How2j学习记录 图形界面
知识点1:图形界面 Hello Swing
1.简单的例子
JFrame是GUI中的容器 JButton是最常见的组件--按钮
setVisible(true);会对所有的组件进行渲染,一定要放在最后面
package gui; import javax.swing.JButton; import javax.swing.JFrame; public class TestGUI { public static void main(String[] args) { // 主窗体 JFrame f = new JFrame("LOL"); // 主窗体设置大小 f.setSize(400, 300); // 主窗体设置位置 f.setLocation(200, 300); // 主窗体中的组件设置为绝对定位 f.setLayout(null); // 按钮组件 JButton b = new JButton("一键秒对方基地挂"); // 同时设置组件的大小和位置 b.setBounds(50, 50, 280, 30); // 把按钮加入到主窗体中 f.add(b); // 关闭窗体的时候,退出程序 f.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE); // 让窗体变得可见 f.setVisible(true); } }
知识点2 事件监听
1.按钮监听
创建一个匿名类实现ActionListener接口,当按钮被点击时,actionPerformed方法就会被调用
package gui; import java.awt.event.ActionEvent; import java.awt.event.ActionListener; import javax.swing.ImageIcon; import javax.swing.JButton; import javax.swing.JFrame; import javax.swing.JLabel; public class TestGUI { public static void main(String[] args) { // 主窗体 JFrame f = new JFrame("LOL"); // 主窗体设置大小 f.setSize(400, 300); // 主窗体设置位置 f.setLocation(580, 200); // 主窗体中的组件设置为绝对定位 f.setLayout(null); final JLabel l = new JLabel(); ImageIcon i = new ImageIcon("D:/project/j2se/src/gui/shana.png"); l.setIcon(i); l.setBounds(50, 50, i.getIconWidth(), i.getIconHeight()); JButton b = new JButton("隐藏图片"); b.setBounds(150, 200, 100, 30); b.addActionListener(new ActionListener() { public void actionPerformed(ActionEvent e) { l.setVisible(false); } }); f.add(l); f.add(b); f.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE); f.setVisible(true); } }
2.键盘监听
KeyListener
keyPressed:代表键盘按下
keyReleased:代表键盘弹起
keyTyped:代表一个按下弹起的组合动作
KeyEvent.getKeyCode():可以获取当前点下了哪个键
package gui; import java.awt.event.KeyEvent; import java.awt.event.KeyListener; import javax.swing.ImageIcon; import javax.swing.JFrame; import javax.swing.JLabel; public class TestGUI { public static void main(String[] args) { // 主窗体 JFrame f = new JFrame("LOL"); f.setSize(400, 300); f.setLocation(580, 200); f.setLayout(null); final JLabel l = new JLabel(); ImageIcon i = new ImageIcon("D:/project/j2se/src/gui/shana.png"); l.setIcon(i); l.setBounds(50, 50, i.getIconWidth(), i.getIconHeight()); // 增加键盘监听 f.addKeyListener(new KeyListener() { // 键盘弹起 public void keyReleased(KeyEvent e) { if (e.getKeyCode() == 39) { // 按下了右键 l.setLocation(l.getX() + 10, l.getY()); } } public void keyPressed(KeyEvent e) { } public void keyTyped(KeyEvent e) { } }); f.add(l); f.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE); f.setVisible(true); } }
3.鼠标监听
package gui; import java.awt.event.MouseEvent; import java.awt.event.MouseListener; import java.util.Random; import javax.swing.ImageIcon; import javax.swing.JFrame; import javax.swing.JLabel; public class TestGUI { public static void main(String[] args) { // 主窗体 final JFrame f = new JFrame("LOL"); f.setSize(400, 600); f.setLocationRelativeTo(null); f.setLayout(null); final JLabel l = new JLabel(); ImageIcon i = new ImageIcon("D:/project/j2se/src/gui/shana_heiheihei.png"); l.setIcon(i); l.setBounds(375, 275, i.getIconWidth(), i.getIconHeight()); f.add(l); l.addMouseListener(new MouseListener() { @Override public void mouseClicked(MouseEvent e) { // TODO Auto-generated method stub } @Override public void mousePressed(MouseEvent e) { // TODO Auto-generated method stub } @Override public void mouseReleased(MouseEvent e) { // TODO Auto-generated method stub } @Override public void mouseEntered(MouseEvent e) { Random r = new Random(); int x = r.nextInt(f.getWidth() - l.getWidth()); int y = r.nextInt(f.getHeight() - l.getHeight()); l.setLocation(x, y); } @Override public void mouseExited(MouseEvent e) { // TODO Auto-generated method stub } }); f.