LeetCode 46. Permutations
Given an array nums of distinct integers, return all the possible permutations. You can return the answer in any order.
Example 1:
Input: nums = [1,2,3]
Output: [[1,2,3],[1,3,2],[2,1,3],[2,3,1],[3,1,2],[3,2,1]]
Example 2:
Input: nums = [0,1]
Output: [[0,1],[1,0]]
Example 3:
Input: nums = [1]
Output: [[1]]
Constraints:
1 <= nums.length <= 6
-10 <= nums[i] <= 10
All the integers of nums are unique.
思路:这是一题基于全排列的题目 经典模板题 是DFS搜索学习很好的一个训练题 上全排列DFS搜索过程的图
具体回溯的过程图:
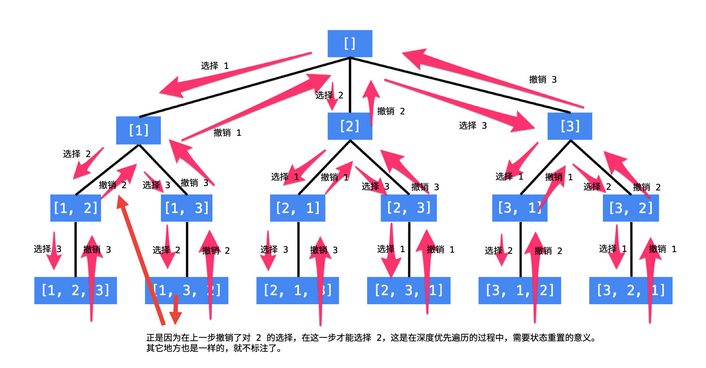
AC代码:
class Solution {
public:
vector<int> path;
bool vist[120]= {false};
void dfs(int idx,vector<int> &sq,vector<vector<int>> &ans) {
if(idx==sq.size()) {
ans.push_back(path);
return;
}
for(int i=0; i<sq.size(); i++) {
int val;
if(sq[i]<0) val=abs(sq[i])+100;//因为序列中有负数 所以如果为负数就+100转化为正数
else val=sq[i];
if(!vist[val]) {//当前值是否已经访问过
path.push_back(sq[i]);
vist[val]=true;
dfs(idx+1,sq,ans);
vist[val]=false;
path.pop_back();//用vector数组存序列的时候必须要递归后进行pop
}
}
}
vector<vector<int>> permute(vector<int>& nums) {
vector<vector<int>> ans;
dfs(0,nums,ans);
return ans;
}
};
运行效率
下面给出一般的C语言下全排列代码模板
//全排列
#include <iostream>
#include <vector>
using namespace std;
const int N=100;
int path[N],n;
bool vist[N]= {false};
void dfs(int idx) {//idx是索引
if(idx==n) {
for(int i=0; i<n; i++) {
printf("%d",path[i]);
if(i<n-1) printf(" ");
else printf("\n");
}
return;
}
for(int i=1; i<=n; i++) {
path[idx]=i;
if(!vist[i]) {
vist[i]=true;
dfs(idx+1);
vist[i]=false;
}
}
}
int main() {
cin>>n;
dfs(0);
return 0;
}