一.代码
#include <stdio.h>
#include<stdlib.h>
#define false 0
#define true 1
typedef struct {
int *data;
int length, maxSize;
}SqList;
SqList* init(SqList* List) {
List = (SqList*)malloc(sizeof(SqList));
if (List) {
List->maxSize = 100;
List->data = (int*)malloc(sizeof(int) * List->maxSize);
List->length = 0;
}
else
printf("fail to allocate menory\n");
return List;
}
int listInsert(SqList* List,int pos,int value) {
if (List->length == List->maxSize) {
printf("the list is full\n");
return false;
}
if (pos<1 || pos>List->length + 1) {
printf("abnormal position\n");
return false;
}
for (int i = List->length; i >= pos; i--)
List->data[i] = List->data[i - 1];
List->data[pos - 1] = value;
List->length++;
return true;
}
int listDelete(SqList* List, int pos,int* value) {
if (List->length < 1) {
printf("the List is empty\n");
return false;
}
if (pos<1 || pos>List->length) {
printf("abnormal position\n");
return false;
}
*value = List->data[pos - 1];
for (int i = pos - 1; i < List->length - 1; i++)
List->data[i] = List->data[i + 1];
List->length--;
return true;
}
int reallocate(SqList* List,int size) {
if (List) {
if (int* temp = (int*)realloc(List->data, sizeof(int) * size)) {
List->data = temp;
List->maxSize = size;
}
else {
printf("fail to reallocate menory\n");
return false;
}
}
else {
printf("fail to reallocate menory\n");
return false;
}
return true;
}
int length(SqList* List) {
return List->length;
}
int locateElem(SqList* List,int *pos,int value) {
if (List->length < 1) {
printf("the List is empty\n");
return false;
}
for (int i = 0; i < List->length; i++)
if (List->data[i] == value) {
*pos = i + 1;
return true;
}
printf("not found\n");
return false;
}
int getElem(SqList* List, int pos,int* value) {
if (List->length < 1) {
printf("teh List is empty\n");
return false;
}
if (pos<1 || pos>List->length) {
printf("abnormal position\n");
return false;
}
*value = List->data[pos-1];
return true;
}
void Print(SqList* List) {
if (List) {
printf("data:");
for (int i = 0; i < List->length; i++)
printf("%d ", List->data[i]);
printf("\n");
return;
}
printf("the list doesn't exist");
}
int Empty(SqList* List) {
if (List->length == 0)
return true;
return false;
}
SqList* destroy(SqList* List) {
free(List);
return NULL;
}
int main() {
SqList* List = NULL;
//初始化
List = init(List);
if (List) {
int pos;
int value;
//插入数据
listInsert(List, 1, 1);
listInsert(List, 1, 2);
listInsert(List, 1, 3);
listInsert(List, 1, 4);
listInsert(List, 1, 5);
listInsert(List, 1, 6);
//打印
Print(List);
//查看顺序表长度
printf("length = %d\n", length(List));
listDelete(List, 1, &value);
Print(List);
printf("length = %d\n", length(List));
//查看目标值在顺序表中的位置
if (locateElem(List, &pos, 6))
printf("position = %d\n", pos);
//为顺序表扩容
if (reallocate(List, 200)) {
printf("maxSize = %d\n", List->maxSize);
printf("size of data = %d\n", _msize(List->data) / sizeof(int));
}
getElem(List, 1, &value);
printf("value = %d\n", value);
if (Empty(List)) {
printf("the list is empty\n");
}
if (!(List = destroy(List)))
printf("the list has been detroyed\n");
}
return 0;
}
二.顺序表的重要操作
1.插入
2.删除
3.按值查找
2.1.插入操作
平均次数:n/2
2.2.删除操作
平均次数:n-1/2
2.3.按值查找
平均次数:n+1/2
三.运行结果
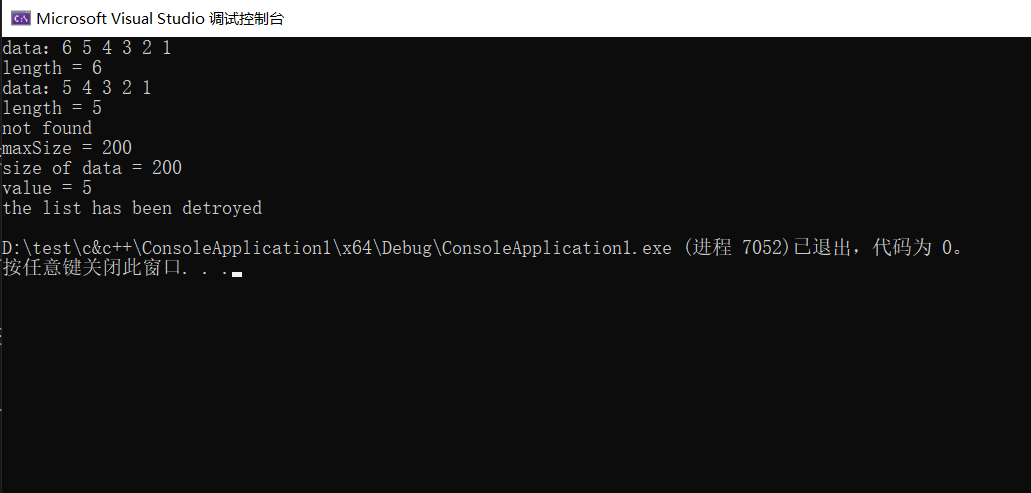