使用VS Code从零开始构建一个ASP .NET Core Web API 项目
首先需要安装.NET SDK 6.0 下载地址:Download .NET 6.0 (Linux, macOS, and Windows) (microsoft.com)
在VS Code终端运行指令创建项目
进入项目所在的文件夹
cd test
重启VS Code
code -r ../test
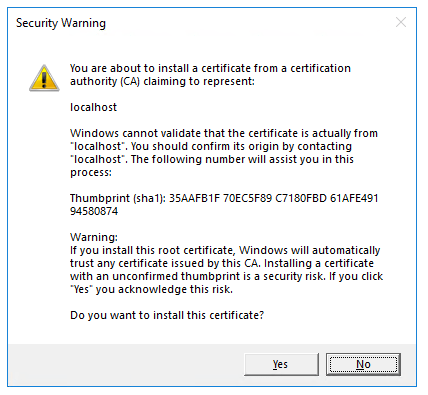
以上步骤结束后,下面就可以开始进行开发了。
新建Models文件夹
mkdir Models
在Models文件夹下添加实体类 UserModel.cs
using System.ComponentModel.DataAnnotations; using System.ComponentModel.DataAnnotations.Schema; namespace test.Models; [Table("User")] public class UserModel { [Key] public long ID { get; set; } [Required] [MaxLength(50)] [Column("UserName")] public string? UserName { get; set; } }
新建数据库上下文 TestDbContext.cs
using Microsoft.EntityFrameworkCore; using test.Models; namespace test; public class TestDbContext : DbContext { public TestDbContext(DbContextOptions<TestDbContext> options) : base(options) { } public DbSet<UserModel> UserModels { get; set; } = null!; }
在Program.cs中进行依赖注入
builder.Services.AddDbContext<TestDbContext>(opt => opt.UseSqlServer("Server=(localdb)\\mssqllocaldb;Database=Test;MultipleActiveResultSets=true;Trusted_Connection=True;"));
在终端执行以下指令,自动生成Controller
dotnet aspnet-codegenerator controller -name UserController -async -api -m UserModel -dc TestDbContext -outDir Controllers
可以看到,此时项目里的Controllers文件夹下自动生成了 UserController.cs 文件,里面自动生成了基本的增删改查方法。
执行以下指令,自动生成数据库
dotnet ef migrations add InitialCreate
dotnet ef database update
成功后,运行项目。
dotnet watch run
此时会自动弹出swagger页面
可以开始测试了。
以上就是全部内容。
参考微软官方教程:教程:使用 ASP.NET Core 创建 Web API | Microsoft Docs