快速入门
介绍
本指南向您展示如何创建使用Java 驱动程序 连接到MongoDB Atlas 集群的应用程序。如果您更喜欢使用不同的驱动程序或编程语言连接到 MongoDB,请参阅我们 的官方 MongoDB 驱动程序列表。
Java 驱动程序允许您从 Java 应用程序连接到 MongoDB 集群并与之通信。
MongoDB Atlas 是一种完全托管的云数据库服务,可将您的数据托管在 MongoDB 集群上。在本指南中,我们将向您展示如何开始使用您自己的免费(无需信用卡)集群。
请参考以下步骤将您的 Java 应用程序与 MongoDB Atlas 集群连接起来。
设置你的项目
安装 Java 开发工具包 (JDK)
确保您的系统安装了 JDK 8 或更高版本。有关如何检查 Java 版本和安装 JDK 的更多信息,请参阅 Oracle JDK 安装概述文档。
创建项目
本指南向您展示如何使用 Maven 或 Gradle 添加 MongoDB Java 驱动程序依赖项。我们建议您使用集成开发环境 (IDE),例如 Intellij IDEA 或 Eclipse IDE,以便更方便地配置 Maven 或 Gradle 来构建和运行您的项目。
如果您没有使用 IDE,请参阅 构建 Maven 或 创建新的 Gradle 构建 以获取有关如何设置项目的更多信息。
将 MongoDB 添加为依赖项
如果您使用的是Maven,请将以下内容添加到您的 pom.xml
依赖项列表中:
<dependencies> <dependency> <groupId>org.mongodb</groupId> <artifactId>mongodb-driver-sync</artifactId> <version>4.3.2</version> </dependency> </dependencies>
如果您使用的是Gradle,请将以下内容添加到您的 build.gradle
依赖项列表中:
dependencies { compile 'org.mongodb:mongodb-driver-sync:4.3.2' }
配置依赖项后,确保它们可用于您的项目,这可能需要运行依赖项管理器并在 IDE 中刷新项目。
创建 MongoDB 集群
在 Atlas 中设置免费层级集群
设置 Java 项目依赖项后,创建一个 MongoDB 集群,您可以在其中存储和管理数据。完成 Atlas 入门指南以设置新的 Atlas 帐户、创建和启动免费层 MongoDB 集群、加载数据集以及与数据交互。
完成 Atlas 指南中的步骤后,您应该在 Atlas 中部署了一个新的 MongoDB 集群、一个新的数据库用户,并将示例数据集加载到您的集群中。
连接到您的集群
在此步骤中,我们创建并运行一个应用程序,该应用程序使用 MongoDB Java 驱动程序连接到您的 MongoDB 集群并对示例数据运行查询。
我们通过一个名为connection string 的字符串向驱动程序传递有关如何连接到 MongoDB 集群的说明。此字符串包括有关集群的主机名或 IP 地址和端口、身份验证机制、适用的用户凭据以及其他连接选项的信息。
如果您要连接到不由 Atlas 托管的实例或集群,请参阅连接到 MongoDB 的其他方式以获取有关如何格式化连接字符串的说明。
要检索您在上一步中创建的集群和用户的连接字符串,请登录您的 Atlas 帐户并导航到Clusters部分,然后单击要连接到的 集群的Connect按钮,如下所示。
继续连接您的应用程序步骤并选择 Java 驱动程序。为版本选择“4.1 或更高版本”。单击复制图标将连接字符串复制到剪贴板,如下所示。
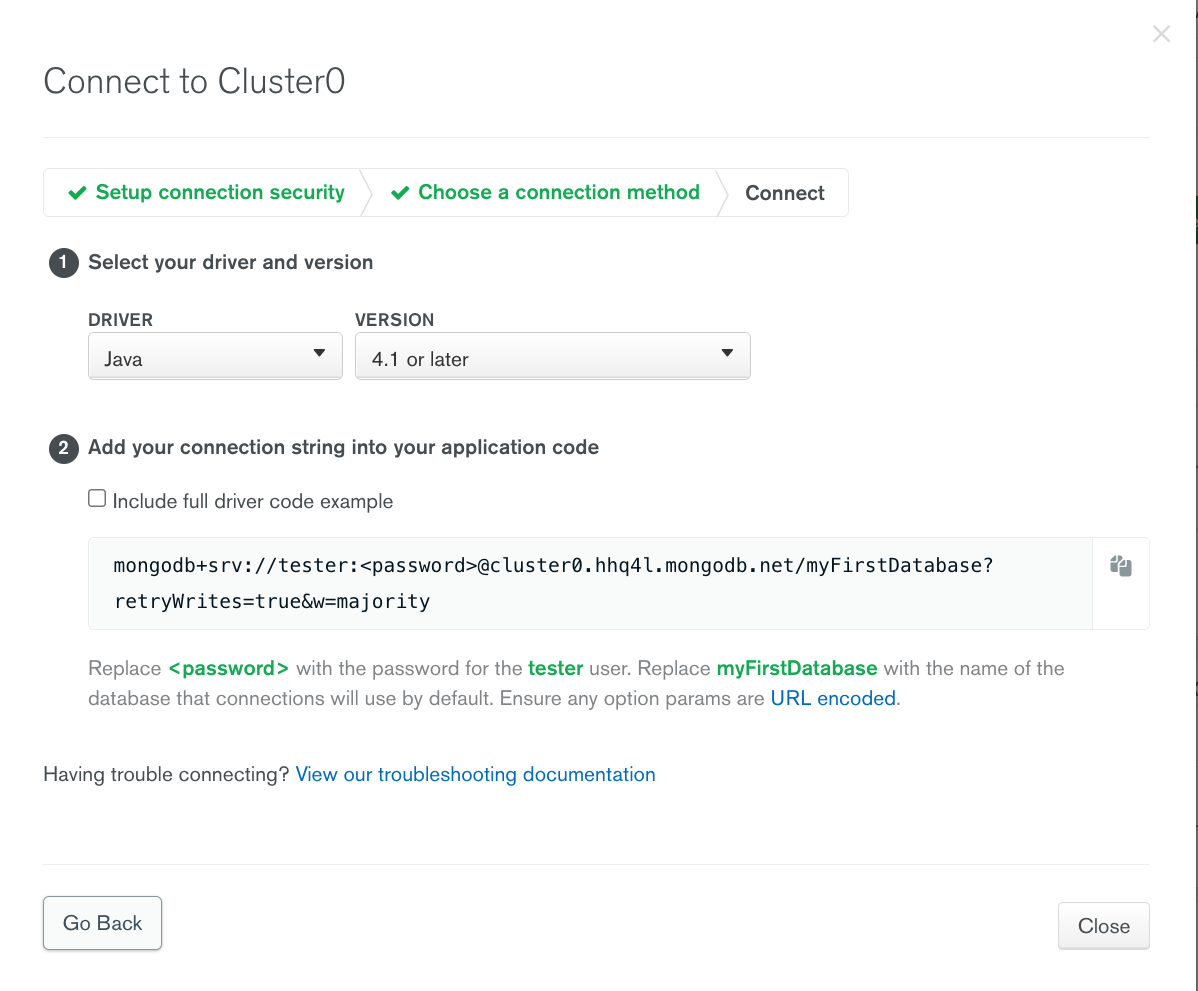
将您的 Atlas 连接字符串保存在一个安全的位置,您可以在下一步中访问该位置。
从您的应用程序查询您的 MongoDB 集群
接下来,创建一个文件以包含QuickStart.java
在项目的基本包目录中调用的应用程序。使用以下示例代码对 MongoDB Atlas 中的示例数据集运行查询,将uri
变量的值替换为您的 MongoDB Atlas 连接字符串。确保将连接字符串的“<password>”部分替换为您为具有atlasAdmin权限的用户创建的密码:
import static com.mongodb.client.model.Filters.eq; import org.bson.Document; import com.mongodb.client.MongoClient; import com.mongodb.client.MongoClients; import com.mongodb.client.MongoCollection; import com.mongodb.client.MongoDatabase;
public static void main( String[] args ) { // Replace the uri string with your MongoDB deployment's connection string String uri = "<connection string uri>"; try (MongoClient mongoClient = MongoClients.create(uri)) { MongoDatabase database = mongoClient.getDatabase("sample_mflix"); MongoCollection<Document> collection = database.getCollection("movies"); Document doc = collection.find(eq("title", "Back to the Future")).first(); System.out.println(doc.toJson()); } }
当你运行这个QuickStart
类时,它应该从样本数据集中输出电影的细节,它看起来像这样:
{ _id: ..., plot: 'A young man is accidentally sent 30 years into the past...', genres: [ 'Adventure', 'Comedy', 'Sci-Fi' ], ... title: 'Back to the Future', ... }
如果您没有收到输出或错误,请检查您的 Java 类中是否包含正确的连接字符串,以及您是否将示例数据集加载到 MongoDB Atlas 集群中。
如果您在运行应用程序时遇到类似于以下内容的连接到 MongoDB 实例或集群的错误,您可能需要将 JDK 更新到最新的补丁版本:
javax.net.ssl.SSLHandshakeException: extension (5) should not be presented in certificate_request
在特定版本的 JDK 中使用 TLS 1.3 协议时,此异常是一个已知问题,但已针对以下版本修复:
- JDK 11.0.7
- JDK 13.0.3
- JDK 14.0.2
要解决此错误,请将 JDK 更新为之前的补丁版本之一或更新的版本。
完成此步骤后,您应该拥有一个使用 Java 驱动程序连接到 MongoDB 集群、对示例数据运行查询并打印出结果的工作应用程序。
使用 POJO(可选)
在上一节中,您对示例集合运行了查询以检索类似地图的类中的数据Document
。在本节中,您可以学习使用您自己的普通旧 Java 对象 (POJO) 来存储和检索 MongoDB 中的数据。
Movie.java
在项目的基本包目录中创建一个文件,并为包含以下字段、setter 和 getter 的类添加以下代码:
public class Movie { String plot; List<String> genres; String title;
public String getPlot() { return plot; } public void setPlot(String plot) { this.plot = plot; } public List<String> getGenres() { return genres; } public void setGenres(List<String> genres) { this.genres = genres; } public String getTitle() { return title; } public void setTitle(String title) { this.title = title; } @Override public String toString() { return "Movie [\n plot=" + plot + ",\n genres=" + genres + ",\n title=" + title + "\n]"; } }