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE); f.setVisible(true); } }
4.适配器
MouseAdapter 鼠标监听适配器
一般说来在写监听器的时候,会实现MouseListener。
但是MouseListener里面有很多方法实际上都没有用到,比如mouseReleased ,mousePressed,mouseExited等等。
这个时候就可以使用 鼠标监听适配器,MouseAdapter 只需要重写必要的方法即可。
package gui; import java.awt.event.MouseAdapter; import java.awt.event.MouseEvent; import java.util.Random; import javax.swing.ImageIcon; import javax.swing.JFrame; import javax.swing.JLabel; public class TestGUI { public static void main(String[] args) { // 主窗体 final JFrame f = new JFrame("LOL"); f.setSize(800, 600); f.setLocationRelativeTo(null); f.setLayout(null); final JLabel l = new JLabel(); ImageIcon i = new ImageIcon("D:/project/j2se/src/gui/shana_heiheihei.png"); l.setIcon(i); l.setBounds(375, 275, i.getIconWidth(), i.getIconHeight()); f.add(l); l.addMouseListener(new MouseAdapter() { public void mouseEntered(MouseEvent e) { Random r = new Random(); int x = r.nextInt(f.getWidth() - l.getWidth()); int y = r.nextInt(f.getHeight() - l.getHeight()); l.setLocation(x, y); } }); f.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE); f.setVisible(true); } }
知识点3 容器
java的图形界面中,容器是用来存放 按钮,输入框等组件的。
窗体型容器有两个,一个是JFrame,一个是JDialog
1.JFrame是最常用的窗体型容器,默认情况下,在右上角有最大化最小化按钮
package gui; import javax.swing.JButton; import javax.swing.JFrame; public class TestGUI { public static void main(String[] args) { JFrame f = new JFrame("LOL"); f.setSize(400, 300); f.setLocation(200, 200); f.setLayout(null); JButton b = new JButton("一键秒对方基地挂"); b.setBounds(50, 50, 200, 30); f.add(b); f.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE); f.setVisible(true); } }
2.JDialog
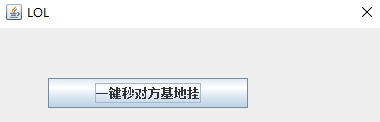
package gui; import javax.swing.JButton; import javax.swing.JDialog; public class TestGUI { public static void main(String[] args) { JDialog d = new JDialog(); d.setTitle("LOL"); d.setSize(400, 300); d.setLocation(200, 200); d.setLayout(null); JButton b = new JButton("一键秒对方基地挂"); b.setBounds(50, 50, 200, 30); d.add(b); d.setVisible(true); } }
3.模态JDialog
当一个对话框被设置为模态的时候,其背后的父窗体,是不能被激活的,除非该对话框被关闭
package gui; import javax.swing.JButton; import javax.swing.JDialog; import javax.swing.JFrame; public class TestGUI { public static void main(String[] args) { JFrame f = new JFrame("外部窗体"); f.setSize(800, 600); f.setLocation(100, 100); JDialog d = new JDialog(); // 设置为模态 d.setModal(true); d.setTitle("模态的对话框"); d.setSize(400, 300); d.setLocation(200, 200); d.setLayout(null); JButton b = new JButton("一键秒对方基地挂"); b.setBounds(50, 50, 280, 30); d.add(b); f.setVisible(true); d.setVisible(true); } }
4.窗体大小不可变化
通过调用方法 setResizable(false); 做到窗体大小不可变化
