[LeetCode] 2280. Minimum Lines to Represent a Line Chart
You are given a 2D integer array stockPrices
where stockPrices[i] = [dayi, pricei]
indicates the price of the stock on day dayi
is pricei
. A line chart is created from the array by plotting the points on an XY plane with the X-axis representing the day and the Y-axis representing the price and connecting adjacent points. One such example is shown below:
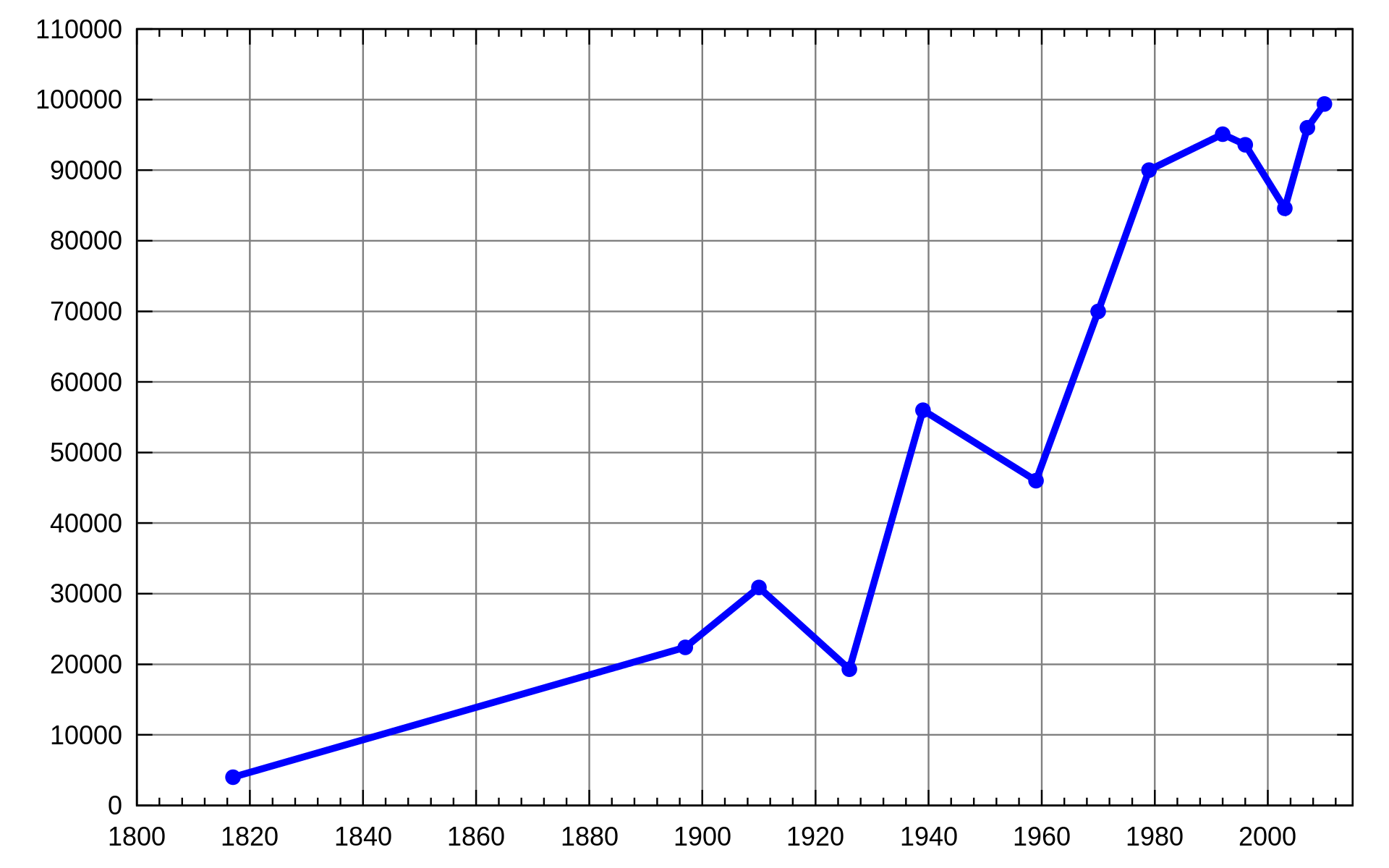
Return the minimum number of lines needed to represent the line chart.
