[LeetCode] 2373. Largest Local Values in a Matrix
You are given an n x n
integer matrix grid
.
Generate an integer matrix maxLocal
of size (n - 2) x (n - 2)
such that:
maxLocal[i][j]
is equal to the largest value of the3 x 3
matrix ingrid
centered around rowi + 1
and columnj + 1
.
In other words, we want to find the largest value in every contiguous 3 x 3
matrix in grid
.
Return the generated matrix.
Example 1:
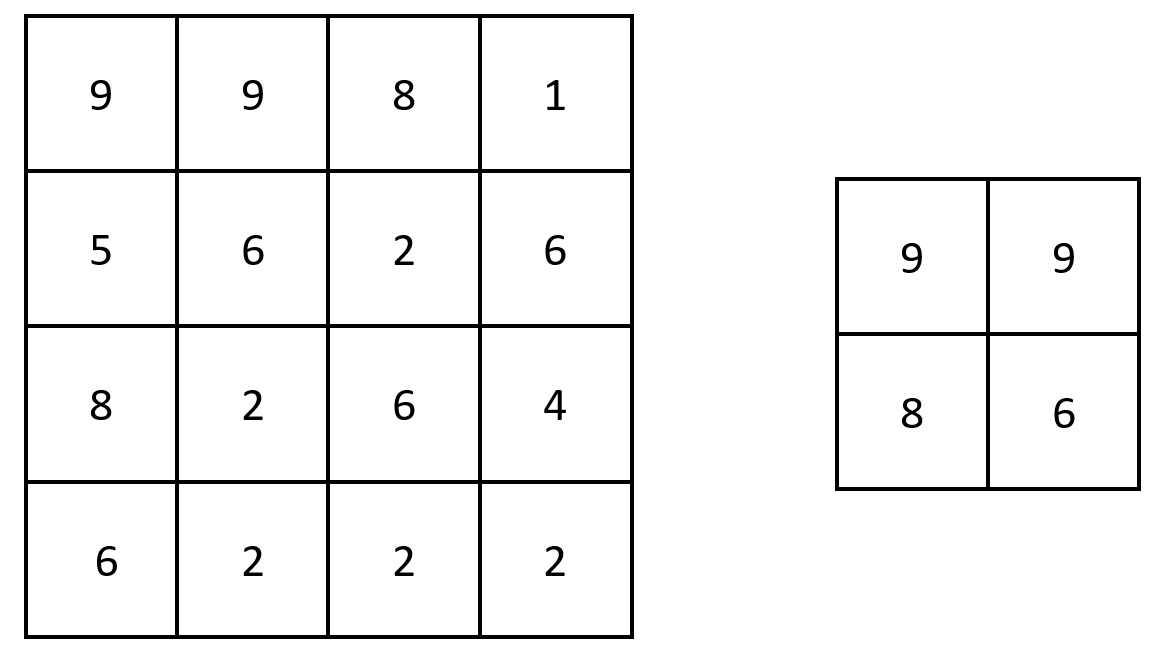
Input: grid = [[9,9,8,1],[5,6,2,6],[8,2,6,4],[6,2,2,2]] Output: [[9,9],[8,6]] Explanation: The diagram above shows the original matrix and the generated matrix. Notice that each value in the generated matrix corresponds to the largest value of a contiguous 3 x 3 matrix in grid.