package gui; import javax.swing.JButton; import javax.swing.JFrame; public class TestGUI { public static void main(String[] args) { JFrame f = new JFrame("LoL"); f.setSize(400, 300); f.setLocation(200, 200); f.setLayout(null); JButton b = new JButton("一键秒对方基地挂"); b.setBounds(50, 50, 280, 30); f.add(b); // 窗体大小不可变化 f.setResizable(false); f.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE); f.setVisible(true); } }
知识点5 组件
1.标签
package gui; import java.awt.Color; import javax.swing.JFrame; import javax.swing.JLabel; public class TestGUI { public static void main(String[] args) { JFrame f = new JFrame("LoL"); f.setSize(400, 300); f.setLocation(200, 200); f.setLayout(null); JLabel l = new JLabel("LOL文字"); l.setForeground(Color.red); l.setBounds(50, 50, 280, 30); f.add(l); f.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE); f.setVisible(true); } }
2.使用JLabel显示图片
package gui; import javax.swing.ImageIcon; import javax.swing.JFrame; import javax.swing.JLabel; public class TestGUI { public static void main(String[] args) { JFrame f = new JFrame("LoL"); f.setSize(400, 300); f.setLocation(580, 200); f.setLayout(null); JLabel l = new JLabel("LOL文字"); ImageIcon i = new ImageIcon("D:/project/j2se/src/gui/shana.png"); l.setIcon(i); l.setBounds(50, 50, i.getIconWidth(), i.getIconHeight()); f.add(l); f.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE); f.setVisible(true); } }
3.按钮
package gui; import javax.swing.JButton; import javax.swing.JFrame; public class TestGUI { public static void main(String[] args) { JFrame f = new JFrame("LoL"); f.setSize(400, 300); f.setLocation(580, 200); f.setLayout(null); JButton b = new JButton("一键秒对方基地挂"); b.setBounds(50, 50, 280, 30); f.add(b); f.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE); f.setVisible(true); } }
4.复选框
JCheckBox复选框
使用isSelected来获取是否选中了
package gui; import javax.swing.JCheckBox; import javax.swing.JFrame; public class TestGUI { public static void main(String[] args) { JFrame f = new JFrame("LoL"); f.setSize(400, 300); f.setLocation(580, 200); f.setLayout(null); JCheckBox bCheckBox = new JCheckBox("物理英雄"); bCheckBox.setSelected(true); bCheckBox.setBounds(50, 50, 130, 30); JCheckBox bCheckBox2 = new JCheckBox("魔法英雄"); bCheckBox2.setBounds(50, 100, 130, 30); System.out.println(bCheckBox2.isSelected()); f.add(bCheckBox); f.add(bCheckBox2); f.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE); f.setVisible(true); } }
5.单选框
package gui; import javax.swing.JFrame; import javax.swing.JRadioButton; public class TestGUI { public static void main(String[] args) { JFrame f = new JFrame("LoL"); f.setSize(400, 300); f.setLocation(580, 200); f.setLayout(null); JRadioButton b1 = new JRadioButton("物理英雄"); b1.setSelected(true); b1.setBounds(50, 50, 130, 30); JRadioButton b2 = new JRadioButton("魔法英雄"); b2.setBounds(50, 100, 130, 30); System.out.println(b2.isSelected()); f.add(b1); f.add(b2); f.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE); f.setVisible(true); } }
6.按钮组
ButtonGroup 对按钮进行分组,把不同的按钮,放在同一个分组里 ,同一时间,只有一个 按钮 会被选中
