List:%d\n",length(List));
print(List);
if (listDelete(List, 5, &value))
printf("deleted data:%d\n", value);
printf("the length of List:%d\n", length(List));
print(List);
}
return 0;
}
二.运行结果
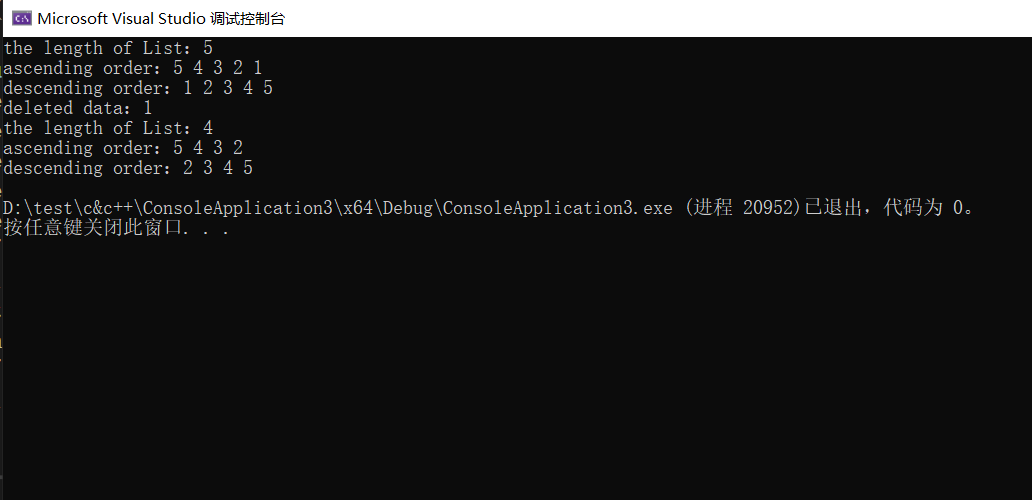
三.遇到的问题
3.1.插入元素时需要考虑下一个元素是否存在,因为需要改变该元素的前驱指针
3.2.删除元素时同理。