import java.io.*;
import java.util.*;
public class Main {
public static int n = 100001;
public static int m = 100001;
public static ArrayList<ArrayList<Integer>> graph = new ArrayList<>();
public static PriorityQueue<Integer> heap = new PriorityQueue<>();
public static int[] indegree = new int[n];
public static int[] ans = new int[n];
public static int cnt = 0;
public static void build() {
graph.clear();
heap.clear();
cnt = 0;
for (int i = 0; i < n; i++) {
graph.add(new ArrayList<>());
}
}
public static void main(String[] args) throws IOException {
BufferedReader br = new BufferedReader(new InputStreamReader(System.in));
StreamTokenizer in = new StreamTokenizer(br);
PrintWriter out = new PrintWriter(new OutputStreamWriter(System.out));
while (in.nextToken() != StreamTokenizer.TT_EOF) {
n = (int) in.nval + 1;
in.nextToken();
m = (int) in.nval;
build();
for (int i = 0, from, to; i < m; i++) {
in.nextToken();
from = (int) in.nval;
in.nextToken();
to = (int) in.nval;
addEdge(from, to);
indegree[to]++;
}
topoSort();
for (int i = 0; i < n; i++) {
out.print(ans[i] + " ");
}
out.println(ans[n - 1]);
}
out.flush();
out.close();
br.close();
}
private static void topoSort() {
for (int i = 1; i < n; i++) {
if (indegree[i] == 0) {
heap.add(i);
}
}
while (!heap.isEmpty()) {
int cur = heap.poll();
ans[cnt++] = cur;
for (int i : graph.get(cur)) {
indegree[i]--;
if (indegree[i] == 0) {
heap.add(i);
}
}
}
}
private static void addEdge(int from, int to) {
graph.get(from).add(to);
}
}
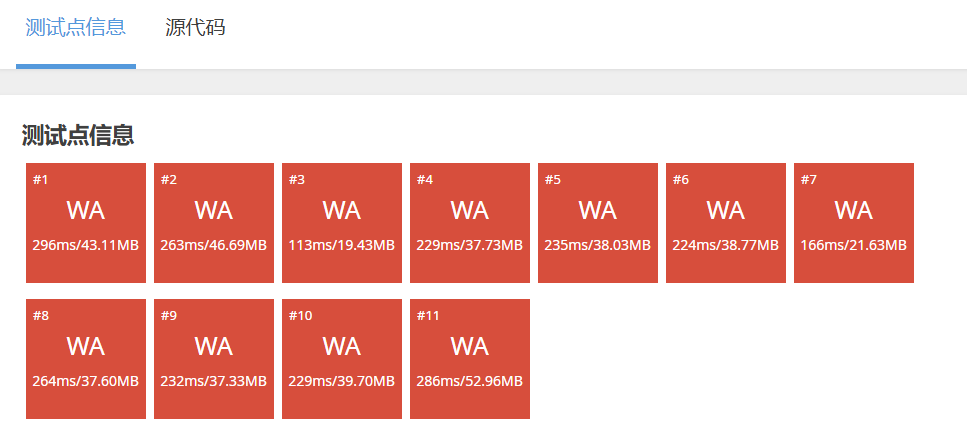