Example 2:
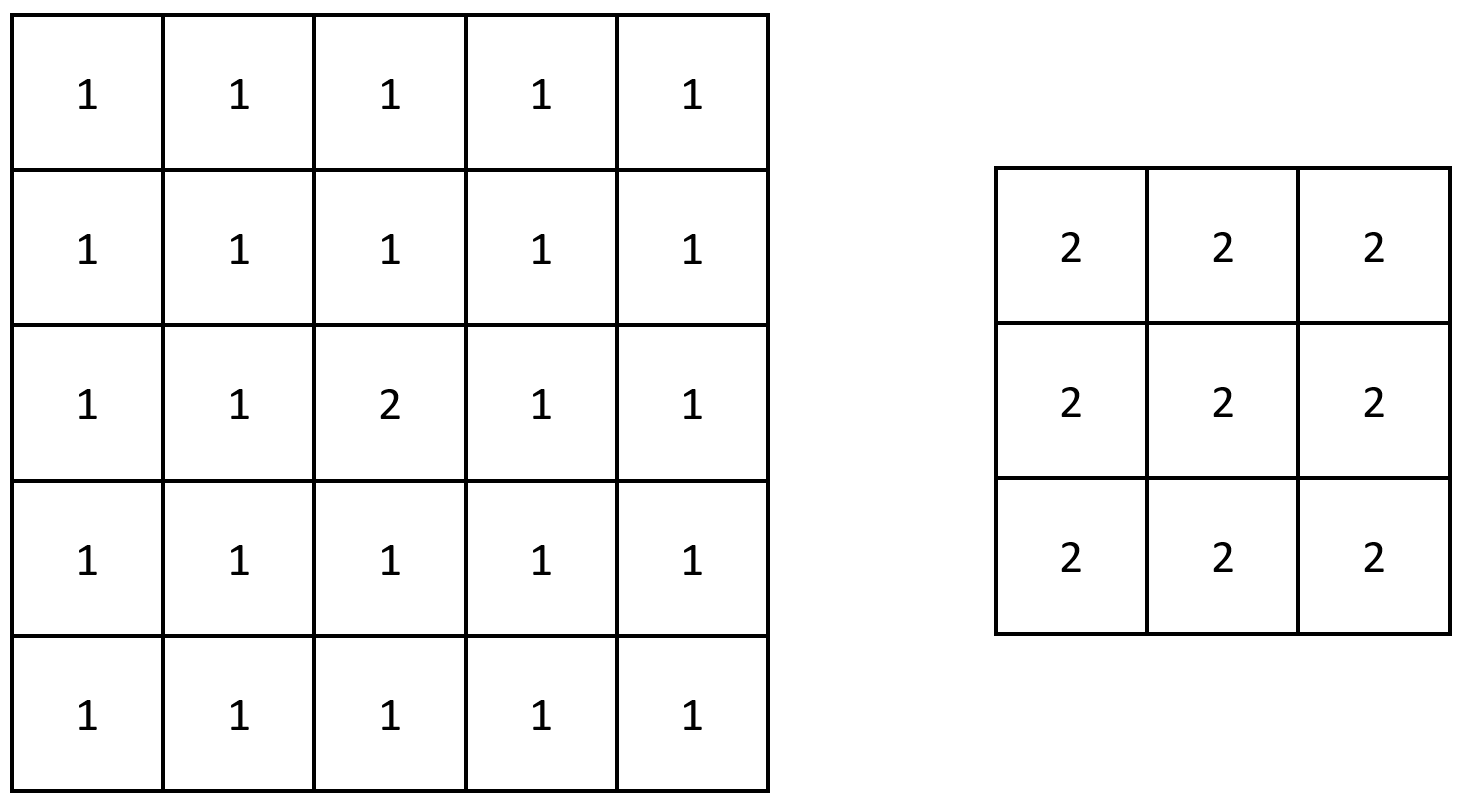
Input: grid = [[1,1,1,1,1],[1,1,1,1,1],[1,1,2,1,1],[1,1,1,1,1],[1,1,1,1,1]] Output: [[2,2,2],[2,2,2],[2,2,2]] Explanation: Notice that the 2 is contained within every contiguous 3 x 3 matrix in grid.
Constraints:
n == grid.length == grid[i].length
3 <= n <= 100
1 <= grid[i][j] <= 100
矩阵中的局部最大值。
给你一个大小为 n x n 的整数矩阵 grid 。
生成一个大小为 (n - 2) x (n - 2) 的整数矩阵 maxLocal ,并满足:
maxLocal[i][j] 等于 grid 中以 i + 1 行和 j + 1 列为中心的 3 x 3 矩阵中的 最大值 。
换句话说,我们希望找出 grid 中每个 3 x 3 矩阵中的最大值。返回生成的矩阵。
来源:力扣(LeetCode)
链接:https://leetcode.cn/problems/largest-local-values-in-a-matrix
著作权归领扣网络所有。商业转载请联系官方授权,非商业转载请注明出处。
这道题可以直接用暴力解扫描。注意返回的矩阵尺寸是原矩阵的长和宽各自减去2。
时间O(mn)
空间O(mn)
Java实现
1 class Solution { 2 public int[][] largestLocal(int[][] grid) { 3 int m = grid.length; 4 int n = grid[0].length; 5 int[][] res = new int[m - 2][n - 2]; 6 // 遍历左上角的元素 7 for (int i = 0; i < m - 2; i++) { 8 for (int j = 0; j < n - 2; j++) { 9 int max = 0; 10 for (int k = i; k < i + 3; k++) { 11 for (int l = j; l < j + 3; l++) { 12 max = Math.max(max, grid[k][l]); 13 } 14 } 15 res[i][j] = max; 16 } 17 } 18 return res; 19 } 20 }