LeetCode 17. Letter Combinations of a Phone Number
Given a string containing digits from 2-9 inclusive, return all possible letter combinations that the number could represent. Return the answer in any order.
A mapping of digit to letters (just like on the telephone buttons) is given below. Note that 1 does not map to any letters.
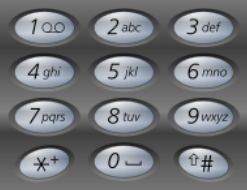
Example 1:
复制Input: digits = "23"
Output: ["ad","ae","af","bd","be","bf","cd","ce","cf"]
Example 2:
Input: digits = ""
Output: []
Example 3:
Input: digits = "2"
Output: ["a","b","c"]
Constraints:
0 <= digits.length <= 4
digits[i] is a digit in the range ['2', '9'].
实现思路:
题目要求给出一组数字按键,然后不同数字按键有一组不同的字符,要求输出所有按键能够组成的不同字符组合。
这种要求不同字符组成不同的组合的题目首先应该想到的就是回溯算法,利用全排列算法作为基础模型,当然本题有点类似于N-皇后问题,不妨先写出N-皇后那种方式,就是2~9所有不同按键中的字符能够组成的不同字符情况。
代码如下:
class Solution {//创建长度为9的随机串
vector<char> sq;
vector<string> str= {"","","abc","def","ghi","jkl","mno","pqrs","tuv","wxyz"},ans;
void dfs(int x) {
if(x>9) {
string temp;
for(int i=0; i<sq.size(); i++)
temp+=sq[i];
ans.push_back(temp);
return;//递归边界
}
string temp;
for(int i=0; i<str[x].size(); i++) {
sq.push_back(str[x][i]);
dfs(x+1);
sq.pop_back();
}
}
public:
vector<string> letterCombinations(string digits) {
dfs(2);
return ans;
}
};
这时候便开始思考,本题要求的是,如果给出“234”,那么这三个按键组成的字符长度就应该是3,所以我们必须要用题目输入string的长度作为递归边界来解答。
AC代码:
class Solution {
vector<char> sq;
vector<string> str= {"","","abc","def","ghi","jkl","mno","pqrs","tuv","wxyz"},ans;
void dfs(int x,string &s,int size) {
if(x==size) {//当得到size个不同案件上的字符时候为递归边界
string temp;
for(int i=0; i<sq.size(); i++)
temp+=sq[i];
if(temp.size()>0)//避免空字符串存入
ans.push_back(temp);
return;
}
string temp;
int k=s[x]-'0';//针对数组中每个数字找到对应按键对应的字母
for(int i=0; i<str[k].size(); i++) {
sq.push_back(str[k][i]);
dfs(x+1,s,size);//x的作用是控制已经存入几个char字符
sq.pop_back();
}
}
public:
vector<string> letterCombinations(string digits) {
dfs(0,digits,digits.size());
return ans;
}
};
【推荐】还在用 ECharts 开发大屏?试试这款永久免费的开源 BI 工具!
【推荐】国内首个AI IDE,深度理解中文开发场景,立即下载体验Trae
【推荐】编程新体验,更懂你的AI,立即体验豆包MarsCode编程助手
【推荐】轻量又高性能的 SSH 工具 IShell:AI 加持,快人一步
· 探秘 MySQL 索引底层原理,解锁数据库优化的关键密码(下)
· 大模型 Token 究竟是啥:图解大模型Token
· 35岁程序员的中年求职记:四次碰壁后的深度反思
· 继承的思维:从思维模式到架构设计的深度解析
· 如何在 .NET 中 使用 ANTLR4
· 在线聊天系统中的多窗口数据同步技术解密
· 2025,回顾出走的 10 年
· 分享 3 款基于 .NET 开源且免费的远程桌面工具
· BotSharp 5.0 MCP:迈向更开放的AI Agent框架
· 【保姆级教程】windows 安装 docker 全流程