package gui; import javax.swing.ButtonGroup; import javax.swing.JFrame; import javax.swing.JRadioButton; public class TestGUI { public static void main(String[] args) { JFrame f = new JFrame("LoL"); f.setSize(400, 300); f.setLocation(580, 200); f.setLayout(null); JRadioButton b1 = new JRadioButton("物理英雄"); b1.setSelected(true); b1.setBounds(50, 50, 130, 30); JRadioButton b2 = new JRadioButton("魔法英雄"); b2.setBounds(50, 100, 130, 30); ButtonGroup bg = new ButtonGroup(); // 把b1,b2放在同一个按钮分组对象里,这样同一时间,只有一个按钮会被选中 bg.add(b1); bg.add(b2); f.add(b1); f.add(b2); f.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE); f.setVisible(true); } }
7.下拉框
package gui; import javax.swing.JComboBox; import javax.swing.JFrame; public class TestGUI { public static void main(String[] args) { JFrame f = new JFrame("LoL"); f.setSize(400, 300); f.setLocation(580, 200); f.setLayout(null); String[] heros = new String[] { "卡特琳娜", "库奇" }; JComboBox cb = new JComboBox(heros); cb.setBounds(50, 50, 80, 30); f.add(cb); f.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE); f.setVisible(true); } }
8.对话框
JOptionPane
JOptionPane.showConfirmDialog(f, "是否 使用外挂 ?");
表示询问,第一个参数是该对话框以哪个组件对齐
JOptionPane.showInputDialog(f, "请输入yes,表明使用外挂后果自负");
接受用户的输入
JOptionPane.showMessageDialog(f, "你使用外挂被抓住! 罚拣肥皂3次!");
显示消息
package gui; import javax.swing.JFrame; import javax.swing.JOptionPane; public class TestGUI { public static void main(String[] args) { JFrame f = new JFrame("LoL"); f.setSize(400, 300); f.setLocation(580, 240); f.setLayout(null); f.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE); f.setVisible(true); int option = JOptionPane.showConfirmDialog(f, "是否使用外挂?"); if (JOptionPane.OK_OPTION == option) { String answer = JOptionPane.showInputDialog(f, "请输入yes,表明使用外挂后果自负"); if ("yes".equals(answer)) { JOptionPane.showMessageDialog(f, "罚捡肥皂3次!"); } } } }
9.文本框
JTextField 输入框
setText 设置文本
getText 获取文本
tfPassword.grabFocus(); 表示让密码输入框获取焦点
package gui; import java.awt.Dimension; import java.awt.FlowLayout; import javax.swing.JFrame; import javax.swing.JLabel; import javax.swing.JTextField; public class TestGUI { public static void main(String[] args) { JFrame f = new JFrame("LoL"); f.setSize(400, 300); f.setLocation(200, 200); f.setLayout(new FlowLayout()); JLabel lName = new JLabel("账号:"); // 输入框 JTextField tfName = new JTextField(""); tfName.setText("请输入账号"); tfName.setPreferredSize(new Dimension(80, 30)); JLabel lPassword = new JLabel("密码:"); // 输入框 JTextField tfPassword = new JTextField(""); tfPassword.setText("请输入密码"); tfPassword.setPreferredSize(new Dimension(80, 30)); f.add(lName); f.add(tfName); f.add(lPassword); f.add(tfPassword); f.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE); f.setVisible(true); tfPassword.grabFocus(); } }
10. 密码框
JPasswordField 密码框
与文本框不同,获取密码框里的内容,推荐使用getPassword,该方法会返回一个字符数组,而非字符串
package gui; import java.awt.Dimension; import java.awt.FlowLayout; import javax.swing.JFrame; import javax.swing.JLabel; import javax.swing.JPasswordField; public class TestGUI { public static void main(String[] args) { JFrame f = new JFrame("LoL"); f.setSize(400, 300); f.setLocation(200, 200); f.setLayout(new FlowLayout()); JLabel l = new JLabel("密码:"); // 密码框 JPasswordField pf = new JPasswordField(""); pf.setText("&48kdh4@#"); pf.setPreferredSize(new Dimension(80, 30)); // 与文本框不同,获取密码框里的内容,推荐使用getPassword,该方法会返回一个字符数组,而非字符串 char[] password = pf.getPassword(); String p = String.valueOf(password); System.out.println(p); f.add(l); f.add(pf); f.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE); f.setVisible(true); } }
