LeetCode 51. N-Queens
The n-queens puzzle is the problem of placing n queens on an n x n chessboard such that no two queens attack each other.
Given an integer n, return all distinct solutions to the n-queens puzzle.
Each solution contains a distinct board configuration of the n-queens' placement, where 'Q' and '.' both indicate a queen and an empty space, respectively.
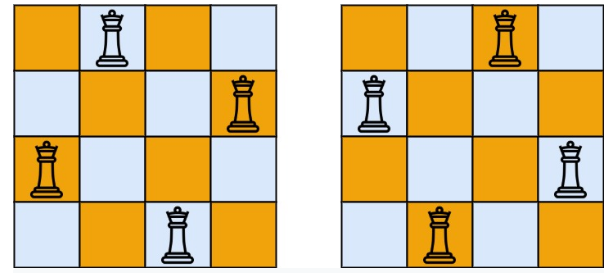
Example 1:
Input: n = 4
Output: [[".Q..","...Q","Q...","..Q."],["..Q.","Q...","...Q",".Q.."]]
Explanation: There exist two distinct solutions to the 4-queens puzzle as shown above
Example 2:
Input: n = 1
Output: [["Q"]]
Constraints:
1 <= n <= 9
实现思路:
不多赘述了,本博客在DFS分类里有三道N皇后问题,总结就是回溯算法。
AC代码:
class Solution {
vector<vector<string>> ans;
vector<string> sq;
int col[100],dg[100],udg[100];
void dfs(int x,int size){
if(x==size){
ans.push_back(sq);
return;
}
for(int i=0;i<size;i++){
if(!col[i]&&!dg[x+i]&&!udg[size-x+i]){
sq[x][i]='Q';//放皇后
col[i]=dg[x+i]=udg[size-x+i]=1;
dfs(x+1,size);
col[i]=dg[x+i]=udg[size-x+i]=0;
sq[x][i]='.';
}
}
}
public:
vector<vector<string>> solveNQueens(int n) {
string tag;
for(int i=0;i<n;i++) tag+='.';
for(int i=0;i<n;i++) sq.push_back(tag);//初始化
cout<<sq.size();
dfs(0,n);
return ans;
}
};