Example 1:
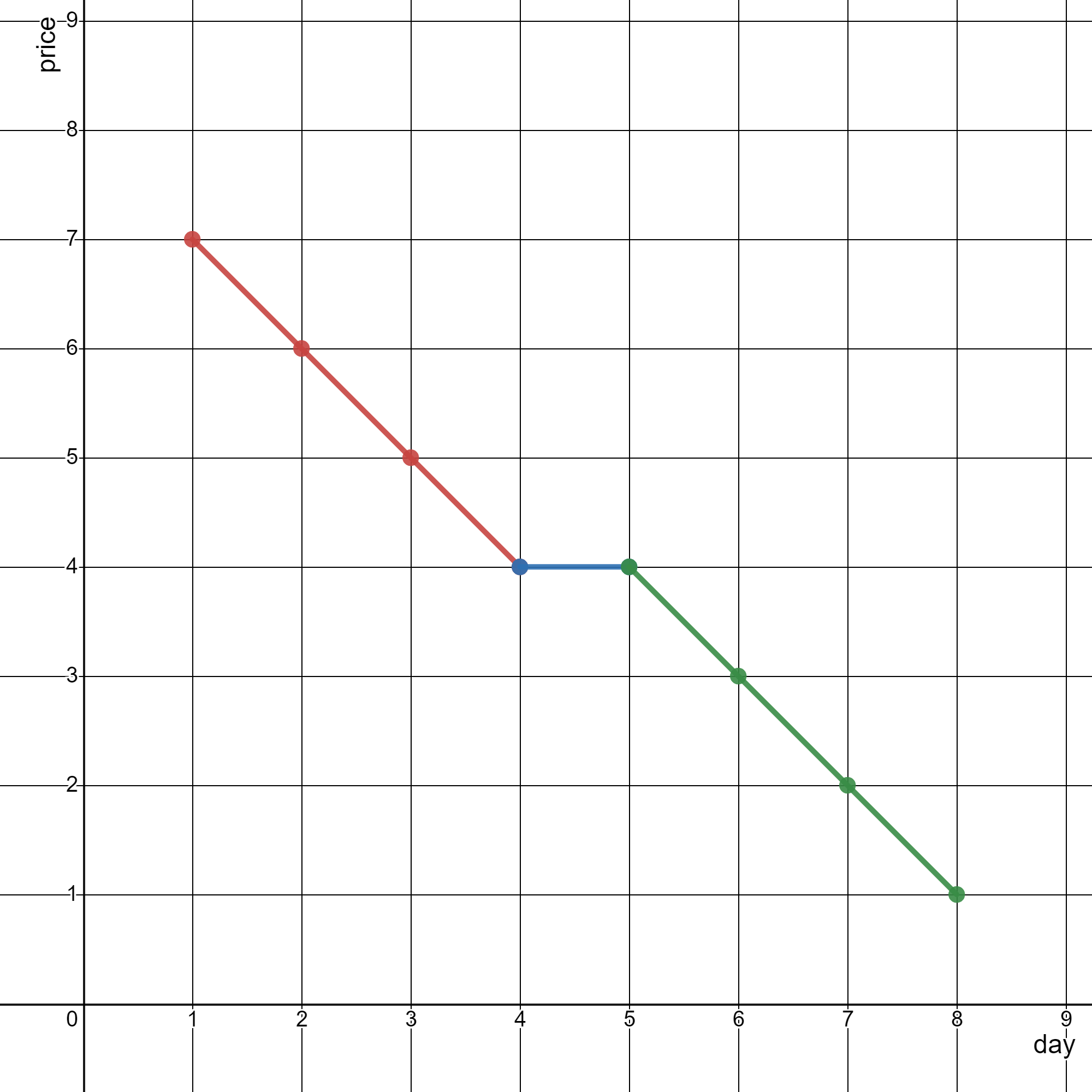
Input: stockPrices = [[1,7],[2,6],[3,5],[4,4],[5,4],[6,3],[7,2],[8,1]] Output: 3 Explanation: The diagram above represents the input, with the X-axis representing the day and Y-axis representing the price. The following 3 lines can be drawn to represent the line chart: - Line 1 (in red) from (1,7) to (4,4) passing through (1,7), (2,6), (3,5), and (4,4). - Line 2 (in blue) from (4,4) to (5,4). - Line 3 (in green) from (5,4) to (8,1) passing through (5,4), (6,3), (7,2), and (8,1). It can be shown that it is not possible to represent the line chart using less than 3 lines.