11.文本域
JTextArea:文本域。
和文本框JTextField不同的是,文本域可以输入多行数据
如果要给文本域初始文本,通过\n来实现换行效果
JTextArea通常会用到append来进行数据追加
如果文本太长,会跑出去,可以通过setLineWrap(true) 来做到自动换行
package gui; import java.awt.Dimension; import java.awt.FlowLayout; import javax.swing.JFrame; import javax.swing.JLabel; import javax.swing.JTextArea; public class TestGUI { public static void main(String[] args) { JFrame f = new JFrame("LoL"); f.setSize(400, 300); f.setLocation(200, 200); f.setLayout(new FlowLayout()); JLabel l = new JLabel("文本域:"); JTextArea ta = new JTextArea(); ta.setPreferredSize(new Dimension(200, 150)); // \n换行符 ta.setText("抢人头!\n抢你妹啊抢!\n"); // 追加数据 ta.append("我去送了了了了了了了了了了了了了了了了了了了了了了了了"); // 设置自动换行 ta.setLineWrap(true); f.add(l); f.add(ta); f.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE); f.setVisible(true); } }
12.进度条
JProgressBar
package gui; import java.awt.FlowLayout; import javax.swing.JFrame; import javax.swing.JProgressBar; public class TestGUI { public static void main(String[] args) { JFrame f = new JFrame("LoL"); f.setSize(400, 300); f.setLocation(200, 200); f.setLayout(new FlowLayout()); JProgressBar pb = new JProgressBar(); // 进度条最大100 pb.setMaximum(100); // 当前进度是50 pb.setValue(50); // 显示当前进度 pb.setStringPainted(true); f.add(pb); f.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE); f.setVisible(true); } }
知识点7:菜单
1.菜单栏和菜单
package gui; import javax.swing.JFrame; import javax.swing.JMenu; import javax.swing.JMenuBar; public class TestGUI { public static void main(String[] args) { JFrame f = new JFrame("LOL"); f.setSize(400, 300); f.setLocation(200, 200); // 菜单栏 JMenuBar mb = new JMenuBar(); JMenu mHero = new JMenu("英雄"); JMenu mItem = new JMenu("道具"); JMenu mWord = new JMenu("符文"); JMenu mSummon = new JMenu("召唤师"); JMenu mTalent = new JMenu("天赋树"); // 把菜单加入到菜单栏 mb.add(mHero); mb.add(mItem); mb.add(mWord); mb.add(mSummon); mb.add(mTalent); // 把菜单栏加入到frame,这里用的是set而非add f.setJMenuBar(mb); f.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE); f.setVisible(true); } }
2.菜单项
package gui; import javax.swing.JFrame; import javax.swing.JMenu; import javax.swing.JMenuBar; import javax.swing.JMenuItem; public class TestGUI { public static void main(String[] args) { JFrame f = new JFrame("LOL"); f.setSize(400, 300); f.setLocation(200, 200); // 菜单栏 JMenuBar mb = new JMenuBar(); JMenu mHero = new JMenu("英雄"); JMenu mItem = new JMenu("道具"); JMenu mWord = new JMenu("符文"); JMenu mSummon = new JMenu("召唤师"); JMenu mTalent = new JMenu("天赋树"); // 菜单项 mHero.add(new JMenuItem("近战-Warriar")); mHero.add(new JMenuItem("远程-Range")); mHero.add(new JMenuItem("物理-physical")); mHero.add(new JMenuItem("坦克-Tank")); mHero.add(new JMenuItem("法系-Mage")); mHero.add(new JMenuItem("辅助-Support")); mHero.add(new JMenuItem("打野-Jungle")); mHero.add(new JMenuItem("突进-Charge")); mHero.add(new JMenuItem("男性-Boy")); mHero.add(new JMenuItem("女性-Girl")); // 分隔符 mHero.addSeparator(); mHero.add(new JMenuItem("所有-All")); // 把菜单加入到菜单栏 mb.add(mHero); mb.add(mItem); mb.add(mWord); mb.add(mSummon); mb.add(mTalent); // 把菜单栏加入到frame,这里用的是set而非add f.setJMenuBar(mb); f.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE); f.setVisible(true); } }
知识点8:工具栏