QuickStartPojoExample.java
在与Movie
项目中的文件相同的包目录中创建一个新文件。使用以下示例代码对 MongoDB Atlas 中的示例数据集运行查询,将uri
变量的值替换为您的 MongoDB Atlas 连接字符串。确保将连接字符串的“<password>”部分替换为您为具有atlasAdmin权限的用户创建的密码:
import static com.mongodb.MongoClientSettings.getDefaultCodecRegistry; import static com.mongodb.client.model.Filters.eq; import static org.bson.codecs.configuration.CodecRegistries.fromProviders; import static org.bson.codecs.configuration.CodecRegistries.fromRegistries; import org.bson.codecs.configuration.CodecProvider; import org.bson.codecs.configuration.CodecRegistry; import org.bson.codecs.pojo.PojoCodecProvider; import com.mongodb.client.MongoClient; import com.mongodb.client.MongoClients; import com.mongodb.client.MongoCollection; import com.mongodb.client.MongoDatabase;
public class QuickStartPojoExample { public static void main(String[] args) { CodecProvider pojoCodecProvider = PojoCodecProvider.builder().automatic(true).build(); CodecRegistry pojoCodecRegistry = fromRegistries(getDefaultCodecRegistry(), fromProviders(pojoCodecProvider)); // Replace the uri string with your MongoDB deployment's connection string String uri = "<connection string uri>"; try (MongoClient mongoClient = MongoClients.create(uri)) { MongoDatabase database = mongoClient.getDatabase("sample_mflix").withCodecRegistry(pojoCodecRegistry); MongoCollection<Movie> collection = database.getCollection("movies", Movie.class); Movie movie = collection.find(eq("title", "Back to the Future")).first(); System.out.println(movie); } } }
当你运行这个 QuickStartPojoExample
类时,它应该从样本数据集中输出电影的细节,它应该看起来像这样:
Movie [ plot=A young man is accidentally sent 30 years into the past..., genres=[Adventure, Comedy, Sci-Fi], title=Back to the Future ]
如果您没有收到输出或错误,请检查您的 Java 类中是否包含正确的连接字符串,以及您是否将示例数据集加载到 MongoDB Atlas 集群中。
有关使用 POJO 存储和检索数据的更多信息,请参阅以下链接:
后续步骤
在我们的基础 CRUD 指南中了解如何使用 Java 驱动程序读取和修改数据,或者在我们的使用示例中了解如何执行常见操作 。
官网地址:https://docs.mongodb.com/drivers/java/sync/current/quick-start/