[LeetCode] 1797. Design Authentication Manager
There is an authentication system that works with authentication tokens. For each session, the user will receive a new authentication token that will expire timeToLive
seconds after the currentTime
. If the token is renewed, the expiry time will be extended to expire timeToLive
seconds after the (potentially different) currentTime
.
Implement the AuthenticationManager
class:
AuthenticationManager(int timeToLive)
constructs theAuthenticationManager
and sets thetimeToLive
.generate(string tokenId, int currentTime)
generates a new token with the giventokenId
at the givencurrentTime
in seconds.renew(string tokenId, int currentTime)
renews the unexpired token with the giventokenId
at the givencurrentTime
in seconds. If there are no unexpired tokens with the giventokenId
, the request is ignored, and nothing happens.countUnexpiredTokens(int currentTime)
returns the number of unexpired tokens at the given currentTime.
Note that if a token expires at time t
, and another action happens on time t
(renew
or countUnexpiredTokens
), the expiration takes place before the other actions.
Example 1:
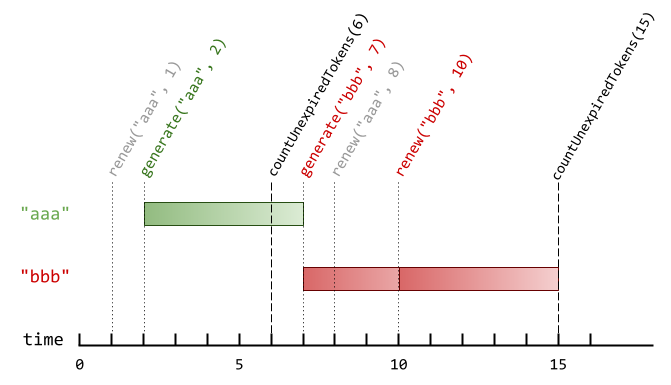
Input ["AuthenticationManager", "renew
", "generate", "countUnexpiredTokens
", "generate", "renew
", "renew
", "countUnexpiredTokens
"] [[5], ["aaa", 1], ["aaa", 2], [6], ["bbb", 7], ["aaa", 8], ["bbb", 10], [15]] Output [null, null, null, 1, null, null, null, 0] Explanation AuthenticationManager authenticationManager = new AuthenticationManager(5); // Constructs the AuthenticationManager withtimeToLive
= 5 seconds. authenticationManager.renew
("aaa", 1); // No token exists with tokenId "aaa" at time 1, so nothing happens. authenticationManager.generate("aaa", 2); // Generates a new token with tokenId "aaa" at time 2. authenticationManager.countUnexpiredTokens
(6); // The token with tokenId "aaa" is the only unexpired one at time 6, so return 1. authenticationManager.generate("bbb", 7); // Generates a new token with tokenId "bbb" at time 7. authenticationManager.renew
("aaa", 8); // The token with tokenId "aaa" expired at time 7, and 8 >= 7, so at time 8 therenew
request is ignored, and nothing happens. authenticationManager.renew
("bbb", 10); // The token with tokenId "bbb" is unexpired at time 10, so therenew
request is fulfilled and now the token will expire at time 15. authenticationManager.countUnexpiredTokens
(15); // The token with tokenId "bbb" expires at time 15, and the token with tokenId "aaa" expired at time 7, so currently no token is unexpired, so return 0.
Constraints:
1 <= timeToLive <= 108
1 <= currentTime <= 108
1 <= tokenId.length <= 5
tokenId
consists only of lowercase letters.- All calls to
generate
will contain unique values oftokenId
. - The values of
currentTime
across all the function calls will be strictly increasing. - At most
2000
calls will be made to all functions combined.
设计一个验证系统。
你需要设计一个包含验证码的验证系统。每一次验证中,用户会收到一个新的验证码,这个验证码在 currentTime 时刻之后 timeToLive 秒过期。如果验证码被更新了,那么它会在 currentTime (可能与之前的 currentTime 不同)时刻延长 timeToLive 秒。
请你实现 AuthenticationManager 类:
AuthenticationManager(int timeToLive) 构造 AuthenticationManager 并设置 timeToLive 参数。
generate(string tokenId, int currentTime) 给定 tokenId ,在当前时间 currentTime 生成一个新的验证码。
renew(string tokenId, int currentTime) 将给定 tokenId 且 未过期 的验证码在 currentTime 时刻更新。如果给定 tokenId 对应的验证码不存在或已过期,请你忽略该操作,不会有任何更新操作发生。
countUnexpiredTokens(int currentTime) 请返回在给定 currentTime 时刻,未过期 的验证码数目。
如果一个验证码在时刻 t 过期,且另一个操作恰好在时刻 t 发生(renew 或者 countUnexpiredTokens 操作),过期事件 优先于 其他操作。来源:力扣(LeetCode)
链接:https://leetcode.cn/problems/design-authentication-manager
著作权归领扣网络所有。商业转载请联系官方授权,非商业转载请注明出处。
思路是 hashmap。这道题不难,hashmap 就能解决,但是注意 renew 的规则,如果给定 tokenId 对应的验证码不存在或已过期,什么都不要做。注意 countUnexpiredTokens() 函数,我们统计有效 token 的时候,不能把无效的 token 删除,这样会报一个多线程的错误。所以只能通过判断当前 token 是否过期来决定他是否有效,没必要删除。
时间O(n)
空间O(n)
Java实现
1 class AuthenticationManager { 2 int timeToLive; 3 // <tokenId, startTime> 4 HashMap<String, Integer> map; 5 6 public AuthenticationManager(int timeToLive) { 7 this.timeToLive = timeToLive; 8 map = new HashMap<>(); 9 } 10 11 public void generate(String tokenId, int currentTime) { 12 map.put(tokenId, currentTime + timeToLive); 13 } 14 15 public void renew(String tokenId, int currentTime) { 16 // 存在的且还未过期的key才能被renew 17 if (map.containsKey(tokenId) && map.get(tokenId) > currentTime) { 18 map.put(tokenId, currentTime + timeToLive); 19 } 20 } 21 22 public int countUnexpiredTokens(int currentTime) { 23 int count = 0; 24 for (String id : map.keySet()) { 25 if (map.get(id) > currentTime) { 26 count++; 27 } 28 } 29 return count; 30 } 31 } 32 33 /** 34 * Your AuthenticationManager object will be instantiated and called as such: 35 * AuthenticationManager obj = new AuthenticationManager(timeToLive); 36 * obj.generate(tokenId,currentTime); 37 * obj.renew(tokenId,currentTime); 38 * int param_3 = obj.countUnexpiredTokens(currentTime); 39 */