(Easy)1071. Greatest Common Divisor of Strings- LeetCode
Description:
For strings S
and T
, we say "T
divides S
" if and only if S = T + ... + T
(T
concatenated with itself 1 or more times)
Return the largest string X
such that X
divides str1 and X
divides str2.
Example 1:
Input: str1 = "ABCABC", str2 = "ABC"
Output: "ABC"
Example 2:
Input: str1 = "ABABAB", str2 = "ABAB"
Output: "AB"
Example 3:
Input: str1 = "LEET", str2 = "CODE"
Output: ""
Note:
1 <= str1.length <= 1000
1 <= str2.length <= 1000
str1[i]
andstr2[i]
are English uppercase letters.
Accepted
12,036
Submissions
22,171
Solution:
First Attemp: Time Limit Exceeded.
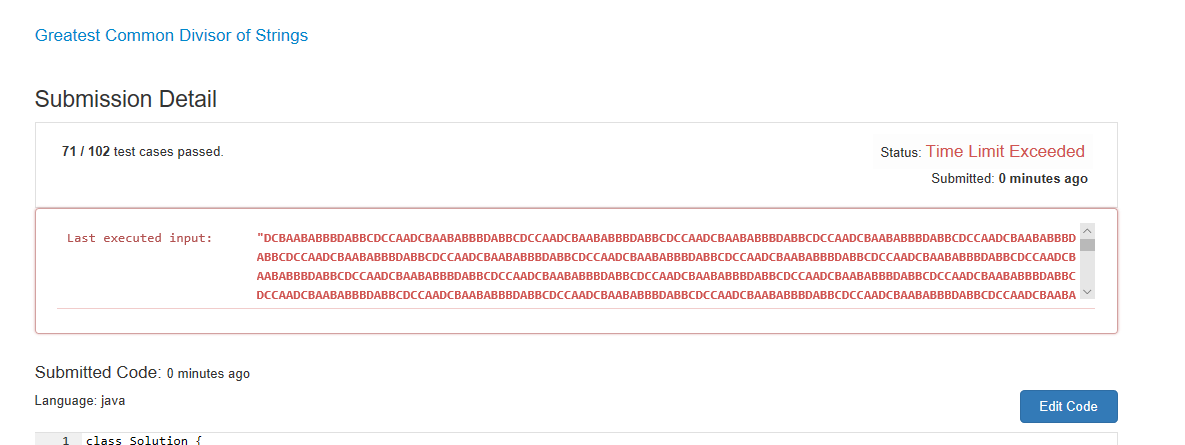
class Solution { public String gcdOfStrings(String str1, String str2) { if(str1 ==null|| str2==null){ return ""; } int max = Integer.MIN_VALUE; String res=""; for(int i = 0; i< str2.length(); i++){ for(int j = i; j<str2.length(); j++){ //System.out.println(str2.substring(i,j+1)); String tmp = str2.substring(i,j+1); if(Find(str1,tmp)&&Find(str2, tmp)){ if(tmp.length()>max){ max = tmp.length(); res = tmp; } } } } return res; } static boolean Find(String s1, String s2){ int a = s1.length(); int b = s2.length(); int times = a/b; String s =""; if(s1.length()%s2.length()!=0){ return false; } else{ for(int i = 0; i<times; i++){ s = s+ s2; } } if(s1.equals(s)){ return true; } else{ return false; } } }
Second Attempt:
class Solution { public String gcdOfStrings(String str1, String str2) { if(str1 ==null|| str2==null){ return ""; } int max = Integer.MIN_VALUE; String res=""; for(int i = 0; i< str2.length(); i++){ //System.out.println(str2.substring(0,i+1)); String tmp = str2.substring(0,i+1); if(Find(str1,tmp)&&Find(str2, tmp)){ if(tmp.length()>max){ max = tmp.length(); res = tmp; } } } return res; } static boolean Find(String s1, String s2){ int a = s1.length(); int b = s2.length(); int times = a/b; String s =""; if(s1.length()%s2.length()!=0){ return false; } else{ for(int i = 0; i<times; i++){ s = s+ s2; } } if(s1.equals(s)){ return true; } else{ return false; } } }