Example 2:
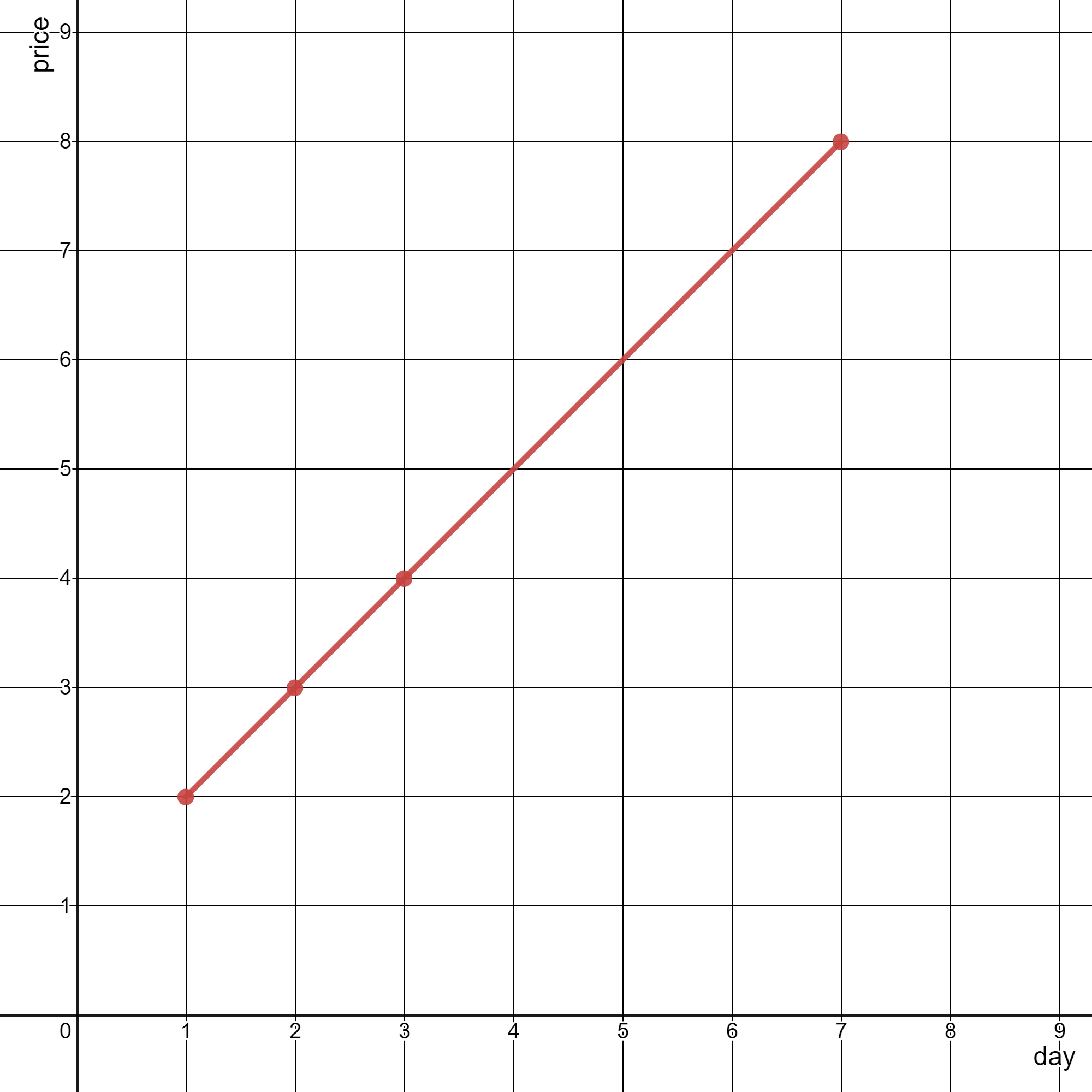
Input: stockPrices = [[3,4],[1,2],[7,8],[2,3]] Output: 1 Explanation: As shown in the diagram above, the line chart can be represented with a single line.
Constraints:
1 <= stockPrices.length <= 105
stockPrices[i].length == 2
1 <= dayi, pricei <= 109
- All
dayi
are distinct.
表示一个折线图的最少线段数。
给你一个二维整数数组 stockPrices ,其中 stockPrices[i] = [dayi, pricei] 表示股票在 dayi 的价格为 pricei 。折线图 是一个二维平面上的若干个点组成的图,横坐标表示日期,纵坐标表示价格,折线图由相邻的点连接而成。请你返回要表示一个折线图所需要的 最少线段数 。
来源:力扣(LeetCode)
链接:https://leetcode.cn/problems/minimum-lines-to-represent-a-line-chart
著作权归领扣网络所有。商业转载请联系官方授权,非商业转载请注明出处。
这是一道数学题,跟斜率 slope 相关。题目给的是股票的价值,用一些点表示,这些点形成一个折线图,题目问折线图至少需要几段。
我的思路是,从第一个点开始,判断每两个相邻的点组成的线段的斜率,比如看第一天和第二天的这段折线的斜率是否跟第二天和第三天的这段折线的斜率一样。如果一样,说明这两条折线其实是一条,如果不一样,就需要多画一条折线了。注意这道题的 input 数组是没有排序的,所以我们需要先排序再做比较。比较的方式还是注意斜率分母不能为 0 的问题。
时间O(nlogn)
空间O(n)
Java实现
1 class Solution { 2 public int minimumLines(int[][] stockPrices) { 3 int len = stockPrices.length; 4 // corner case 5 if (len == 1) { 6 return 0; 7 } 8 9 // normal case 10 Arrays.sort(stockPrices, (a, b) -> (a[0] - b[0])); 11 // for (int i = 0; i < len; i++) { 12 // System.out.println(stockPrices[i][0] + ", " + stockPrices[i][1]); 13 // } 14 15 int y1 = stockPrices[1][1] - stockPrices[0][1]; 16 int x1 = stockPrices[1][0] - stockPrices[0][0]; 17 int res = 1; 18 for (int i = 2; i < len; i++) { 19 int y2 = stockPrices[i][1] - stockPrices[i - 1][1]; 20 int x2 = stockPrices[i][0] - stockPrices[i - 1][0]; 21 if (x1 * y2 != x2 * y1) { 22 res++; 23 y1 = y2; 24 x1 = x2; 25 } 26 } 27 return res; 28 } 29 }
相关题目
1232. Check If It Is a Straight Line